Moq and Xunit are two powerful frameworks that, when combined, provide a robust and efficient solution for unit testing in .NET. Moq offers a flexible and expressive mocking framework, while Xunit provides a simple and extensible testing framework.
In this guide, we will delve into the depths of Moq and Xunit, exploring their features, integration techniques, best practices, and real-world examples.
Whether you are new to unit testing or looking to improve your skills, this guide will provide you with the information and tools you need to write complete tests using Moq and Xunit, allowing you to design strong and reliable software systems. So, let us begin our quest to learn the art of unit testing with Moq and Xunit!
What is Moq?
Moq is a popular open-source mocking library for.NET developers, particularly those working in C# and VB.NET. It is intended to simplify and facilitate the creation of mock objects in unit tests. By building fake implementations of such dependencies, Moq allows you to separate the code being tested from its dependencies.
Why to use Moq?
Moq, and mocking libraries in general, have a number of advantages that make them useful tools for unit testing.
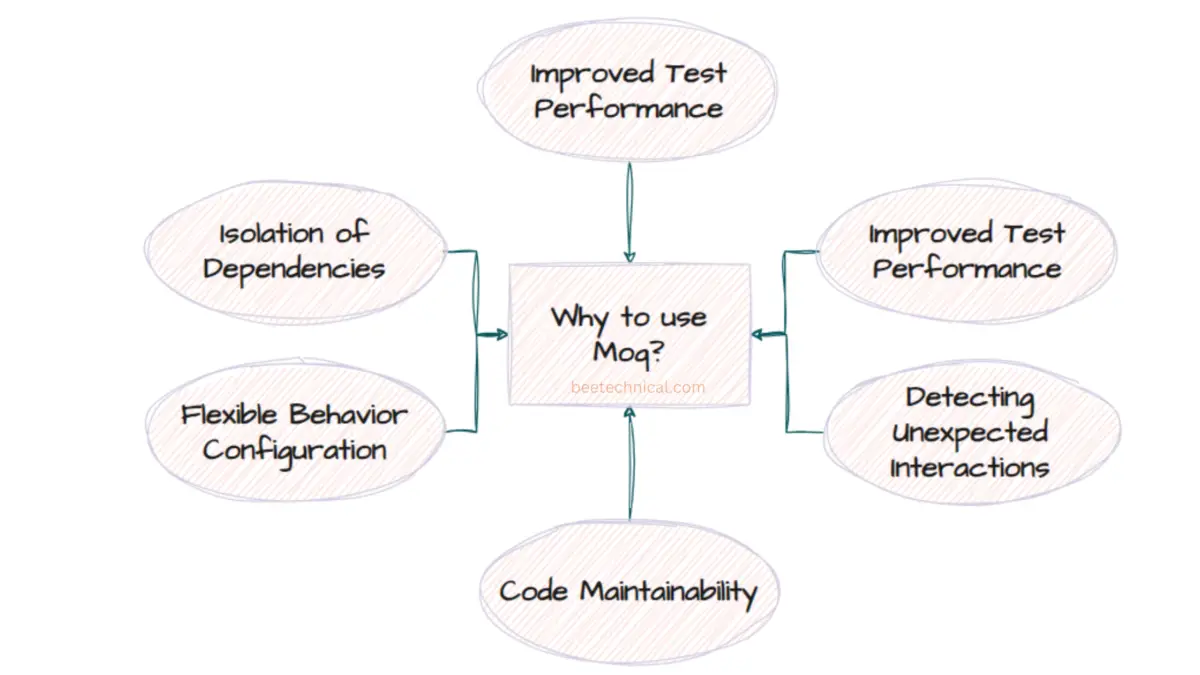
- Isolation of Dependencies: It is critical to isolate the unit of code being tested from its dependencies (such as databases, web services, or external APIs) during unit testing. Mocking frameworks such as Moq enable you to generate mock implementations of these dependencies, allowing the tests to focus entirely on the behaviour of the unit under test.
- Flexible Behavior Configuration: Moq allows you to configure different behaviours for your mock objects. When a method is called, you may specify what it should return, throw exceptions, and even set up callbacks to do custom actions. This adaptability allows you to simulate many circumstances, making it easier to test edge cases and error handling.
- Improved Test Performance: Mock objects are in-memory representations of dependencies that are lightweight. Because there is no need to contact external resources or conduct complex actions when utilising mocks instead of actual implementations, your tests will run faster. Faster test execution and feedback cycles result from enhanced performance.
- Detecting Unexpected Interactions: During test execution, Moq maintains historical records of interactions with mock objects. This feature helps you to ensure that the intended methods were invoked with the appropriate parameters. You can also assert that specific methods were not called at all, which can help you detect unexpected or redundant actions.
- Code Maintainability: Using Moq fosters improved code architecture and the use of dependency inversion and dependency injection principles. When you design code with testability in mind, you develop more modular and loosely linked components, which leads to better code maintainability and flexibility.
Setup xUnit in .Net Core
Let’s begin by creating the project that will be used throughout the tutorial to explore the features of the Moq framework.
Follow the steps as per the IDE being used. I am using visual studio for demo this. I have setup an .Net Core 6 API with layered archotecture to demostrate the usage of Moq.
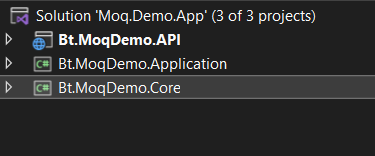
It consists of three projects: Core, Application, and API. The core layer will contain the core classes or domain classes, while the application layer will have the logic or services to save the information sent from the API to persistent storage utilising the infrastructure layer. Which we will introduce in the following steps.
We want to build an api to provide the information about the movies and there ratting like IMDB system. And,Let’s introduce our first class in the core project to get started.
namespace Bt.MoqDemo.Core; public class Movie { public string Title { get; } public string Description { get; } public DateTime ReleaseDate { get; } public double Rating { get; private set; } public Movie(string title, string description, DateTime releaseDate) { ArgumentNullException.ThrowIfNull(title); ArgumentNullException.ThrowIfNull(description); ArgumentNullException.ThrowIfNull(releaseDate); Title = title; Description = description; ReleaseDate = releaseDate; Rating = 0; } public void SetRating(double rating) { if (rating < 0) { throw new ArgumentException("Rating cannot be negative."); } Rating = rating; } }
So we wrote our first class, which will be used to store information about the movie.And this isn’t just a simple class for storing data in a database. Rather, it is a rich model that holds the valdiations to never enable the model to be in an incorrect state. Let’s begin by making our first xUnit project to test this class.
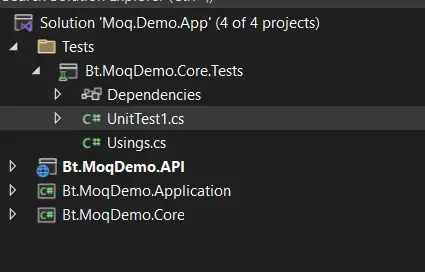
Let’s begin by renaming the auto-generated class to MovieTests
. Then, we’ll proceed to write test cases for the Movie
class. To enhance our testing approach, we’ll utilize the Theory
attribute along with the InlineData
attribute, as we discussed in our previous article on Efficient Xunit with Theory and Inline Data.
