C# is a powerful programming language that offers a wide array of features and data structures to work with. One such data structure is the ReadOnly List, which was introduced to provide a convenient way to work with collections that should not be modified after initialization. In this article, we will explore what a ReadOnly List is, its benefits, and how to use it effectively in C#.
What is a ReadOnly List?
A ReadOnly List is a collection in C# that represents an immutable list of elements. Once initialized, the contents of a ReadOnly List cannot be modified. It is part of the System.Collections.Immutable namespace, which is available as a NuGet package and is often used for creating immutable data structures in C#.
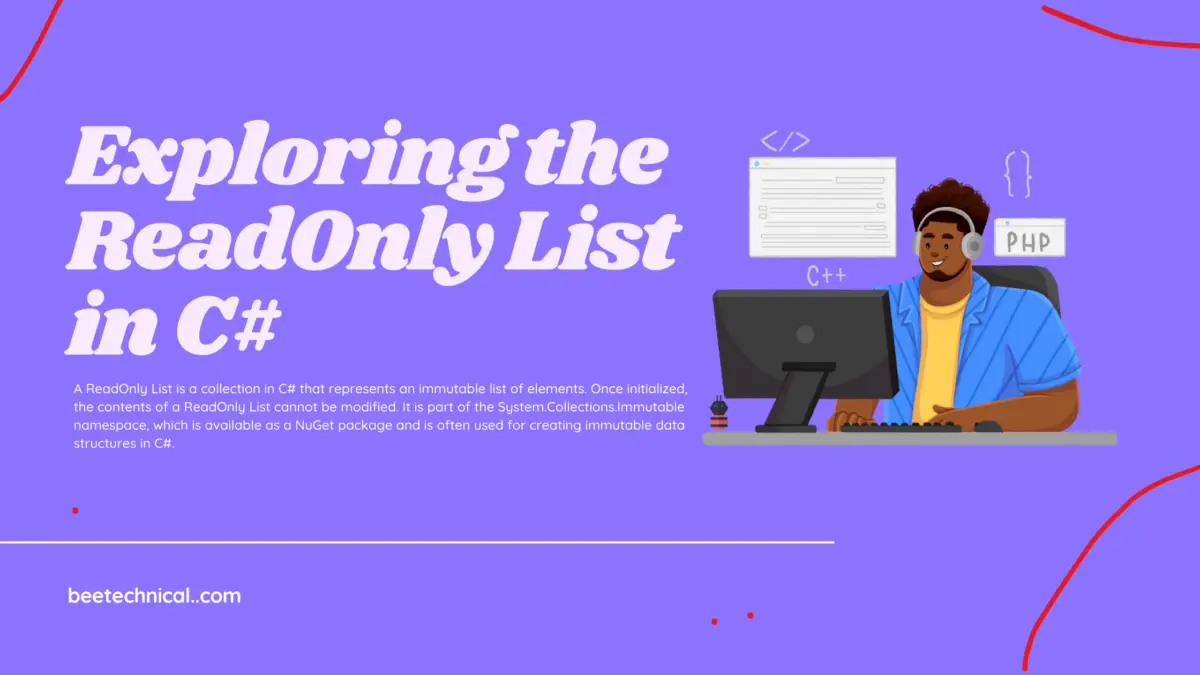
Benefits of using a ReadOnly List
- Immutability: The primary benefit of using a ReadOnly List is that it ensures immutability. Once the list is created, it cannot be changed. This property makes it useful in scenarios where you want to ensure that a collection remains constant throughout its lifetime, preventing unintentional modifications.
- Thread Safety: ReadOnly Lists are inherently thread-safe because they cannot be modified after initialization. This feature simplifies concurrent programming, as you don’t need to worry about synchronization issues when accessing the list from multiple threads.
- Predictable Behavior: With a ReadOnly List, you can be confident that the data won’t change unexpectedly. This predictability is essential in scenarios where maintaining data integrity is critical, such as caching or sharing data between different parts of an application.
