C# MemoryStream is a built-in class in the .NET Framework that allows developers to work with data streams in memory. It provides a way to read and write bytes to an in-memory stream, rather than to a file or network socket.
Using MemoryStream in C#, developers can perform a variety of operations such as buffering data, compressing data, or converting data between different formats. MemoryStream also provides features such as seek and position, which allow developers to read or write data at any point in the stream.
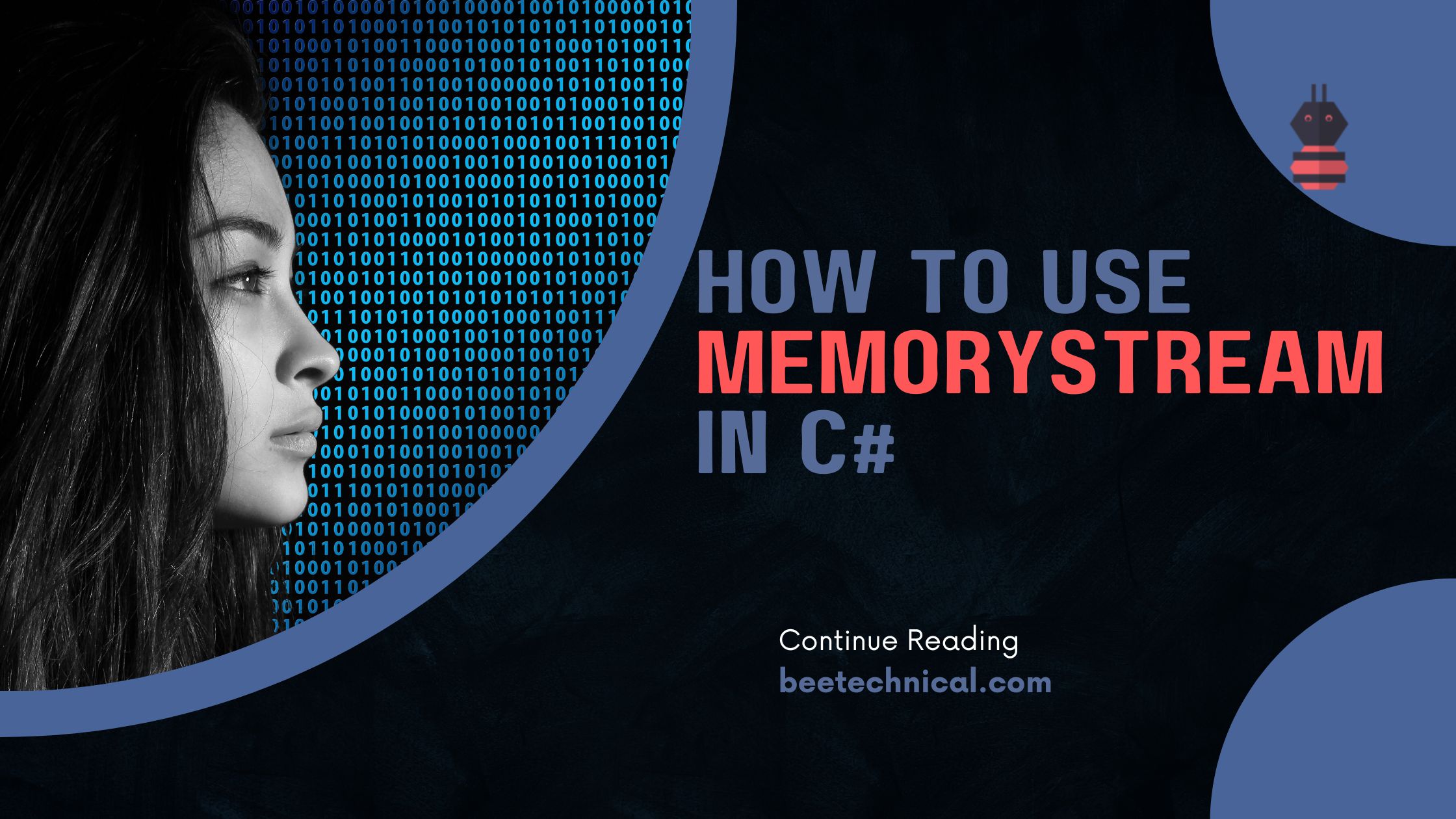
One of the key benefits of using MemoryStream is that it allows for faster data access than traditional file-based operations, which can result in improved performance for applications that handle large amounts of data. Additionally, since data is stored in memory, it is not subject to the same security risks as data stored on disk.
