Azure Functions are at the forefront of serverless computing, offering a powerful solution for developers to build and deploy code efficiently in response to various events. In this comprehensive guide, we present the top 30 Azure Functions interview questions and answers to help you master the essential concepts, features, and best practices. Whether you’re preparing for an interview or looking to enhance your understanding of Azure Functions, this resource will be your key to success.
What is Azure Functions?
Azure Functions is a serverless compute service that enables developers to build, deploy, and manage small pieces of code without worrying about server infrastructure. These functions can be triggered by various events, such as HTTP requests, timer-based schedules, or data changes.
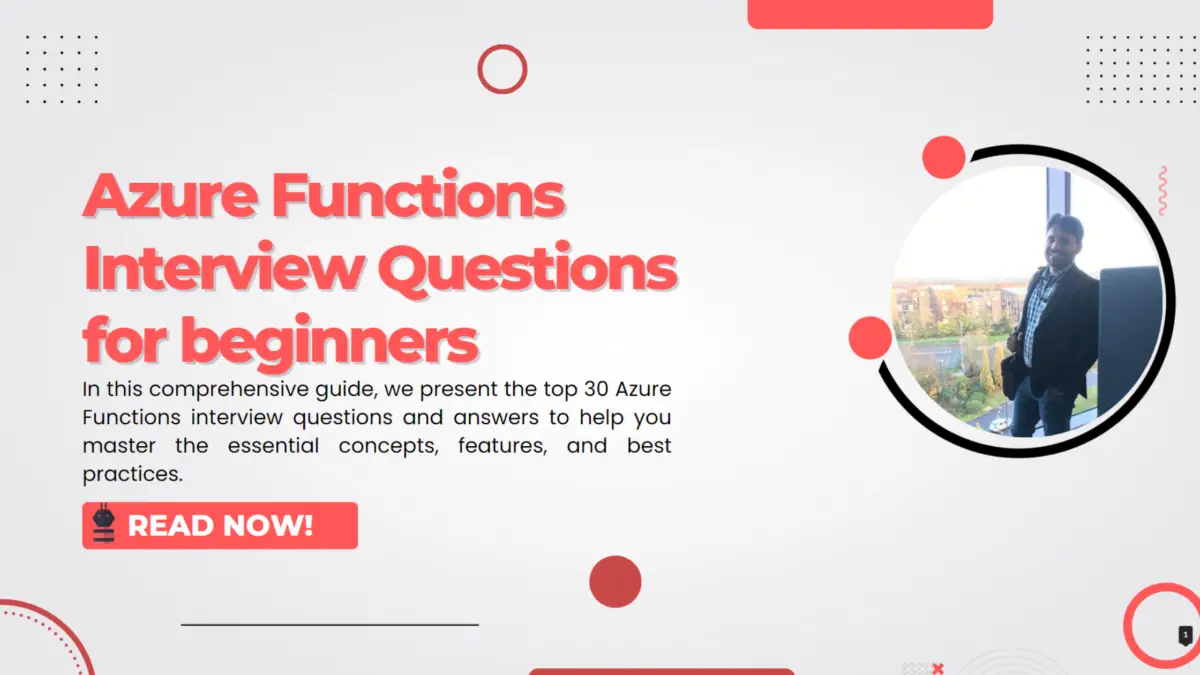
What are the supported languages for writing Azure Functions?
C#,Javascript,Python, Java, and PowerShell are also supported with their respective syntax.
How can you deploy an Azure Function?
You can deploy an Azure Function using various methods, such as:
- Azure Portal: You can create and deploy functions directly through the Azure Portal by creating a Function App and uploading your code.
- Visual Studio: You can use Visual Studio to build and publish Azure Functions.
- Azure DevOps: Set up a CI/CD pipeline in Azure DevOps to automate the deployment process.
What is an HTTP Trigger and a Timer Trigger in Azure Functions?
An HTTP Trigger can be used to create an API endpoint that executes your function when an HTTP request is made. For example, it can respond to an HTTP GET request by returning data to the client.
[FunctionName("HttpTriggerExample")] public static async Task<IActionResult> Run( [HttpTrigger(AuthorizationLevel.Function, "get", Route = null)] HttpRequest req, ILogger log) { log.LogInformation("C# HTTP trigger function processed a request."); // Your code here return new OkObjectResult("Hello, Azure Functions!"); }
What is a Function App in Azure?
A Function App is a logical container for Azure Functions. It provides a unified management and deployment platform for your functions. You can create and manage Function Apps through the Azure Portal, and within a Function App, you can host multiple Azure Functions.
What are the key benefits of Azure Functions?
Key benefits of Azure Functions include:
- Scalability: Azure Functions automatically scale based on the number of incoming requests or events, ensuring optimal performance
- Cost-Efficiency: You pay only for the resources consumed during function execution, which makes it cost-effective for variable workloads.
- Simplified Management: Azure Functions abstract away server management, allowing developers to focus on code and application logic.
What is the Azure Functions Consumption Plan?