namespace Bt.MoqDemo.Core.Tests; public class MovieTests { [Fact] public void CreateMovie_ValidInput_ReturnsMovieObject() { // Arrange string title = "Sample Movie"; string description = "This is a sample movie."; DateTime releaseDate = new DateTime(2023, 7, 1); // Act var movie = new Movie(title, description, releaseDate); // Assert Assert.NotNull(movie); Assert.Equal(title, movie.Title); Assert.Equal(description, movie.Description); Assert.Equal(releaseDate, movie.ReleaseDate); } [Theory] [InlineData(null, "This is a sample movie.", "Value cannot be null. (Parameter 'title')")] [InlineData("Sample Movie", null, "Value cannot be null. (Parameter 'description')")] public void CreateMovie_InvalidInput_ThrowsArgumentException(string title, string description, string errorMessage) { // Arrange DateTime releaseDate = new DateTime(2023, 7, 1); // Act & Assert var ex = Assert.Throws<ArgumentNullException>(() => new Movie(title, description, releaseDate)); Assert.Equal(errorMessage, ex.Message); } [Fact] public void SetRating_ValidRating_SetsRating() { // Arrange var movie = new Movie("Sample Movie", "This is a sample movie.", new DateTime(2023, 7, 1)); double rating = 4.5; // Act movie.SetRating(rating); // Assert Assert.Equal(rating, movie.Rating); } [Theory] [InlineData(-1)] [InlineData(-3.5)] public void SetRating_InvalidRating_ThrowsArgumentException(double rating) { // Arrange var movie = new Movie("Sample Movie", "This is a sample movie.", new DateTime(2023, 7, 1)); // Act & Assert var ex = Assert.Throws<ArgumentException>(() => movie.SetRating(rating)); Assert.Equal("Rating cannot be negative.", ex.Message); } }
These test cases will run perfectly fine wihtout any issues. Let’s run the test cases.
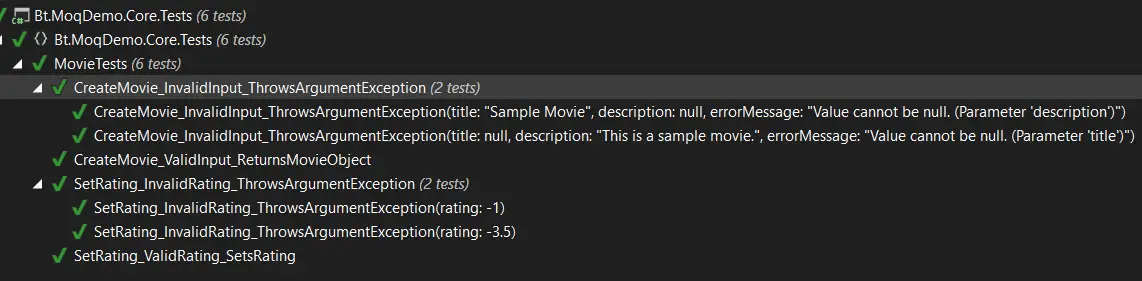
Let’s move on to enhancing our demo application by introducing new features. After successfully designing the data model and conducting thorough unit testing, the next step is to implement data persistence.
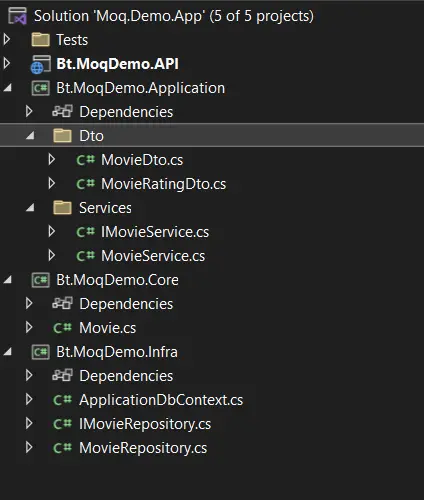
Indeed, our project has undergone significant changes. Previously, we lacked the Infrastructure layer responsible for persistence.
Now, with its introduction, we have the means to store data securely in a database. Additionally, we have incorporated the Service class, which serves as an intermediary between our application and the API and also handles data persistence.
Now that we have added more features and introduced multiple layers in our application, testing has become more complex. To tackle this, we can leverage the Moq framework, which will be incredibly helpful.
By using Moq
, we can create mock implementations of dependencies, such as the MovieRepository, when testing the MovieService. This way, we can control the behavior of the repository during testing, ensuring that the Service class operates as expected without relying on the actual database.
Setting Up Moq
Setting up Moq is a straightforward process, and it involves installing the Moq library via NuGet package manager. Moq is available as a free and open-source package and can be used with most .NET frameworks and versions.
Open nuget package manager and look for Moq.
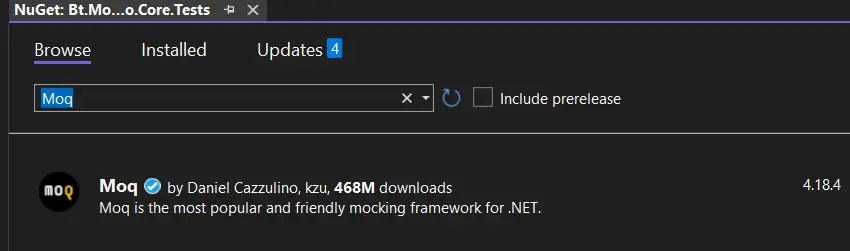
You have to install the first nuget package built by Daniel Cazzulino, it has around 468 millions download.
Alternativly you can install by the package manager tool.
NuGet\Install-Package Moq -Version 4.18.4
Create Mock Objects
In this section, we will explore the process of creating mock objects using the Moq library. Mock objects are powerful tools for testing, and Moq provides a convenient way to generate them. We’ll delve into how to use Moq to create mock objects to simulate dependencies and interactions within our tests.
Mocking Interfaces
Mocking interfaces is a common scenario in unit testing, where we use mock objects to simulate the behavior of interface implementations.When testing classes that depend on these interfaces, we can use Moq to create mock objects that mimic the interface’s behavior without relying on the actual implementations.
Let’s add some test cases for the MovieService, which adds, updates, and rates movies and stores the data in a database.For the reason of covering the unit test cases for the service layer, we can add one more project to this solution.
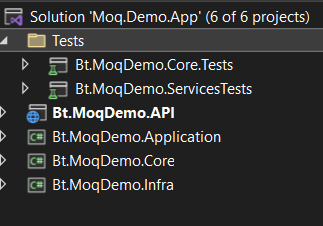
using Bt.MoqDemo.Application.Dto; using Bt.MoqDemo.Application.Services; using Bt.MoqDemo.Core; using Bt.MoqDemo.Infra; using Moq; namespace Bt.MoqDemo.ServicesTests; public class MovieServiceTests { private readonly Mock<Logger> _loggerMock; private readonly Mock<IMovieRepository> _movieRepositoryMock; public MovieServiceTests() { _loggerMock = new Mock<Logger>(); _movieRepositoryMock = new Mock<IMovieRepository>(); } [Fact] public void AddMovie_Should_Add_Movie_To_Repository() { // Arrange var movieDto = new MovieDto { Title = "Test Movie", Description = "This is a test movie.", ReleaseDate = new DateTime(2023, 7, 1) }; _movieRepositoryMock.Setup(repo => repo.Add(It.IsAny<Movie>())).Verifiable(); _movieRepositoryMock.Setup(repo => repo.SaveChanges()).Verifiable(); var movieService = new MovieService(_movieRepositoryMock.Object, _loggerMock.Object); // Act var result = movieService.AddMovie(movieDto); // Assert _movieRepositoryMock.Verify(); // Verifies that Add and SaveChanges were called Assert.NotNull(result); Assert.NotEqual(Guid.Empty, result.Id); Assert.Equal(0, result.Rating); // Assuming the default rating is 0 } [Fact] public void UpdateMovie_Should_Update_Existing_Movie() { // Arrange var existingMovie = new Movie("Existing Movie", "Old Description", new DateTime(2022, 1, 1)) { Id = Guid.NewGuid() }; var movieDto = new MovieDto { Id = existingMovie.Id, Title = "Updated Movie", Description = "New Description", ReleaseDate = new DateTime(2023, 8, 15) }; _movieRepositoryMock.Setup(repo => repo.GetById(existingMovie.Id)).Returns(existingMovie); _movieRepositoryMock.Setup(repo => repo.SaveChanges()).Verifiable(); var movieService = new MovieService(_movieRepositoryMock.Object, _loggerMock.Object); // Act var result = movieService.UpdateMovie(movieDto); // Assert _movieRepositoryMock.Verify(); // Verifies that GetById and SaveChanges were called Assert.NotNull(result); Assert.Equal(movieDto.Title, existingMovie.Title); Assert.Equal(movieDto.Description, existingMovie.Description); Assert.Equal(movieDto.ReleaseDate, existingMovie.ReleaseDate); Assert.Equal(existingMovie.Rating, result.Rating); // Rating should remain the same } [Fact] public void RateMovie_Should_Update_Movie_Rating() { // Arrange var movieId = Guid.NewGuid(); var movieRatingDto = new MovieRatingDto { MovieId = movieId, Rating = 8.5 }; var existingMovie = new Movie("Test Movie", "Description", new DateTime(2023, 7, 1)) { Id = movieId }; _movieRepositoryMock.Setup(repo => repo.GetById(movieId)).Returns(existingMovie); _movieRepositoryMock.Setup(repo => repo.SaveChanges()).Verifiable(); var movieService = new MovieService(_movieRepositoryMock.Object, _loggerMock.Object); // Act movieService.RateMovie(movieRatingDto); // Assert _movieRepositoryMock.Verify(); // Verifies that GetById and SaveChanges were called Assert.Equal(movieRatingDto.Rating, existingMovie.Rating); // Rating should be updated to 8.5 } }
In this example, we have a MovieService
class that provides functionality for adding, updating, and rating movies. The class depends on an IMovieRepository
interface, which defines the contract for interacting with the movie data store. We will use Moq to mock the IMovieRepository
interface to test the MovieService
class in isolation.
