In this article, we will see the methods of the string class in Java. But before directly moving towards string methods, let us first see a little bit about strings in Java, like what they are, how to initialize them, and why strings are immutable in Java.
In Java, the string is basically an object that represents a sequence of char values. An array of characters works the same as a Java string.
Implementing a string as an object provides some of the string handling methods, so we can do some operations on strings like comparing two strings, searching in a string, concatenating two strings,sub-string method, and so on.
What is the immutability of string in Java?
When you are creating a string in Java, you are creating an object of the String class that cannot be changed. Formally, when an object of string type is created, you can’t modify the characters of that string.
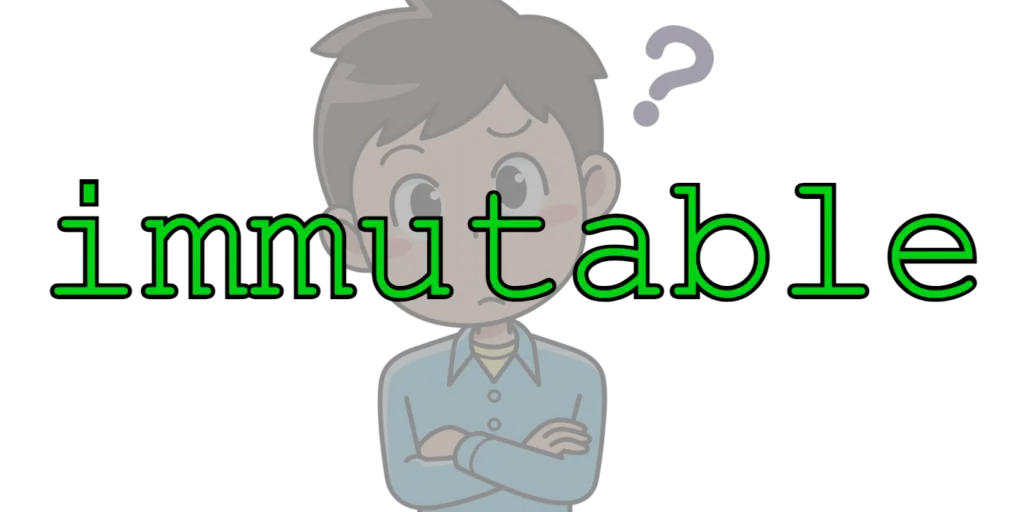
At first glance, it can look unbelievable. But Java String objects have this property for some security reasons. You can, however, perform methods on strings to modify them; the difference is that each time you will obtain an altered version of an existing string, Which recreates at each modification.
How to initialize a String in Java
We will see the initialization of a string in two ways. in which one is putting a string input in the string() function directly and the other way is by passing a character array as an argument.
Using the Constructor which takes the string as input
package newPackage; public class BeeTechnical{ public static void main(String[] args) { String str = new String("This is an input as string"); System.out.println(str); } }
Using the Constructor which takes the char array as input
package newPackage; public class BeeTechnical{ public static void main(String[] args) { char[] array = {'B','e','e','T','e','c','h','n','i','c','a','l'}; String str = new String(array); System.out.println(str); } }
As we have seen in the beginning string has multiple methods to perform the different operations. Let’s explore each method with some example code.
Length() Method in String
The length of a string is the number of characters it contains in itself. To obtain the value of length, we call the length( ) method on the String object by using the dot operator. The general representation of the length method is obj.length(), where “obj” is the object of the string class.
package newPackage; public class BeeTechnical{ public static void main(String[] args) { char[] array = {'B','e','e','T','e','c','h','n','i','c','a','l'}; String str = new String(array); System.out.println("Number of characters are : "+str.length()); } }
Output:
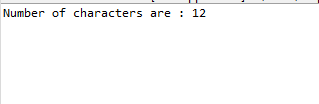
String Concatenation using the + Operator
We can concatenate the strings by using the “+” operator. We can concatenate a string with two types. One is to concatenate a string with another string-type object, and the other is to concatenate a string with other type objects(int).
But the output produced in both cases will be the same. Because the int value in age is automatically converted into its object of the String class.
After that, the string is then concatenated as before. The compiler will automatically convert an operand to its string equivalent whenever the other operand of the + is an instance of a String. The general representation of the concatenating is: Str1 + Str2 and “Bee” + “Technical”, where “Str1” and “Str2” are objects of string class and concatenate through the dot operator, and “Bee” and “Technical” are direct string concatenates through the plus operator.
We can see the code concatenation of String with both types of data types.
package newPackage; public class BeeTechnical{ public static void main(String[] args) { String str_1 = "Bee"; String str_2 = "Technical"; System.out.println("Concatenate of str_1 and str_2 is : "+(str_1+str_2)); } }
Output:
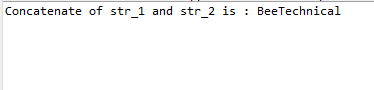
ChatAt() Method to Find the Specific Character
To obtain the value of a single character from a string, you can directly use the charAt() method by passing a 0-based index value as an argument inside this method. It has this general form: charAt(int index)
package newPackage; public class BeeTechnical{ public static void main(String[] args) { String str = "BeeTechnical"; System.out.println("Character at 4th index : "+str.charAt(4)); } }
Output :
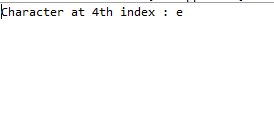
GetChars() Method to Find the list of Characters
If We need to obtain more than one character, Then we need to use the getChars( ) method. The general form of the method is as follows: void getChars(int Start, int End, char arr[ ], int arrStart).