Use cases of MemoryStream in C#
The MemoryStream
class in C# provides an in-memory stream that can be used as a target or source for reading and writing operations. Here are some common use cases for MemoryStream
:
- In-Memory Data Manipulation:
MemoryStream
can be used to perform operations on data stored in memory without the need for physical files. It allows you to read, write, and manipulate data using familiar stream-based operations. - Serialization and Deserialization:
MemoryStream
is often used in the serialization and deserialization of objects. You can write serialized object data into aMemoryStream
, and later deserialize the data from the stream. This is useful when you need to convert objects into a byte stream representation or vice versa. - Testing and Mocking:
MemoryStream
can be employed in unit testing scenarios where you want to simulate reading from or writing to a stream. It allows you to create a stream with predefined data and use it in place of an actual file or network stream during testing. - Memory-Based File Operations:
MemoryStream
can serve as a temporary storage location when performing file-related operations in memory. For example, you can read the content of a file intoMemoryStream
for manipulation or perform operations on the stream before writing it back to a file. - Interoperability with Other APIs:
MemoryStream
can be useful when working with APIs that expect stream-based input or output. It allows you to easily convert data between different representations, such as strings, byte arrays, or streams, to interface with various components or libraries. - Caching and Buffering:
MemoryStream
can be used as an in-memory cache or buffer to store frequently accessed or computed data. By utilizing aMemoryStream
for caching, you can avoid unnecessary disk I/O operations and improve overall performance.
Remember to manage the size of the MemoryStream
appropriately, as it will consume memory resources based on the data stored in it. Be mindful of memory limitations, especially when dealing with large amounts of data.
Save Images into Database Using MemoryStream in C#
This is one of the use cases of using the memorystream in C#. As part of this demo project, we will be reading and writing pdf files into the MySQL database.
Database table for storing the image along with some other properties. As you see in the image, Datatype for the resume column is in binary(max). That will be used for storing any kind of file(image, pdf, or ms word) file.
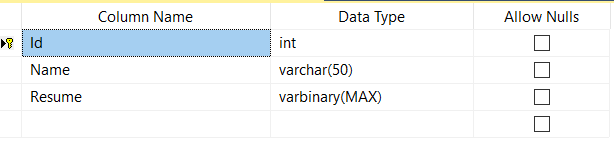
using System; using System.IO; using System.Net.Sockets; using Microsoft.EntityFrameworkCore; namespace Demo.App { class Program { static void Main(string[] args) { using var fs = new FileStream(@"C:\Users\DKU127\Desktop\Reusme.pdf", FileMode.Open); using var ms = new MemoryStream(); fs.CopyTo(ms); SaveEmployeeDetails("Deependra", ms.ToArray()); Console.ReadKey(); } private static void SaveEmployeeDetails(string EmployeeName, byte[] bytes) { var employee = new Employee() { Name = EmployeeName, Resume = bytes }; var context = new EmployeeContext(); context.Employees.Add(employee); context.SaveChanges(); } } }
As we see in the above piece of code. FileStream will help us to read the physical file from the device, and memorystream will use the filestream to read all the bytes and provides an array of bytes.

We can validate the saved entry by navigating to the SQL server and querying the [Employees] table. So we are done with writing the file into the SQL server database.
Convert C# Memorystream to File
Certainly! You can convert the data stored in a MemoryStream
to a file by writing the bytes from the MemoryStream
to a physical file using the FileStream
class. Here’s how you can do it.
using System; using System.IO; class Program { static void Main() { // Create a MemoryStream with some data byte[] dataToWrite = Encoding.UTF8.GetBytes("Data from MemoryStream to file."); MemoryStream memoryStream = new MemoryStream(dataToWrite); // Specify the path for the output file string filePath = "output.txt"; // Write the content of the MemoryStream to a file using FileStream using (FileStream fileStream = new FileStream(filePath, FileMode.Create)) { memoryStream.CopyTo(fileStream); } Console.WriteLine("MemoryStream data has been written to the file."); } }
- Create a
MemoryStream
and populate it with some data (dataToWrite
). - Define a file path for the output file (in this case, “output.txt”).
- Use a
FileStream
to create or overwrite the output file. - Use the
CopyTo
method to copy the data from theMemoryStream
to theFileStream
.
Remember to include error handling and proper disposal of resources (like using using
statements) to ensure that the code works reliably and efficiently.
After running the code, you’ll find the content of the MemoryStream
saved in the specified output file (“output.txt” in this case).
Keep in mind that this is a basic example. Depending on your requirements, you might need to handle exceptions, use different file access modes, or work with different data formats.