Mocking Classes
Let’s begin by writing some bad code. Although I think this is a poor example, there are times when legacy code—code that we don’t introduce interfaces to—may have examples of this kind.
Within the MovieService class, we have integrated the Logger class, responsible for logging messages to the console. However, this Logger class does not expose any interface externally.
using Bt.MoqDemo.Application.Dto; using Bt.MoqDemo.Core; using Bt.MoqDemo.Infra; namespace Bt.MoqDemo.Application.Services; public class MovieService { private readonly IMovieRepository _movieRepository; private readonly Logger _logger; public MovieService(IMovieRepository movieRepository,Logger logger) { _movieRepository = movieRepository; _logger = logger; } public MovieDto AddMovie(MovieDto movieDto) { var movie = new Movie(movieDto.Title, movieDto.Description, movieDto.ReleaseDate); _movieRepository.Add(movie); _movieRepository.SaveChanges(); _logger.Log($"New Movie Added:{movieDto.Title}"); movieDto.Id = movie.Id; movieDto.Rating = movie.Rating; return movieDto; } public MovieDto UpdateMovie(MovieDto movieDto) { var movie = _movieRepository.GetById(movieDto.Id); if (movie == null) { throw new ArgumentException("Movie not found."); } movie.Title = movieDto.Title; movie.Description = movieDto.Description; movie.ReleaseDate = movieDto.ReleaseDate; _movieRepository.SaveChanges(); _logger.Log($"Movie Updated:{movieDto.Title}"); movieDto.Rating = movie.Rating; return movieDto; } public void RateMovie(MovieRatingDto movieRatingDto) { var movie = _movieRepository.GetById(movieRatingDto.MovieId); if (movie == null) { throw new ArgumentException("Movie not found."); } movie.SetRating(movieRatingDto.Rating); _movieRepository.SaveChanges(); _logger.Log($"Rating Provided for Movie:{movie.Title}"); } }
We have introduced the additional depedency on the MovieService class which has to be fixed in our unti test cases as well. Let’s update the MovieServiceTests
class.
private readonly Mock<Logger> _loggerMock; private readonly Mock<IMovieRepository> _movieRepositoryMock; public MovieServiceTests() { _loggerMock = new Mock<Logger>(); _movieRepositoryMock = new Mock<IMovieRepository>(); }
There is not diffrent while creating a mock object for interface vs concrete class using Moq framework. As see in the above example.
Setting Expectations
Setting expectations in a mock involves specifying what behavior or method calls you expect to occur on the mock object during a unit test. This ensures that the code being tested interacts with the mock object as intended and that the expected interactions are taking place.
Verifying Method Calls
Verifying method calls on mock objects is an essential part of unit testing with mocks. It allows you to ensure that the methods you expect to be called during the test have indeed been called with the correct parameters and number of times.
By verifying method calls, you can check whether your code under test interacts correctly with its dependencies.
Let’s take an exmaple of MovieService Tests. Where we are testing the Ratign movie method.
[Fact] public void RateMovie_Should_Update_Movie_Rating() { // Arrange var movieId = Guid.NewGuid(); var movieRatingDto = new MovieRatingDto { MovieId = movieId, Rating = 8.5 }; var existingMovie = new Movie("Test Movie", "Description", new DateTime(2023, 7, 1)) { Id = movieId }; _movieRepositoryMock.Setup(repo => repo.GetById(movieId)).Returns(existingMovie); _movieRepositoryMock.Setup(repo => repo.SaveChanges()).Verifiable(); var movieService = new MovieService(_movieRepositoryMock.Object, _loggerMock.Object); // Act movieService.RateMovie(movieRatingDto); // Assert _movieRepositoryMock.Verify(); // Verifies that GetById and SaveChanges were called Assert.Equal(movieRatingDto.Rating, existingMovie.Rating); // Rating should be updated to 8.5 }
Invoking the _movieRepositoryMock.Verify(); code to verify that method on the mock to ensure that the GetById
and SaveChanges
methods were called exactly once as expected.
In Moq, there are several Verify
methods that allow you to assert different aspects of the method calls on mock objects.
Verifies that a method on the mock object was called at least once. It does not specify the number of times the method should be called.
mockObject.Verify(mock => mock.MethodName(), Times.AtLeastOnce);
Verifies that a method on the mock object was called a specific number of times.
mockObject.Verify(mock => mock.MethodName(), Times.Exactly(3));
Verifies that a method on the mock object was never called.
mockObject.Verify(mock => mock.MethodName(), Times.Never);
Verifies that a method on the mock object was called at least once with specific arguments.
mockObject.Verify(mock => mock.MethodName(arg1, arg2), Times.AtLeastOnce);
Verifies that a method on the mock object was called with specific arguments a specific number of times.
mockObject.Verify(mock => mock.MethodName(arg1, arg2), Times.Exactly(3));
Verifies that no other methods on the mock object were called during the test.
mockObject.VerifyNoOtherCalls();
Setting Return Values
In Moq, you can set return values for methods on mock objects to control the behavior of the mock during testing. This allows you to define specific responses that the mock should return when its methods are called with specific arguments.
Setting return values on mock methods is particularly useful when you want to simulate different scenarios in your unit tests.
Let’s add another method to the Movie service class that will offer us movies with ratings beyond four.
IEnumerable<MovieDto> HighRatedMovies();
Also, implement the same in the service class. The repository class must also be extended in order to offer a list of movies with ratings higher than four.
public IEnumerable<MovieDto> HighRatedMovies() { var movies = _movieRepository.GetMoviesWhereRatingMoreThan(4).ToList(); var moviesDto = movies.Select(x => new MovieDto() { Title = x.Title, Rating = x.Rating, Description = x.Description, ReleaseDate = x.ReleaseDate }); return moviesDto; }
Let’s write some unit test cases to validate the funcaiontlity of this method.We need to upadte the unit test cases in the MovieService class.
