A Hashtable in C# is a collection that stores key-value pairs in a way that allows fast retrieval of values based on their associated keys. It provides an efficient and flexible way to organize and access data, making it a valuable tool for many programming tasks.
In this article, we’ll explore the key features of C# Hashtable, including its differences from the Dictionary class. We’ll also provide examples of how to create and use Hashtable in your C# code, helping you to unlock the full potential of this useful data structure.
Characteristics of C# Hashtable
Here are some features of the Hashtable data structure in C#:
- Fast and efficient access to values based on their associated keys: Hashtables uses a hashing algorithm to store and retrieve key-value pairs, which provides constant-time access to values based on their keys, making them a fast and efficient data structure.
- Keys are unique: Each key in a Hashtable must be unique, which ensures that values are associated with the correct key and avoids key collisions.
- Dynamic size: Hashtables can dynamically grow or shrink in size as needed, based on the number of key-value pairs they contain, making them flexible and adaptable to changing data needs.
- Supports different data types: Hashtables in C# can store key-value pairs of different data types, including strings, integers, and objects.
- Enumeration: Hashtables can be enumerated using the foreach statement, which allows for easy iteration over all key-value pairs in the Hashtable.
- Thread-safe: Hashtables in C# are thread-safe, meaning they can be accessed by multiple threads simultaneously without causing race conditions or other thread-related issues.
Create, Update, Remove, Access, and Enumerate Hashtable in C#?
To create a Hashtable in C#, you first need to import the System.Collections namespace, which contains the Hashtable class. Then, you can create an instance of the Hashtable class using the “new” keyword
using System; using System.Collections; class Program { static void Main(string[] args) { // Create a new Hashtable Hashtable myHashtable = new Hashtable(); // Add some key-value pairs to the Hashtable myHashtable.Add("apple", "red"); myHashtable.Add("banana", "yellow"); myHashtable.Add("orange", "orange"); // Access a value using its key string colorOfApple = (string)myHashtable["apple"]; Console.WriteLine("The color of an apple is " + colorOfApple); // Update a value using its key myHashtable["banana"] = "green"; string colorOfBanana = (string)myHashtable["banana"]; Console.WriteLine("The color of a banana is " + colorOfBanana); // Remove a key-value pair myHashtable.Remove("orange"); // Enumerate over the Hashtable and print each key-value pair foreach (DictionaryEntry entry in myHashtable) { Console.WriteLine(entry.Key + ": " + entry.Value); } } }
In this example, we create a Hashtable and add three key-value pairs to it, where the keys are “apple”, “banana”, and “orange”, and the values are “red”, “yellow”, and “orange”, respectively. We then access the value associated with the key “apple”, update the value associated with the key “banana”, remove the key-value pair associated with the key “orange”, and enumerate over the Hashtable to print each key-value pair.
The output of this program will be:
The color of an apple is red The color of a banana is green apple: red banana: green
Difference between dictionary and hashtable in c#
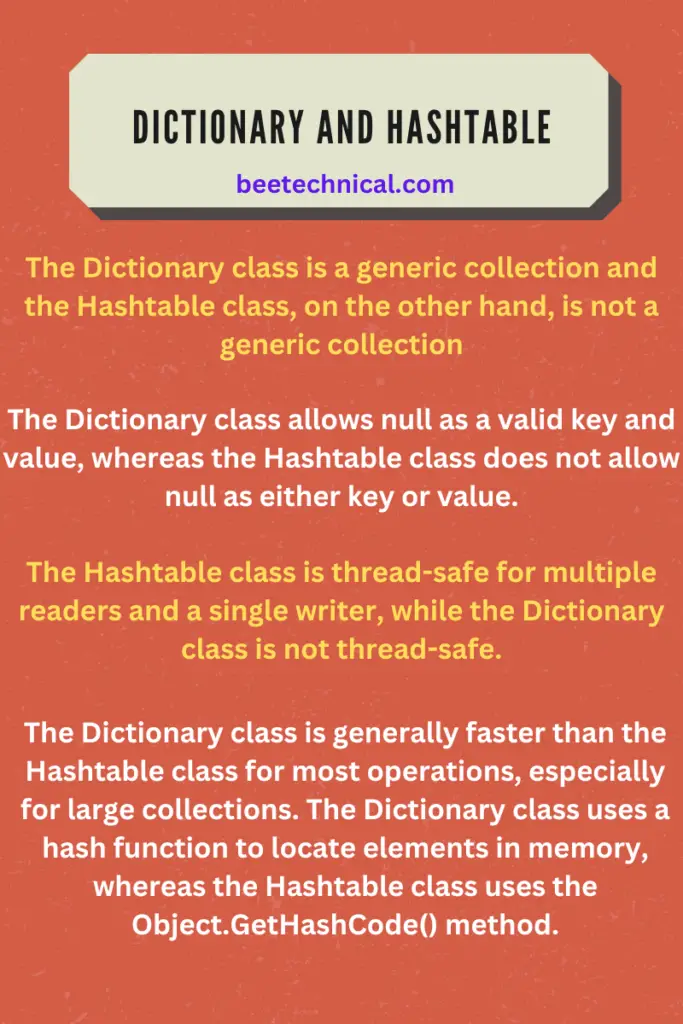
In C#, both the Dictionary and Hashtable classes provide key-value mapping functionality, but there are some differences between them:
- Generics vs. non-generics: The Dictionary class is a generic collection, which means that it can be strongly typed to hold a specific type of key-value pair. The Hashtable class, on the other hand, is not a generic collection, which means that it stores objects as keys and values, and requires explicit casting to retrieve values.
- Null keys and values: The Dictionary class allows null as a valid key and value, whereas the Hashtable class does not allow null as either key or value.
- Thread safety: The Hashtable class is thread-safe for multiple readers and a single writer, while the Dictionary class is not thread-safe.
- Performance: The Dictionary class is generally faster than the Hashtable class for most operations, especially for large collections. The Dictionary class uses a hash function to locate elements in memory, whereas the Hashtable class uses the Object.GetHashCode() method.
- Enumeration order: The Dictionary class maintains the order in which items were added to the collection, whereas the Hashtable class does not guarantee the order of the elements.
Can Hashtable store duplicate values in C#?
Yes, Hashtable in C# can store duplicate values. In a Hashtable, the keys must be unique, but the values can be duplicates.
For example, in the following code, we add three key-value pairs to a Hashtable, where two of the values are the same:
Hashtable ht = new Hashtable(); ht.Add("key1", "value1"); ht.Add("key2", "value2"); ht.Add("key3", "value1");
In this case, “value1” appears twice in the Hashtable, associated with two different keys (“key1” and “key3”).
However, it’s important to note that the keys in a Hashtable must be unique. If you try to add a key-value pair with a key that already exists in the Hashtable, it will replace the existing value with the new value.
Conclusion
In conclusion, both the Hashtable and Dictionary classes in C# provide key-value mapping functionality, but they differ in their implementation, performance, and usage.
If you need a strongly typed, generic collection that allows null keys and values, and the order of elements is important, you should use the Dictionary class. On the other hand, if you need a thread-safe collection that doesn’t allow null keys or values, and the order of elements is not important, you should use the Hashtable class.
Overall, it’s important to consider your specific requirements when choosing between these two classes.
Comments are closed.