C# is a versatile and powerful programming language that offers developers various ways to work with collections of data. Two commonly used approaches for handling immutable collections are ReadOnlyList
and ImmutableList
. While they might sound similar, they have distinct characteristics, and choosing between them depends on your specific use case. In this article we will try to understand Readonly List vs. Immutable List utilizing illustrative examples, exploring their usage scenarios, and evaluating their respective performance characteristics.
Overview of ReadOnlyList and ImmutableList
C# ReadOnlyList
is a read-only wrapper for an existing list, preventing modifications while ImmutableList
is truly immutable, creating new instances upon modification. ReadOnlyList
offers lower performance overhead but depends on the underlying list’s thread safety, while ImmutableList
is inherently thread-safe due to immutability. Choose ReadOnlyList
for read-only access to existing lists and ImmutableList
for data integrity across operations.
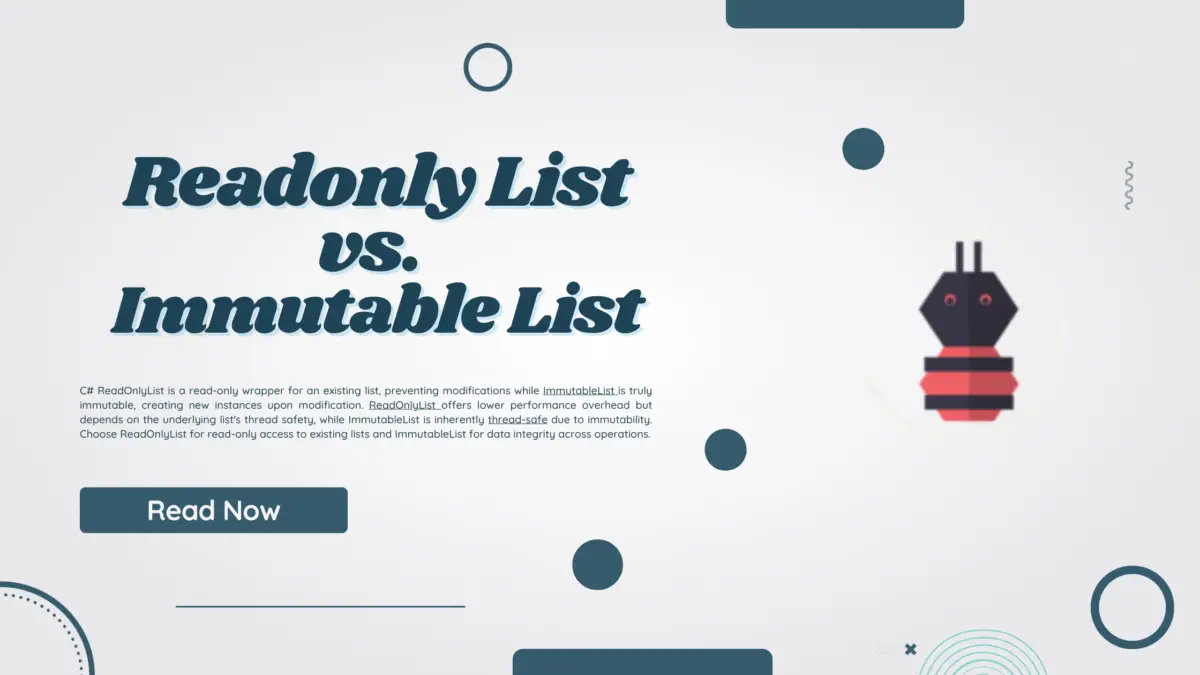
Comparison Table
To better understand the differences between ReadOnlyList
and ImmutableList
, let’s compare them in the following table:
Aspect | ReadOnlyList | ImmutableList |
---|---|---|
Mutability | Read-only wrapper around an existing list. | Truly immutable; any modification creates a new instance. |
Performance | Minimal performance overhead since it operates on an existing list. | Creates new instances when modified, potentially impacting performance in certain scenarios. |
Memory Usage | Low memory footprint as it doesn’t create new copies of the list. | Generates new instances, potentially consuming more memory. |
Thread Safety | Not inherently thread-safe; thread safety depends on the underlying list. | Thread-safe by design, thanks to immutability. |
Usage Scenarios | Suitable for scenarios where you want to prevent modifications to a list. | Ideal for scenarios where you need to ensure data integrity across multiple operations. |
Creation | Created using the AsReadOnly() method or constructor. | Created using builder methods or extension methods. |
Examples | List<int> myList = new List<int> { 1, 2, 3 }; <br>ReadOnlyList<int> readOnlyList = myList.AsReadOnly(); | ImmutableList<int> immutableList = ImmutableList.Create(1, 2, 3); |
Now, let’s dive deeper into each of these aspects to understand the implications and use cases.