[Fact] public void HighRatedMovies_Should_Return_High_Rated_Movies() { // Arrange var highRatedMovies = new List<Movie> { new Movie("The Shawshank Redemption", "Two imprisoned men bond over several years..", new DateTime(1994, 9, 23)), new Movie("The Godfather", "The aging patriarch of an organized crime dynasty...", new DateTime(1972, 3, 24)), new Movie("The Dark Knight", "When the menace known as The Joker emerges...", new DateTime(2008, 7, 18)), new Movie("Pulp Fiction", "The lives of two mob hitmen, a boxer, a gangster's ...", new DateTime(1994, 10, 14)) }; highRatedMovies.ForEach(x => { x.SetRating(4); }); var movieRepositoryMock = new Mock<IMovieRepository>(); movieRepositoryMock.Setup(repo => repo.GetMoviesWhereRatingMoreThan(4)).Returns(highRatedMovies); var movieService = new MovieService(movieRepositoryMock.Object, new Mock<Logger>().Object); // Act var result = movieService.HighRatedMovies(); // Assert Assert.NotNull(result); Assert.Equal(4, result.Count()); Assert.True(result.All(movie => movie.Rating > 3)); // Check if all movies have a rating more than 4 }
To set a return value for the GetMoviesWhereRatingMoreThan
method on the mock IMovieRepository
, we use the Setup
method with the Returns
clause:
movieRepositoryMock.Setup(repo => repo.GetMoviesWhereRatingMoreThan(4)).Returns(highRatedMovies);
Here, we specified that when the GetMoviesWhereRatingMoreThan
method is called on the mock with a rating greater than 4, it should return the highRatedMovies
list that we created in the test setup.
In Moq, you have several options to set return values for methods on mock objects depending on your testing needs. Let’s explore some of the common options for setting return values:
Returns
: This option allows you to specify a fixed value to return when the method is called.
mockObject.Setup(x => x.MethodName()).Returns(someValue);
ReturnsAsync
: Similar to Returns
, but specifically used for methods that return a Task<T>
. Useful for testing asynchronous code.
mockObject.Setup(x => x.MethodNameAsync()).ReturnsAsync(someValue);
Returns
with Lambda: You can use a lambda expression to calculate the return value based on the input arguments to the method.
mockObject.Setup(x => x.MethodName(It.IsAny<string>())).Returns((string input) => CalculateReturnValue(input));
Throws
: This option allows you to throw an exception when the method is called. Useful for testing error-handling scenarios.
mockObject.Setup(x => x.MethodName()).Throws(new Exception("Some error occurred."));
Callback
: Use this option to perform custom actions or operations when the method is called.
mockObject.Setup(x => x.MethodName()).Callback(() => DoSomething());
ReturnsInOrder
: If you want the mock to return different values in a sequence, you can use this option.
mockObject.Setup(x => x.MethodName()).ReturnsInOrder(value1, value2, value3);
Returns<T>
: This allows you to create a custom sequence of return values.
var sequence = new Queue<T>();
sequence.Enqueue(value1);
sequence.Enqueue(value2);
mockObject.Setup(x => x.MethodName()).Returns(sequence.Dequeue);
Handling Out and Ref Parameters
In Moq, you can handle methods with out
and ref
parameters by using the out
and ref
keywords in the Setup
method to define the behavior of the mock when these methods are called during testing.
Let’s demonstrate how to handle methods with out
and ref
parameters in Moq with examples:
We can add another method in MovieService class which does calculate the average rating of the movie.
bool TryCalculateAverageRating(Guid movieId, out double averageRating, ref int totalRatings);
Raising Events With Moq
In Moq, you can raise events on mock objects to simulate event occurrences and test how your code responds to those events. Mocking events is useful for testing event-driven code or verifying that event handlers are called as expected.
Mock Behavior
In Moq, you can control the behavior of your mock objects by specifying various setups and expectations. Mock behavior determines how the mock object responds when its methods are called during testing.
There are three main behaviors you can set for a mock object in Moq:
- Default: By default, a mock object returns default values for methods. For reference types, it returns
null
, and for value types, it returns their default values (e.g.,0
forint
,false
forbool
, etc.). - Strict: A strict mock enforces strict behavior, which means it will throw an exception for any method call that has not been explicitly set up with a return value or expectation. Strict mocks help catch unintended interactions during testing.
- Loose: A loose mock is more lenient and allows any method to be called without throwing exceptions. If you haven’t set up a specific behavior for a method, it will return default values as described in the default behavior.
To set the behavior of a mock object in Moq, you use the MockBehavior
enumeration when creating the mock.
// Default behavior (loose mock) var mockObject = new Mock<IMyInterface>(); // Strict behavior var strictMockObject = new Mock<IMyInterface>(MockBehavior.Strict);
Mock behavior is particularly useful for setting up expectations and ensuring that your code interacts with dependencies as intended. It helps you create more robust and reliable unit tests by defining precisely how your mock objects should behave during testing.
To demonstrate different mock behaviors using the MovieService
example, we’ll create three test cases:
- Default Behavior (Loose Mock): This test case will use the default behavior, where unconfigured methods will return default values.
- Strict Behavior: This test case will use strict behavior, where unconfigured methods will throw exceptions.
- Custom Behavior: This test case will demonstrate how to set up specific return values for a method on the mock object.
Let’s proceed with the test implementation:
using Moq; using Xunit; public class MovieServiceTests { // MovieService class and other necessary setups... [Fact] public void DefaultBehavior_Should_Return_Default_Values() { // Arrange var movieRepositoryMock = new Mock<IMovieRepository>(); // Default behavior (loose mock) var loggerMock = new Mock<Logger>(); // Default behavior (loose mock) var movieService = new MovieService(movieRepositoryMock.Object, loggerMock.Object); // Act var movieDto = new MovieDto { Title = "Test Movie", Description = "This is a test movie.", ReleaseDate = new DateTime(2023, 7, 1) }; var result = movieService.AddMovie(movieDto); // Assert Assert.Null(result.Id); // Since it is a loose mock, the Id will be the default value (null) Assert.Equal(0, result.Rating); // Since it is a loose mock, the Rating will be the default value (0) } [Fact] public void StrictBehavior_Should_Throw_Exception_For_Unconfigured_Method() { // Arrange var movieRepositoryMock = new Mock<IMovieRepository>(MockBehavior.Strict); var loggerMock = new Mock<Logger>(MockBehavior.Strict); var movieService = new MovieService(movieRepositoryMock.Object, loggerMock.Object); // Act and Assert Assert.Throws<MockException>(() => movieService.AddMovie(null)); // Since it is a strict mock, calling the AddMovie method with null will throw a MockException } [Fact] public void CustomBehavior_Should_Setup_Specific_Return_Value() { // Arrange var movieRepositoryMock = new Mock<IMovieRepository>(); var loggerMock = new Mock<Logger>(); var movieService = new MovieService(movieRepositoryMock.Object, loggerMock.Object); // Setting up custom behavior movieRepositoryMock.Setup(repo => repo.Add(It.IsAny<Movie>())).Returns((Movie movie) => { movie.Id = Guid.NewGuid(); // Set a custom Id before adding movie.Rating = 4.5; // Set a custom Rating before adding return movie; }); // Act var movieDto = new MovieDto { Title = "Test Movie", Description = "This is a test movie.", ReleaseDate = new DateTime(2023, 7, 1) }; var result = movieService.AddMovie(movieDto); // Assert Assert.NotEqual(Guid.Empty, result.Id); // Id should be a custom value set in the setup Assert.Equal(4.5, result.Rating); // Rating should be a custom value set in the setup } }
Mocking Database Calls, Services, and APIs
Mocking database calls, services, and APIs is crucial for writing effective unit tests for applications that interact with external systems. By mocking these interactions, you can isolate your tests from the actual external dependencies, making the tests faster, more reliable, and easier to maintain.
Database Calls
When your code interacts with a database, you can use an in-memory database or a lightweight database framework specifically designed for testing, like SQLite. Alternatively, you can mock the database access layer by creating a mock object for the database context or repository interface.