Creating a ReadOnly List
To create a ReadOnly List in C#, you can use the ImmutableList<T> class from the System.Collections.Immutable namespace.
using System.Collections.Immutable; using System; class Program { static void Main() { var readOnlyList = ImmutableList<string>.Empty.Add("apple").Add("banana").Add("cherry"); foreach (var item in readOnlyList) { Console.WriteLine(item); } } }
In this example, we create a ReadOnly List of strings and initialize it with three elements. Once created, you cannot modify the contents of readOnlyList
.
Working with a ReadOnly List
While you cannot modify the contents of a ReadOnly List directly, you can perform various operations on it, such as filtering, mapping, or sorting, to obtain new ReadOnly Lists with modified data. These operations return new ReadOnly Lists, leaving the original list unchanged.
var fruitsStartingWithA = readOnlyList.Where(fruit => fruit.StartsWith("a", StringComparison.OrdinalIgnoreCase)).ToImmutableList();
In the code snippet above, we create a new ReadOnly List fruitsStartingWithA
containing fruits that start with the letter ‘A’, without modifying the original list.
Diffrent Use Cases for ReadOnly List
C# ReadOnly Lists are particularly useful in various scenarios where you want to ensure immutability and prevent unintentional modifications to a collection. Here are some common use cases for ReadOnly Lists.
Configuration Data: When you need to store configuration settings for your application, using a ReadOnly List ensures that these settings remain constant throughout the application’s execution. This prevents accidental changes to critical configuration values.
public static readonly ImmutableList<string> AppSettings = ImmutableList<string>.Empty .Add("ServerAddress=example.com") .Add("Port=8080") .Add("MaxConnections=100");
Caching: In caching mechanisms, you might want to store a collection of cached items that should not change while they are in the cache. ReadOnly Lists ensure that the cache contents are consistent and predictable.
public static readonly ImmutableList<CacheItem> Cache = ImmutableList<CacheItem>.Empty;
API Responses: When designing APIs, you may return data as a ReadOnly List to indicate that the response is read-only. This can help clients understand that they should not attempt to modify the received data.
public IActionResult GetItems() { var items = GetItemsFromDatabase(); return Ok(items.ToImmutableList()); }
- Immutable State: In applications that use a state management pattern like Redux in web development or a similar pattern in desktop applications, ReadOnly Lists can represent the application state, ensuring that state updates are handled through well-defined actions and reducers.
- Multithreaded Environments: In multithreaded applications, ReadOnly Lists offer a thread-safe way to share data between threads without the need for explicit locking mechanisms. This ensures data integrity and minimizes the risk of race conditions.
public class DataProcessor { private readonly ImmutableList<int> data; public DataProcessor(IEnumerable<int> initialData) { data = initialData.ToImmutableList(); } public int SumData() { return data.Sum(); } }
Data Transfer Objects (DTOs): When you create DTOs to transfer data between different parts of your application, using ReadOnly Lists can signal that the DTO is intended for reading only, not modification.
public class UserDTO { public string Name { get; } public ImmutableList<string> Roles { get; } public UserDTO(string name, IEnumerable<string> roles) { Name = name; Roles = roles.ToImmutableList(); } }
In all these scenarios, ReadOnly Lists help maintain data integrity and prevent accidental modifications, leading to more robust and predictable code. They are a valuable tool in your C# programming arsenal when you need to work with immutable collections.
Performance Considerations
ReadOnly Lists in C# are designed for performance. They use structural sharing to create new instances efficiently, especially when you perform operations that result in changes. This structural sharing minimizes memory overhead and ensures that common operations like adding or removing elements have near-constant time complexity.
Conclusion
C# ReadOnly Lists are a valuable addition to the language for scenarios where immutability, thread safety, and predictable behavior are essential. By using the System.Collections.Immutable namespace and the ImmutableList<T> class, you can create and work with collections that provide these benefits while maintaining good performance. When designing your C# applications, consider using ReadOnly Lists to enhance data integrity and simplify your code.