Mutability
ReadOnlyList
A ReadOnlyList
is essentially a read-only view of an existing list. It allows you to prevent modifications to the original list while still providing access to its elements. Any attempt to modify a ReadOnlyList
will result in an exception. This is suitable when you want to expose data as read-only to external code.
ImmutableList
An ImmutableList
, by its very nature, is immutable. Once you create an instance, you cannot modify it. Instead, any modification operation produces a new ImmutableList
with the desired changes. This immutability is crucial when you need to ensure that your data remains unchanged throughout your program’s execution.
Performance
ReadOnlyList
ReadOnlyList
generally has minimal performance overhead because it operates directly on the underlying list. Since it’s essentially a wrapper, read operations are fast. However, if the underlying list is modified frequently, there may be a slight overhead due to validation checks to ensure read-only behavior.
ImmutableList
ImmutableList
creates new instances when modified. While this immutability guarantees data integrity, it can introduce performance overhead in scenarios with frequent updates. Each modification operation creates a new instance, potentially leading to increased memory usage and slower performance compared to mutable collections.
Memory Usage
ReadOnlyList
ReadOnlyList
has a low memory footprint because it doesn’t create new copies of the underlying list. It shares memory with the original list and only adds a thin layer of read-only behavior.
ImmutableList
ImmutableList
generates new instances when modified. This can lead to increased memory usage, especially in situations with frequent modifications. However, the memory overhead is usually manageable unless you’re working with extremely large collections.
Thread Safety
ReadOnlyList
ReadOnlyList
is not inherently thread-safe. Its thread safety depends on the thread safety of the underlying list. If the original list is thread-safe, the ReadOnlyList
will be as well. If the underlying list is not thread-safe, concurrent access to the ReadOnlyList
can result in race conditions.
ImmutableList
ImmutableList
is inherently thread-safe due to its immutability. Since you can’t modify an existing instance, there’s no risk of race conditions when multiple threads access it concurrently.
Usage Scenarios
ReadOnlyList
- Use
ReadOnlyList
when you want to expose a read-only view of an existing list. - It’s suitable for scenarios where you need to prevent modifications to a collection while allowing others to read its contents.
ImmutableList
- Choose
ImmutableList
when you need to ensure data integrity across multiple operations. - Ideal for scenarios where you want to avoid unexpected changes to data, such as in functional programming or multi-threaded environments.
Creation
ReadOnlyList
You can create a ReadOnlyList
by using the AsReadOnly()
method or the constructor that accepts an existing list as a parameter. Here’s an example using AsReadOnly()
:
List<int> myList = new List<int> { 1, 2, 3 }; ReadOnlyList<int> readOnlyList = myList.AsReadOnly();
ImmutableList
Creating an ImmutableList
involves using builder methods or extension methods. Here’s an example:
ImmutableList<int> immutableList = ImmutableList.Create(1, 2, 3);
Examples
Let’s walk through some practical examples to illustrate the usage of ReadOnlyList
and ImmutableList
.
Example 1: ReadOnlyList
List<int> myList = new List<int> { 1, 2, 3 }; ReadOnlyList<int> readOnlyList = myList.AsReadOnly(); // Read operations are allowed. int firstItem = readOnlyList[0]; // Attempting to modify the read-only list will result in an exception. // readOnlyList.Add(4); // This line would throw an exception.
Example 2: ImmutableList
csharpCopy code
ImmutableList<int> immutableList = ImmutableList.Create(1, 2, 3); // Any modification creates a new instance. ImmutableList<int> updatedList = immutableList.Add(4); // The original list remains unchanged. bool isOriginalListSame = ReferenceEquals(immutableList, updatedList); // false // Read operations on the original list are still allowed. int firstItem = immutableList[0];
In Example 1, the ReadOnlyList
prevents modifications to the underlying list, whereas Example 2 demonstrates how ImmutableList
ensures immutability by creating new instances upon modification.
Conclusion
In summary, ReadOnlyList
and ImmutableList
are both valuable tools in C# for working with immutable collections, but they serve different purposes. ReadOnlyList
is ideal when you need a read-only view of an existing list, while ImmutableList
ensures data integrity by creating new