Example using Entity Framework Core and an in-memory database:
public class MovieRepositoryTests { [Fact] public void GetMoviesByGenre_Should_Return_Movies_From_Database() { // Arrange var options = new DbContextOptionsBuilder<MyDbContext>() .UseInMemoryDatabase(databaseName: "TestDatabase") .Options; using (var context = new MyDbContext(options)) { context.Movies.Add(new Movie { Title = "Movie 1", Genre = "Action" }); context.Movies.Add(new Movie { Title = "Movie 2", Genre = "Action" }); context.Movies.Add(new Movie { Title = "Movie 3", Genre = "Comedy" }); context.SaveChanges(); } using (var context = new MyDbContext(options)) { var movieRepository = new MovieRepository(context); // Act var actionMovies = movieRepository.GetMoviesByGenre("Action"); // Assert Assert.Equal(2, actionMovies.Count()); } } }
External Services Calls
When testing classes that depend on external services, such as email services or third-party APIs, you should create mock versions of those services that implement the same interface as the real services. Mock objects allow you to control the behavior and responses of these services during testing.
Example using a simple email service:
public interface IEmailService { void SendEmail(string to, string subject, string body); } public class MovieService { private readonly IEmailService _emailService; public MovieService(IEmailService emailService) { _emailService = emailService; } public void NotifyUsersAboutNewMovie(string movieTitle) { // Code to notify users about the new movie _emailService.SendEmail("[email protected]", "New Movie Added", $"A new movie '{movieTitle}' has been added!"); } }
Example test using a mock email service:
public class MovieServiceTests { [Fact] public void NotifyUsersAboutNewMovie_Should_Send_Email() { // Arrange var emailServiceMock = new Mock<IEmailService>(); var movieService = new MovieService(emailServiceMock.Object); // Act movieService.NotifyUsersAboutNewMovie("Test Movie"); // Assert emailServiceMock.Verify(mock => mock.SendEmail("[email protected]", "New Movie Added", "A new movie 'Test Movie' has been added!"), Times.Once); } }
Api Calls
When your code makes HTTP requests to external APIs, you can use a library like HttpClient
to interact with the API. During testing, you can mock HttpClient
using HttpMessageHandler
to return predefined responses.
Example using HttpClient
with a mock message handler:
public class MovieApiClient { private readonly HttpClient _httpClient; public MovieApiClient(HttpClient httpClient) { _httpClient = httpClient; } public async Task<MovieDto> GetMovieAsync(Guid movieId) { var response = await _httpClient.GetAsync($"api/movies/{movieId}"); response.EnsureSuccessStatusCode(); return await response.Content.ReadAsAsync<MovieDto>(); } }
Example test using a mock HTTP client:
public class MovieApiClientTests { [Fact] public async Task GetMovieAsync_Should_Return_Movie() { // Arrange var httpClientMock = new Mock<HttpClient>(); httpClientMock.Protected() .Setup<Task<HttpResponseMessage>>("GetAsync", ItExpr.IsAny<string>()) .ReturnsAsync(new HttpResponseMessage { StatusCode = HttpStatusCode.OK, Content = new StringContent("{\"Id\":\"123\",\"Title\":\"Test Movie\",\"Description\":\"This is a test movie.\",\"ReleaseDate\":\"2023-07-01T00:00:00\"}", Encoding.UTF8, "application/json") }); var movieApiClient = new MovieApiClient(httpClientMock.Object); // Act var movieDto = await movieApiClient.GetMovieAsync(Guid.NewGuid()); // Assert Assert.Equal("Test Movie", movieDto.Title); } }
In this example, we use Moq to create a mock HttpClient
and set up a predefined response using the HttpMessageHandler
. This allows us to test the MovieApiClient
class without actually making HTTP requests to the external API.
Mocking Asynchronous Code
Mocking asynchronous code is an essential part of unit testing when dealing with async methods, such as asynchronous API calls, task-based operations, or async database calls. To effectively test asynchronous code, you can use Moq along with Task.FromResult
, Task.Delay
, and TaskCompletionSource
to create mock async responses.
Let’s demonstrate how to mock asynchronous code using Moq with the MovieService
example and a fictional asynchronous repository method.
Suppose we have the following asynchronous method in the IMovieRepository
interface:
public interface IMovieRepository { Task<Movie> GetMovieAsync(Guid movieId); }
And the MovieService
class using the IMovieRepository
public class MovieService { private readonly IMovieRepository _movieRepository; public MovieService(IMovieRepository movieRepository) { _movieRepository = movieRepository; } public async Task<MovieDto> GetMovieAsync(Guid movieId) { var movie = await _movieRepository.GetMovieAsync(movieId); if (movie == null) return null; return new MovieDto { Id = movie.Id, Title = movie.Title, Description = movie.Description, ReleaseDate = movie.ReleaseDate }; } }
Now, let’s create a unit test for the GetMovieAsync
method, mocking the IMovieRepository
asynchronous call:
using Moq; using System; using System.Threading.Tasks; using Xunit; public class MovieServiceTests { [Fact] public async Task GetMovieAsync_Should_Return_MovieDto() { // Arrange var movieId = Guid.NewGuid(); var movieRepositoryMock = new Mock<IMovieRepository>(); var movie = new Movie { Id = movieId, Title = "Test Movie", Description = "This is a test movie.", ReleaseDate = new DateTime(2023, 7, 1) }; movieRepositoryMock.Setup(repo => repo.GetMovieAsync(movieId)).ReturnsAsync(movie); var movieService = new MovieService(movieRepositoryMock.Object); // Act var result = await movieService.GetMovieAsync(movieId); // Assert Assert.NotNull(result); Assert.Equal(movie.Id, result.Id); Assert.Equal(movie.Title, result.Title); Assert.Equal(movie.Description, result.Description); Assert.Equal(movie.ReleaseDate, result.ReleaseDate); } [Fact] public async Task GetMovieAsync_Should_Return_Null_When_Movie_Not_Found() { // Arrange var movieId = Guid.NewGuid(); var movieRepositoryMock = new Mock<IMovieRepository>(); movieRepositoryMock.Setup(repo => repo.GetMovieAsync(movieId)).ReturnsAsync((Movie)null); var movieService = new MovieService(movieRepositoryMock.Object); // Act var result = await movieService.GetMovieAsync(movieId); // Assert Assert.Null(result); } }
In the first test, we set up the IMovieRepository.GetMovieAsync
method to return a pre-defined Movie
instance. We use ReturnsAsync
to return the result as a Task
. The test then verifies that the GetMovieAsync
method of MovieService
returns the expected MovieDto
.
In the second test, we set up the IMovieRepository.GetMovieAsync
method to return null using ReturnsAsync((Movie)null)
. The test verifies that the GetMovieAsync
method of MovieService
returns null when the movie is not found.
Remember that when testing asynchronous methods, it’s essential to await the async operations properly using await
, ensure that exceptions are handled correctly, and use Moq’s ReturnsAsync
, Task.FromResult
, and Task.CompletedTask
methods to mock async method behavior. This approach ensures that your tests are reliable and provide accurate coverage for your asynchronous code.
Exception handling with Mock
Exception handling with mock objects is a way to test how your code reacts when something goes wrong. In Moq, you can make a mock object throw an error when a specific action is performed on it.