Here, Start specifies the index of the starting of the required sub-string, and End specifies an index that is one
past the end of the required sub-string. the obtained sub-string contains the characters from Start through End
1. The array that will receive the characters is specified by a target in the method argument and the index within the target at which index the sub-string will be copied is passed in arrStart.
package newPackage; import java.util.*; public class BeeTechnical{ public static void main(String[] args) { String str = "BeeTechnical"; int start_index=0; int end_index=5; char[] arr = new char[end_index-start_index]; str.getChars(start_index, end_index, arr, 0); System.out.println("Characters from 0 to 4 are : "+Arrays.toString(arr)); } }
Output :
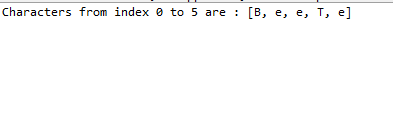
Convert String to Array using, ToCharArray() method
The simplest way to obtain the character array from the String object is to use the toCharArray () method via the dot operator on the String class object.
It returns an array of characters for the entire string. It has this general form as follows: obj.toCharArray( ), where “obj” is the object of the String class.
The result is the same and can also be obtained by using the getChars() method.
package newPackage; import java.util.*; public class BeeTechnical{ public static void main(String[] args) { String str = "BeeTechnical"; char[] arr = str.toCharArray(); System.out.println(Arrays.toString(arr)); } }
Output :
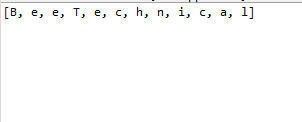
Compare Two Strings Using Equals()
To compare two strings for their values of characters, we use equals( ). It has this general form:
boolean equals(String str).
package newPackage; import java.util.*; public class BeeTechnical{ public static void main(String[] args) { String str1="abc"; String str2="abc"; String str3="dec"; System.out.println("str1 is equal to str2 : "+str1.equals(str2)); System.out.println("str2 is equal to str3 : "+str2.equals(str3)); } }
Output :
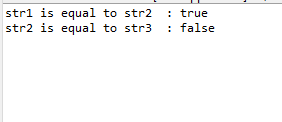
StartWith() & EndWith() Methods
The startsWith( ) method returns the result as a boolean type after checking whether a given string begins with a specified string. On the other hand, endsWith( ) determines whether the string ends with a specified string or not.
They have the following general forms: boolean startsWith(String str) and boolean endsWith(String str), where str is the string type object.
package newPackage; import java.util.*; public class BeeTechnical{ public static void main(String[] args) { String str="BeeTechnical"; System.out.println("str1 starts with Bee : "+str.startsWith("Bee")); System.out.println("str1 ends with Technical : "+str.endsWith("Technical")); } }
Output :
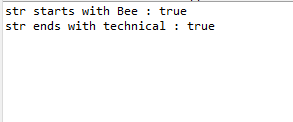
IndexOf() & LastIndexOf() Methods
To search for the first occurrence of a character in a string-type object, We use int indexOf(int ch) and for the last occurrence of a character, We use int lastIndexOf(int ch).
package newPackage; import java.util.*; public class BeeTechnical{ public static void main(String[] args) { String str="BeeTechnical"; System.out.println("First index of e is : "+str.indexOf("e")); System.out.println("Last index of e is : "+str.lastIndexOf("e")); } }
Output :
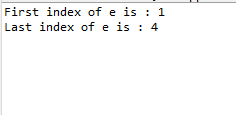
SubString() Method to Extract the Substring
We can obtain the sub-string using substring( ) method of the string class.
It has two forms.
The first one is String substring(int start_Index), Where start_Index specifies the index from which the sub-string will start. This form returns a copy of the sub-string that begins from the integer type argument passed in place of start_Index and runs to the end of the string at which this method is invoked.
The second form of substring( ) allows us to obtain the sub-string from both the start and end index of the sub-string as String substring(int start_Index, int end_Index), Where start_Index specifies the starting index of required sub-string and end_Index specifies the ending point of sub-string.
package newPackage; import java.util.*; public class BeeTechnical{ public static void main(String[] args) { String str="BeeTechnical"; System.out.println("Sub-string from index 1 to last of the string : "+str.substring(1)); System.out.println("Sub-string from index 1 to 5th index of the string : "+str.substring(1,5)); } }
Output :
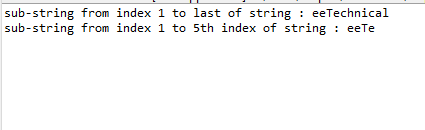
ToUpperCase() & ToLowerCase() Methods
When the method toLowerCase() is invoked on the string using the dot operator, it converts all the characters in a string from uppercase to lowercase, and the toUpperCase( ) method converts all the characters in a string from lowercase to uppercase.
package newPackage; import java.util.*; public class BeeTechnical{ public static void main(String[] args) { String str="BeeTechnical"; System.out.println("LowerCase is : "+str.toLowerCase()); System.out.println("UpperCAse is : "+str.toUpperCase()); } }
Output :
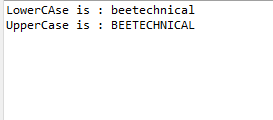
Contains() Method
If we want to check if a string contains a sub-string or not then we use contain() method. Which returns its result as a boolean type answer. Its general form is: boolean contains(String str)
package newPackage; import java.util.*; public class BeeTechnical{ public static void main(String[] args) { String str="BeeTechnical"; System.out.println("str contains T : "+str.contains("T")); System.out.println("str contains Z : "+str.contains("Z")); } }
Output :
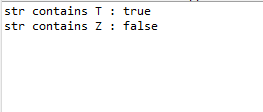
Conclusion
We tried to understand the concepts of String in Java and the list of methods available in the string class to perform various operations.
Also, We tried understanding each method in detail using the example code and output of it
Comments are closed.