The Consumption Plan is the default hosting plan for Azure Functions. It automatically scales based on the number of incoming requests. For example, if you have an HTTP-triggered function in a Consumption Plan, it automatically scales to handle increased web traffic without any manual intervention.
How does Azure Functions handle scaling automatically?
Azure Functions scale automatically based on the number of incoming requests or events. For example, if you have a function handling incoming messages from a queue, as the queue load increases, Azure Functions will provision additional instances to process the messages in parallel, ensuring timely processing.
Explain Azure Durable Functions.
Azure Durable Functions allow you to create complex, stateful workflows by orchestrating multiple Azure Functions. For instance, you can create a durable workflow that involves multiple functions to process an order, update inventory, and notify customers when their order is complete.
What is a binding in Azure Functions?
A binding in Azure Functions is a way to connect input and output data to your functions without writing boilerplate code. For example, if you want to read from an Azure Queue Storage, you can use an input binding like this:
[FunctionName("QueueTriggerExample")] public static void Run( [QueueTrigger("myqueue-items", Connection = "AzureWebJobsStorage")] string myQueueItem, ILogger log) { log.LogInformation($"C# Queue trigger function processed: {myQueueItem}"); // Your code here }
What is the purpose of the Azure Functions runtime?
The Azure Functions runtime manages the execution of Azure Functions. It takes care of function scaling, load balancing, and resource allocation. This means developers don’t have to worry about infrastructure management, and they can focus on writing code.
Explain the concept of “Function Invocation” in Azure Functions.
Function Invocation refers to the process of executing a specific Azure Function in response to a trigger or an event. For example, when an HTTP request is made to an HTTP-triggered function, it invokes the function code.
What is the difference between an Azure Function and a WebJob?
Azure Functions are event-driven, whereas WebJobs are background processing tasks. For example, if you have a WebJob, it can continuously process items in a queue, whereas Azure Functions are triggered to process items as events occur.
Can you run long-duration processes in Azure Functions?
Azure Functions are optimized for short-duration tasks. For long-running processes, consider using Azure Durable Functions or other Azure services like Azure Logic Apps or Azure Kubernetes Service (AKS).
How can you secure Azure Function endpoints?
Azure Functions can be secured using authentication and authorization mechanisms. For example, you can enable authentication for an HTTP-triggered function and require valid API keys or tokens for access. You can set up authentication in the Azure Portal like this:
What is an “Output Binding” in Azure Functions?
An Output Binding allows you to send data from an Azure Function to external services or storage. For example, if you want to store the result of a function in Azure Table Storage, you can use an output binding like this:
[FunctionName("StoreInTableStorage")] [return: Table("MyTable")] public static MyData StoreInTableStorage( [HttpTrigger(AuthorizationLevel.Function, "post")] MyData data) { return data; }
Distinguish between Azure Function and WebJob.
Azure Functions are event-driven, whereas WebJobs are background processing tasks that can be triggered in various ways.
Can you run long-duration processes in Azure Functions?
Azure Functions are better for short tasks; long processes are suitable for other services like Azure Durable Functions.
How do you secure Azure Function endpoints?
Authentication and authorization mechanisms, including API keys or Azure AD, can secure endpoints.
What is an Output Binding in Azure Functions?
Output Bindings send data from a function to external services or storage.
How can you handle errors and exceptions in Azure Functions?
Use try-catch blocks and built-in exception handling to manage errors.
What is an Event Grid Trigger in Azure Functions?
Event Grid Trigger allows Azure Functions to respond to events from Azure Event Grid.
What is Azure Functions Premium Plan, and when is it beneficial?
Premium Plan offers advanced features and is suitable for enterprise-level workloads with VNET integration and unlimited execution duration.
How can you access environment variables in Azure Functions?
Access environment variables using System.Environment.GetEnvironmentVariable.
Explain the difference between a stateless and stateful Azure Function.
Stateless functions do not maintain state between executions, while stateful functions can retain state
What is “Triggers and Bindings” in Azure Functions and how do they relate?
Triggers initiate function execution, while bindings connect input and output data to functions
Can you run Docker containers in Azure Functions?
Yes, with Azure Functions Premium Plan and custom Docker images.
How do you set up authentication for an HTTP-triggered Azure Function?
Enable authentication in the Azure Portal and configure authentication providers.
How can you schedule the execution of an Azure Function at a specific time?
Use a Timer Trigger with a CRON expression to schedule function execution.
What is the “Azure Functions Core Tools” and its use?
Azure Functions Core Tools is a command-line interface for developing, testing, and debugging Azure Functions locally.
What is the purpose of “Function Keys” in Azure Functions?
Function Keys are used for securing and controlling access to HTTP-triggered functions.
Explain the concept of “Managed Identities” in Azure Functions.
Managed Identities enable secure access to Azure resources without explicit credentials.
Conclusion
Azure Functions is a versatile serverless compute service that empowers developers to build and deploy code in response to various events without the hassle of managing infrastructure. With support for multiple languages, automatic scaling, and a wide range of triggers and bindings, Azure Functions simplifies application development and offers efficient solutions for a variety of use cases. Understanding its core concepts and features is essential for effectively harnessing the power of serverless computing in the Azure ecosystem.