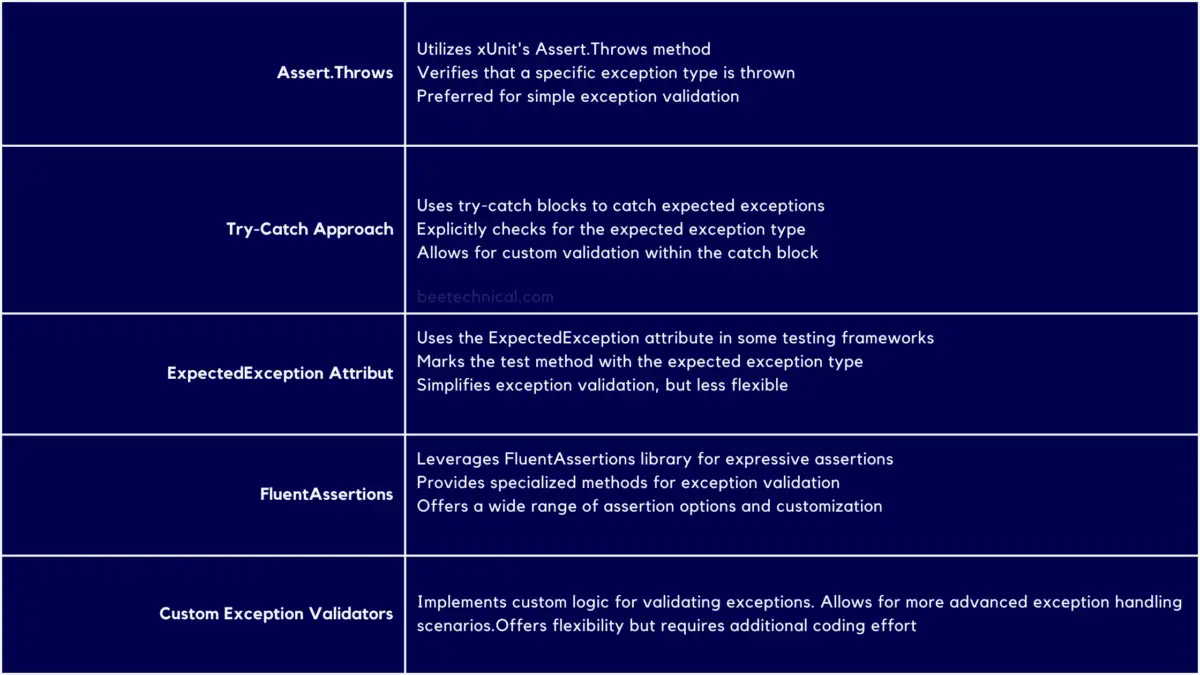
This helps you check if your code handles errors properly. By doing this, you can make sure that your program doesn’t crash or behave unexpectedly when problems occur.
We’ve already extensively covered the topic of managing exceptions through mock objects in a detailed article. https://beetechnical.com/tech-tutorial/xunit-assert-exception/
Advanced Scenarios
In addition to mocking methods and asynchronous methods, there are scenarios where you might need to mock properties, indexes, and even extension methods. These situations arise when you’re dealing with more complex interactions in your codebase.
Mocking Properties
Mocking properties involves creating mock objects that simulate the behavior of properties in a class or interface. This allows you to control the values returned by the properties during testing, ensuring that the properties behave as expected in different scenarios.
Let’s consider an example where we want to mock a property of the Movie
class using Moq. We’ll use the Movie
class that we’ve been using throughout our discussions.
Suppose the Movie
class has a property IsAvailable
that indicates whether a movie is currently available for viewing. We want to mock the behavior of this property to test how our code interacts with it.
Here’s how you can mock a property of the Movie
class using Moq:
using Moq; using Xunit; public class PropertyMockingMovieTests { [Fact] public void Mocking_Movie_Property_Should_Return_Custom_Value() { // Arrange var movieMock = new Mock<Movie>(); movieMock.SetupGet(m => m.IsAvailable).Returns(true); var mockedMovie = movieMock.Object; // Act bool isAvailable = mockedMovie.IsAvailable; // Assert Assert.True(isAvailable); } }
In this example, we create a mock object of the Movie
class using Mock<Movie>
. We then use SetupGet
to define the behavior of the IsAvailable
property, specifying that it should return true
. When we access the IsAvailable
property on the mock object, it returns the value we defined.
Mocking Extension Methods
Mocking extension methods involves simulating the behavior of static methods that are defined as extension methods on certain types. Since extension methods are essentially static methods, they cannot be directly mocked using Moq.
However, you can still effectively test code that relies on extension methods by properly structuring your code and using interfaces and dependency injection.
Let’s modify the MovieService
example to demonstrate how to handle code that relies on an extension method using interfaces and dependency injection.
Suppose we have the following extension method that operates on the IEnumerable<Movie>
type:
namespace Bt.MoqDemo.Core; public static class MovieExtensions { public static IEnumerable<Movie> FilterByRating(this IEnumerable<Movie> movies, double minRating) { return movies.Where(movie => movie.Ratings.Sum() >= minRating); } }
And We are utilizing this extension method inside the movieservice class. Let’s update the method called GetHighRatedMovies in service class to utlize this method.
public async Task<IEnumerable<MovieDto>> HighRatedMovies() { var movies = await _movieRepository.GetAllMovies(); var highRatedMovies = movies.FilterByRating(4); return highRatedMovies.Select(movie => new MovieDto { Title = movie.Title, Rating = movie.Ratings.Average(), Description = movie.Description, ReleaseDate = movie.ReleaseDate }); }
Once we will change the logic in movieservice class to utilize the extenstion method instead of using the GetMoviesWhereRatingMoreThan method. Test case should start failing for that chage.

[Fact] public void HighRatedMovies_Should_Return_High_Rated_Movies() { // Arrange var highRatedMovies = new List<Movie> { new Movie("The Shawshank Redemption", "Two imprisoned men bond over several years..", new DateTime(1994, 9, 23)), new Movie("The Godfather", "The aging patriarch of an organized crime dynasty...", new DateTime(1972, 3, 24)), new Movie("The Dark Knight", "When the menace known as The Joker emerges...", new DateTime(2008, 7, 18)), new Movie("Pulp Fiction", "The lives of two mob hitmen, a boxer, a gangster's ...", new DateTime(1994, 10, 14)) }; highRatedMovies.ForEach(x => { x.SetRating(4); }); var movieRepositoryMock = new Mock<IMovieRepository>(); movieRepositoryMock.Setup(repo => repo.GetMoviesWhereRatingMoreThan(4)).Returns(highRatedMovies); var movieService = new MovieService(movieRepositoryMock.Object, new Mock<Logger>().Object); // Act var result = movieService.HighRatedMovies().GetAwaiter().GetResult(); // Assert Assert.NotNull(result); Assert.Equal(4, result.Count()); Assert.True(result.All(movie => movie.Rating > 3)); // Check if all movies have a rating more than 4 }
this is the old test case logic where we are trying to setup the GetMoviesWhereRatingMoreThan method which is no more used in the service class. As per our new code we must setup the extension method to pass this test case.
In order to setup the extension method we need to write it inside the wrapper class. So we have implemented the IMovieFilter interface and class to wrap the logic of extension method.
namespace Bt.MoqDemo.Core; public interface IMovieFilter { IEnumerable<Movie> FilterHighRatedMovies(IEnumerable<Movie> movies); } public class MovieFilter { public IEnumerable<Movie> FilterHighRatedMovies(IEnumerable<Movie> movies) { return movies.FilterByRating(8.0); } } public static class MovieExtensions { public static IEnumerable<Movie> FilterByRating(this IEnumerable<Movie> movies, double minRating) { return movies.Where(movie => movie.Ratings.Average() >= minRating); } }
And, modify the test metthod to setup the FilterHighRatedMovies method exposed by the interface.
