Callbacks in C# are a way to pass a method as an argument to another method. The method that receives the callback can then call the passed method at some point during its execution.
To give you an example, let’s say we have a method that performs a long-running operation, such as downloading a large file from the internet. We want to be notified when the download is complete, so we can take some action, such as displaying a message to the user.
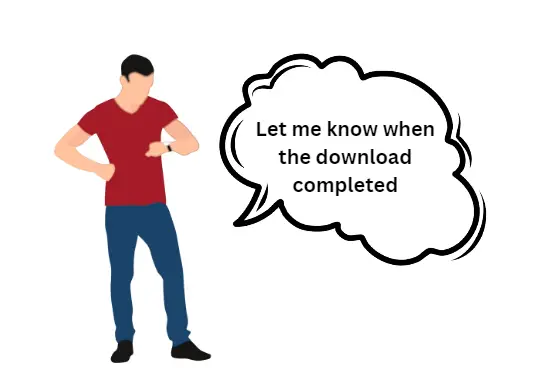
We can use a callback to accomplish this. We define a method that we want to be called when the download is complete, and we pass it as an argument to the download method. When the download is complete, the download method calls the callback method, and we can take the appropriate action.
Understand With Example
In this example, we define a DownloadFile
method that takes a URL and a Action
delegate (which represents a method with no arguments and no return value) as arguments. We start the download, and when it’s complete, we call the onComplete
callback.
public void DownloadFile(string url, Action onComplete) { // start the download... // when the download is complete, call the onComplete callback onComplete(); } public void HandleDownloadComplete() { Console.WriteLine("Download complete!"); } public void StartDownload() { DownloadFile("http://example.com/file.txt", HandleDownloadComplete); }
We also define a HandleDownloadComplete
the method that simply writes a message to the console.
Finally, we define a StartDownload
method that calls DownloadFile
with the appropriate arguments, including the HandleDownloadComplete
a method as the callback.
When we call, the download starts, and when it’s complete, the HandleDownloadComplete
method is called, which writes “Download complete!” to the console.
I hope that helps explain callbacks in C# in a way that’s easy to understand!
Difference between delegate and callback in C#?
I know it is a little bit confusing, However, delegates and callbacks are similar concepts, they serve slightly different purposes in C#.
A delegate is a type that represents a reference to a method with a specific signature. Delegates can be used to pass methods as arguments to other methods, to define events and event handlers, and to implement callback methods.
On the other hand, a callback is a method that is passed as an argument to another method and is intended to be called by that method at a later time. The callback method can be used to perform some action or to provide some data back to the calling method.
So, while a callback is a specific use case of a delegate, delegates have a wider range of uses beyond just callbacks.
In terms of implementation, delegates are defined using the delegate
keyword, while callbacks are just regular methods that are passed as arguments to other methods.
// Define a delegate that takes two integers and returns a boolean public delegate bool ComparisonDelegate(int x, int y); // Define a method that takes a comparison delegate as an argument and uses it to compare two integers public void Sort(int[] array, ComparisonDelegate comparisonDelegate) { // implementation omitted for brevity } // Define a callback method that prints the result of the comparison to the console public void ComparisonCallback(int x, int y, bool result) { Console.WriteLine($"The comparison of {x} and {y} is {result}"); } // Usage: pass the ComparisonCallback method as the callback to the Sort method int[] numbers = { 5, 2, 8, 1 }; Sort(numbers, ComparisonCallback);
In this example, we define a ComparisonDelegate
delegate that takes two integers and returns a boolean. We also define a Sort
method that takes an array of integers and a ComparisonDelegate
delegate as arguments. The Sort
method uses the delegate to compare the integers in the array.
We then define a ComparisonCallback
method that takes two integers and a boolean as arguments and prints the result of the comparison to the console. Finally, we pass the ComparisonCallback
method as the callback to the Sort
method.
So, in summary, while delegates and callbacks are similar concepts, delegates have a wider range of uses beyond just callbacks, and delegates are defined using the delegate
keyword, while callbacks are just regular methods that are passed as arguments to other methods.
When to use callbacks instead of events in c#?
Callbacks are typically used when you want to pass a method as an argument to another method and have that method call the passed method when a certain condition is met.
Callbacks are often used in scenarios where you need more fine-grained control over the invocation of the callback method, such as when you need to control the order in which callbacks are executed, or when you need to pass additional data to the callback method.
On the other hand, events are typically used when you want to allow multiple subscribers to be notified when an event occurs. Events are often used in scenarios where you have a large number of potential subscribers, or when you need to decouple the event source from the event handlers.
So, in general, you should use callbacks when you want to control the invocation of the callback method and events when you want to allow multiple subscribers to be notified when an event occurs.
Conclusion
In conclusion, callbacks and events are both powerful tools for implementing asynchronous programming in C#. The choice between using callbacks or events depends on the specific requirements of your application.
If you need fine-grained control over the invocation of the callback method, or if you need to pass additional data to the callback method, then callbacks are a good choice. If you need to allow multiple subscribers to be notified when an event occurs, then events are the way to go.
Ultimately, the key is to choose the approach that best meets the needs of your application while maintaining clean and maintainable code.
Can delegates be used as callbacks?
Yes, delegates can be used as callbacks in C#. In fact, using delegates as callbacks is a common pattern in C# programming.
What is the difference between delegate and interface in C#?
Delegates and interfaces are both mechanisms for defining contracts in C#, but they serve different purposes.
A delegate is a type that represents a reference to a method with a specific signature. Delegates are typically used to pass methods as arguments to other methods or to define event handlers. Delegates allow you to treat methods as first-class objects, which can be passed around and assigned to variables.
On the other hand, an interface is a blueprint for a class that defines a set of properties, methods, and events. Interfaces allow you to define a contract that other classes can implement. When a class implements an interface, it must provide implementations for all of the methods and properties defined in the interface.
Comments are closed.