Convert a Stream to byte array in C#
To convert a Stream
into a byte[]
array in C#, you can use the MemoryStream
class to read the data from the Stream
and store it in a byte array.Here’s an example of how you can convert a Stream
into a byte[]
array.
using System; using System.IO; class Program { static void Main() { // Assuming you have a Stream object called "inputStream" Stream inputStream = GetInputStream(); // Create a MemoryStream to read the data from the Stream using (MemoryStream memoryStream = new MemoryStream()) { // Copy the contents of the Stream to the MemoryStream inputStream.CopyTo(memoryStream); // Get the byte array from the MemoryStream byte[] byteArray = memoryStream.ToArray(); // Do something with the byte array Console.WriteLine("Byte array length: " + byteArray.Length); } } static Stream GetInputStream() { // Return your desired Stream object here // For example, you can create a FileStream or a NetworkStream // This is just a placeholder method for demonstration purposes return new MemoryStream(Encoding.UTF8.GetBytes("Hello, Stream!")); } }
In this example, we assume you have a Stream object called inputStream. You can replace the GetInputStream() method with your own logic to obtain the desired Stream. Here, we use a MemoryStream as a placeholder to demonstrate the conversion process.
Inside the using
statement, we create a MemoryStream
call memoryStream and use the CopyTo() method to copy the contents of inputStream to the memoryStream. Then, we use the ToArray() method memoryStream
to retrieve the byte array.
Finally, you can perform any desired operations with the resulting byte[]
array. In the example, we simply print the length of the array to the console.
Remember to handle exceptions and dispose of them MemoryStream
and other disposable objects appropriately in a real-world scenario.
Convert Bytes Array to Stream in C#
In C#, you can easily convert a byte array to a stream using the MemoryStream
class, which provides a convenient way to work with streams in memory. By initializing a MemoryStream
with the byte array, you can seamlessly work with the byte data as if it were a stream. This is particularly useful when you need to interface with APIs or libraries that expect data in stream format.
using System; using System.IO; class Program { static void Main() { // Sample byte array byte[] byteArray = new byte[] { 65, 66, 67, 68, 69 }; // Convert byte array to a MemoryStream using (MemoryStream memoryStream = new MemoryStream(byteArray)) { // You can now work with 'memoryStream' as if it were any other stream // For example, read from it, write to it, or pass it to methods requiring a stream Console.WriteLine("Byte array converted to a MemoryStream."); } } }
In this example, the byte array “byteArray” is converted to a MemoryStream
, and you can then use the memoryStream
as if it were any other stream, performing read and write operations on it. After you’re done with the stream, it’s wrapped in a using
statement to ensure proper disposal of resources.
Convert C# MemoryStream to String
In C#, you can convert the content of a MemoryStream
to a string using the Encoding
class. Here’s how you can do it:
using System; using System.IO; using System.Text; class Program { static void Main() { // Create a MemoryStream with some data byte[] dataBytes = Encoding.UTF8.GetBytes("This is the content of MemoryStream."); using (MemoryStream memoryStream = new MemoryStream(dataBytes)) { // Convert the content of the MemoryStream to a string string content = Encoding.UTF8.GetString(memoryStream.ToArray()); // Now you can work with the 'content' string Console.WriteLine(content); } } }
In this example, the byte content of the MemoryStream
is converted to a string using Encoding.UTF8.GetString()
. This works when the content of the MemoryStream
is encoded using UTF-8. If a different encoding was used, you would need to adjust the encoding parameter accordingly.
Remember that working with character encodings is important to ensure that the byte data is correctly interpreted as characters. Always use the appropriate encoding that matches the encoding used when writing the data to the MemoryStream
.
Memorystream vs FileStream
Both MemoryStream
and FileStream
are classes in C# that deal with streams, but they serve different purposes based on where the data is stored and how it is accessed.
Aspect | MemoryStream | FileStream |
---|---|---|
Storage | In-memory byte array | On-disk file |
Use Case | Working with data in memory | Reading/writing files on disk |
Performance | Faster I/O operations | Slower I/O operations |
Scalability | Less suitable for very large data | Suitable for larger files |
Lifecycle | Managed by .NET framework | Requires manual opening/closing |
Remember that the choice between MemoryStream
and FileStream
depends on your specific use case and requirements.
Dispose MemotyStream Object
In C#, it’s important to dispose of a MemoryStream
(and other similar disposable objects) to ensure proper resource management and to prevent potential memory leaks. Disposing of objects properly is a good practice that helps your application run efficiently and reliably.
To ensure proper disposal, it’s recommended to use the using
statement, which automatically calls the Dispose
method when you’re done with the object:
using (MemoryStream memoryStream = new MemoryStream()) { // Work with the memoryStream } // memoryStream.Dispose() is automatically called here
If you don’t use the using
statement, remember to call the Dispose
method manually:
MemoryStream memoryStream = new MemoryStream(); try { // Work with the memoryStream } finally { memoryStream.Dispose(); }
Overall, proper disposal of MemoryStream
instances is a crucial part of writing robust and efficient code in C#.
Conclusion
Overall, C# MemoryStream is a versatile and powerful tool for working with data streams in memory and is widely used in many different types of applications, from web development to desktop applications to mobile app development.
Comments are closed.