[Fact] public void HighRatedMovies_Should_Return_High_Rated_Movies() { // Arrange var highRatedMovies = new List<Movie> { new Movie("The Shawshank Redemption", "Two imprisoned men bond over several years..", new DateTime(1994, 9, 23)), new Movie("The Godfather", "The aging patriarch of an organized crime dynasty...", new DateTime(1972, 3, 24)), new Movie("The Dark Knight", "When the menace known as The Joker emerges...", new DateTime(2008, 7, 18)), new Movie("Pulp Fiction", "The lives of two mob hitmen, a boxer, a gangster's ...", new DateTime(1994, 10, 14)) }; highRatedMovies.ForEach(x => { x.SetRating(4); }); var movieRepositoryMock = new Mock<IMovieRepository>(); movieRepositoryMock.Setup(repo => repo.GetAllMovies()).ReturnsAsync(highRatedMovies); var mockFilter = new Mock<IMovieFilter>(); mockFilter.Setup(x => x.FilterHighRatedMovies(It.IsAny<IEnumerable<Movie>>())).Returns(new List<Movie>() { highRatedMovies.FirstOrDefault() }); var movieService = new MovieService(movieRepositoryMock.Object, mockFilter.Object, new Mock<Logger>().Object); // Act var result = movieService.HighRatedMovies().GetAwaiter().GetResult(); // Assert Assert.NotNull(result); Assert.Equal(1, result.Count()); Assert.True(result.All(movie => movie.Rating > 3)); // Check if all movies have a rating more than 4 }

Mocking Test Data
Mocking test data means creating imitation or fake data for testing purposes. This lets you check if your code works as expected without using real, sensitive, or complex information. Mocked test data helps ensure that your code functions correctly and securely during testing without exposing actual data.
Creating and Using Test Data Factories
Creating and using test data factories involves building structures that generate consistent and controlled test data for your software tests. This ensures that you have reliable and standardized data to work with during testing.
Let’s explore this concept with an example using the Movie
class. Now, let’s create a test data factory for the Movie
class using a simple method. This is just one example for demo purpose. However, you can keep the complex data set with lot of varients.
public static class MovieTestDataFactory { public static Movie CreateMovie(string title, string description, DateTime releaseDate, double rating) { return new Movie { Title = title, Description = description, ReleaseDate = releaseDate, Rating = rating }; } }
Let’s utilize the MovieTestDataFactory in the test cases.
public class MovieServiceTests { [Fact] public void AddMovie_Should_Add_Movie_To_Repository() { // Arrange var movieDto = new MovieDto { Title = "Test Movie", Description = "This is a test movie.", ReleaseDate = new DateTime(2023, 7, 1) }; var movie = MovieTestDataFactory.CreateMovie(movieDto.Title, movieDto.Description, movieDto.ReleaseDate, 0); var loggerMock = new Mock<Logger>(); var movieRepositoryMock = new Mock<IMovieRepository>(); movieRepositoryMock.Setup(repo => repo.Add(movie)).Verifiable(); movieRepositoryMock.Setup(repo => repo.SaveChanges()).Verifiable(); var movieService = new MovieService(movieRepositoryMock.Object, loggerMock.Object); // Act var result = movieService.AddMovie(movieDto); // Assert movieRepositoryMock.Verify(); // Verifies that Add and SaveChanges were called Assert.NotNull(result); Assert.NotEqual(Guid.Empty, result.Id); Assert.Equal(0, result.Rating); // Assuming the default rating is 0 } }
In this example, the MovieTestDataFactory
class helps create Movie
instances with consistent data for testing. This improves the clarity and maintainability of your tests while ensuring that you’re working with known and controlled data.
Utilizing Data Builders for Test Data
Utilizing data builders for test data involves creating specialized structures that help construct complex and meaningful test data for your software tests. These data builders streamline the process of creating intricate test scenarios by providing clear and focused methods for setting up data.
let’s create a data builder for the Movie
class to help create test data with more complexity.
public class MovieDataBuilder { private string _title = "Default Title"; private string _description = "Default Description"; private DateTime _releaseDate = DateTime.UtcNow; private double _rating = 0; public MovieDataBuilder WithTitle(string title) { _title = title; return this; } public MovieDataBuilder WithDescription(string description) { _description = description; return this; } public MovieDataBuilder WithReleaseDate(DateTime releaseDate) { _releaseDate = releaseDate; return this; } public MovieDataBuilder WithRating(double rating) { _rating = rating; return this; } public Movie Build() { return new Movie { Title = _title, Description = _description, ReleaseDate = _releaseDate, Rating = _rating }; } }
With this data builder, you can easily create complex and customized test data.
[Fact] public void AddMovie_Should_Add_Movie_To_Repository() { // Arrange var movieDto = new MovieDto { Title = "Test Movie", Description = "This is a test movie.", ReleaseDate = new DateTime(2023, 7, 1) }; var movieBuilder = new MovieDataBuilder() .WithTitle(movieDto.Title) .WithDescription(movieDto.Description) .WithReleaseDate(movieDto.ReleaseDate); var movie = movieBuilder.Build(); var loggerMock = new Mock<Logger>(); var movieRepositoryMock = new Mock<IMovieRepository>(); movieRepositoryMock.Setup(repo => repo.Add(movie)).Verifiable(); movieRepositoryMock.Setup(repo => repo.SaveChanges()).Verifiable(); var movieService = new MovieService(movieRepositoryMock.Object, new Mock<IMovieFilter>().Object, loggerMock.Object); // Act var result = movieService.AddMovie(movieDto); // Assert movieRepositoryMock.Verify(x=>x.Add(It.IsAny<Movie>()),Times.Exactly(1)); movieRepositoryMock.Verify(x => x.SaveChanges(), Times.Exactly(1));// Verifies that Add and SaveChanges were called Assert.NotNull(result); Assert.NotEqual(Guid.Empty, result.Id); Assert.Equal(0, result.Rating); // Assuming the default rating is 0 }
In this example, the MovieDataBuilder
class allows you to construct Movie
instances with specific attributes for testing. This approach enhances test data creation for complex scenarios, making your tests more readable and maintainable while providing the flexibility to customize data as needed.
Mocking Multithreaded Code
Mocking multithreaded code involves creating a controlled environment to test how your software behaves when multiple parts of it run at the same time. Think of it like a simulation where you can see how your code handles situations when different things happen simultaneously.
Let’s use the example of a bank account. Imagine you have a bank account that people can withdraw money from. You want to make sure that if multiple people try to take money out of the account at the same time, your code doesn’t mess up the account balance or cause any problems.
To test this, you can use a technique called mocking. Instead of involving real bank accounts, you create fake or “mock” bank accounts that behave just like the real ones. You then simulate many people trying to take money out of these fake accounts at once.
Let’s use the bank account example to demonstrate how to mock multithreaded code using C# and Xunit. In this example, we’ll simulate multiple withdrawals from a bank account concurrently and ensure that the account balance remains accurate.
using System; using System.Threading.Tasks; using Moq; using NUnit.Framework; public class BankAccount { private decimal _balance; public BankAccount(decimal initialBalance) { _balance = initialBalance; } public decimal Balance => _balance; public void Withdraw(decimal amount) { if (amount > _balance) throw new InvalidOperationException("Insufficient balance."); _balance -= amount; } }
Unit test case to simulate the concurrency behaviour for bank account class.
[TestFixture] public class BankAccountTests { [Test] public void Concurrent_Withdrawals_Should_Not_Cause_Balance_Negative() { // Arrange var initialBalance = 1000; var accountMock = new Mock<BankAccount>(initialBalance); var account = accountMock.Object; // Simulate concurrent withdrawals Parallel.For(0, 100, _ => { account.Withdraw(10); }); // Assert Assert.That(account.Balance >= 0); } }
In this example, we’re using the Moq library to create a mock BankAccount
object. The Concurrent_Withdrawals_Should_Not_Cause_Balance_Negative
test simulates 100 people trying to withdraw $10 from the account concurrently. After the simulation, we check that the account balance is not negative.
Please note that mocking multithreaded code involves some complexities, and this example provides a simplified illustration. In real-world scenarios, you might need to consider synchronization mechanisms like locks or semaphores to handle concurrent access correctly.
Dealing with Circular Dependencies
Circular dependencies occur when two or more modules or classes depend on each other directly or indirectly. These dependencies can make code harder to understand, maintain, and test.
Let’s consider a scenario where we have two classes, User
and Profile
, that exhibit circular dependencies. A User
has a reference to a Profile
, and a Profile
has a reference back to the User
.
public class User { private Profile _profile; public User() { _profile = new Profile(this); } } public class Profile { private User _user; public Profile(User user) { _user = user; } }
This circular dependency between User
and Profile
can make the codebase harder to manage and test. Let’s see how we can break this circular dependency using dependency injection.
Breaking Circular Dependency Using Dependency Injection
public interface IUserService { void CreateUser(); } public class User : IUserService { private readonly IProfileService _profileService; public User(IProfileService profileService) { _profileService = profileService; } public void CreateUser() { // User creation logic _profileService.CreateProfile(this); } } public interface IProfileService { void CreateProfile(User user); } public class Profile : IProfileService { public void CreateProfile(User user) { // Profile creation logic } }
In this example, we’ve introduced interfaces IUserService
and IProfileService
to break the direct circular dependency. The User
class now depends on IProfileService
, and the Profile
class depends on IUserService
. This allows us to inject the required dependencies, breaking the circular relationship.
Best Practices for Effective Mocking
Effective mocking is crucial for writing reliable and maintainable tests. Here are some best practices to ensure you’re using mocking techniques effectively:
- Focus on Behavior, Not Implementation: Mocks should simulate behavior, not replicate implementation details. Define what methods should do and return in different scenarios, rather than replicating their actual code.
- Use Mocking Frameworks: Utilize established mocking frameworks like Moq, Mockito, or Jest, as they provide convenient APIs for creating and configuring mock objects.
- Isolate Dependencies: Mock external dependencies to isolate the unit of code you’re testing. This ensures that test failures are due to the code under test and not external factors.
- Keep Tests Independent: Tests should be independent and not reliant on the order of execution. Mocks should reset between tests to avoid cross-test contamination.
- Avoid Over-Mocking: Only mock what’s necessary for the test. Over-mocking can make tests brittle and obscure the actual behavior being tested.
- Use Verifications Sparingly: While verification is useful, focus on asserting the final outcomes of your code. Too many verifications can lead to testing implementation details.
- Use Real Objects Where Appropriate: Sometimes using real objects or stubs instead of mocks is more appropriate, especially for simple dependencies that don’t affect the outcome of the test.
- Refactor if Mocking Becomes Complicated: If your mocking setup becomes complex, consider refactoring your code to make it more testable and less dependent on external dependencies.
Mocking in Non-.NET Frameworks
Mocking techniques are not limited to the .NET framework; they can be applied in various programming languages and frameworks to create effective tests. While the specifics may vary based on the tools available for each language, the underlying principles remain similar. Here’s how mocking can be done in non-.NET frameworks:
- Java (JUnit and Mockito):
- Use the popular testing framework JUnit for writing unit tests.
- Mockito is a widely used mocking framework for Java. It allows you to create mock objects, set up behaviors, and verify interactions.
- Python (unittest and unittest.mock):
- Python’s built-in
unittest
framework provides a way to write tests. - The
unittest.mock
module (available asunittest.mock
in Python 3.3+ and asmock
for earlier versions) provides mocking capabilities similar to other frameworks.
- Python’s built-in
- JavaScript (Jest and Sinon):
- Jest is a popular testing framework for JavaScript that supports mocking.
- Sinon.js is a powerful mocking library for JavaScript. It provides spies, stubs, and mocks to verify behavior and manage dependencies.
- Ruby (RSpec and RSpec Mocks):
- RSpec is a widely used testing framework for Ruby.
- RSpec Mocks (part of the RSpec ecosystem) offers mocking and stubbing capabilities.
- PHP (PHPUnit and Mockery):
- PHPUnit is a widely used testing framework for PHP.
- Mockery is a PHP mocking framework that provides a convenient way to create mock objects and define expectations.
- Go (testing and testify):
- Go’s built-in
testing
package allows you to write tests. - The
testify
library provides additional testing utilities, including mocking capabilities.
- Go’s built-in
- C++ (Google Test and Google Mock):
- Google Test is a widely used testing framework for C++.
- Google Mock is an extension of Google Test that provides mocking capabilities for C++.
- Swift (XCTest and third-party libraries):
- XCTest is the default testing framework for Swift.
- There are third-party libraries like Mockingbird and Cuckoo that provide mocking capabilities for Swift.
Moq vs. Other Mocking Libraries
Moq, NSubstitute, Rhino Mocks, and other mocking frameworks serve a similar purpose: helping developers create mock objects for testing. However, they have different features, syntax, and philosophies. Here’s a brief comparison:
- Moq:
- Syntax: Moq uses a fluent interface with methods like
Setup
,Returns
, andVerifiable
for defining mock behavior and expectations. - Lambda Expressions: Moq leverages C#’s lambda expressions for specifying mocked method calls.
- Verification: Moq provides verification methods like
Verify
to check if specific methods were called on the mock. - Setup Hierarchies: Moq can set up hierarchies of mock objects to simulate more complex scenarios.
- Strong Community: Moq has a large user base, making it well-documented and frequently updated.
- Syntax: Moq uses a fluent interface with methods like
- NSubstitute:
- Syntax: NSubstitute offers a concise and readable syntax using methods like
When
andReturns
. - Lambdas and Functions: NSubstitute allows you to use both lambdas and functions to define mock behavior.
- No Setup/Verification Separation: NSubstitute combines method setup and verification into a single step.
- Readability: NSubstitute aims for high readability and simplicity in test code.
- Clean Syntax for Complex Behavior: NSubstitute provides clean syntax for complex returns, exceptions, and callback behaviors.
- Syntax: NSubstitute offers a concise and readable syntax using methods like
- Rhino Mocks:
- Dynamic Mocks: Rhino Mocks uses dynamic proxy generation for creating mock objects.
- AAA Syntax: Rhino Mocks follows an “Arrange-Act-Assert” (AAA) syntax for test structure.
- Rigorous Verification: Rhino Mocks supports strict verification, ensuring that all expectations are met.
- Stub and Mock Generation: Rhino Mocks can generate both stubs (simple behavior) and mocks (with expectations).
When choosing a mocking framework, consider factors such as syntax preferences, ease of use, community support, and integration with your preferred testing framework.
Mocking Anti-Patterns and Pitfalls
Mocking is a powerful technique, but it’s important to be aware of certain anti-patterns and pitfalls that can lead to ineffective tests or increased complexity. Here are some common mocking anti-patterns and how to avoid them:
- Over-Mocking:
- Pitfall: Creating mock objects for every dependency, even when unnecessary, can lead to tests that are tightly coupled to implementation details and brittle.
- Solution: Mock only the dependencies that directly impact the behavior you’re testing. Use real objects or lightweight stubs for simple dependencies.
- Testing Implementation Details:
- Pitfall: Writing tests that verify implementation details rather than desired behavior. This makes tests fragile and hard to refactor.
- Solution: Focus on testing behavior and outcomes. Use mocks to verify interactions, not implementation specifics.
- Verifying Internal Methods:
- Pitfall: Overusing verification to check if internal methods are called, leading to tests that are tightly coupled to the implementation structure.
- Solution: Verify interactions with external dependencies, not internal methods. Test public API behavior.
- Complex Mock Setups:
- Pitfall: Creating complex mock setups with long chains of methods can make tests hard to understand and maintain.
- Solution: Keep mock setups simple and focused on the test’s purpose. If setups become complex, consider refactoring your code.
- State Verification Overload:
- Pitfall: Focusing solely on verifying state changes due to method calls can lead to excessive assertions and unclear test intent.
- Solution: Prioritize verifying significant outcomes over every intermediate state change.
Demo Project Link
https://github.com/dkushwah/MoqWithXunit
Conclusion
Moq is a flexible and effective mocking framework that makes it much easier to create mock objects for testing.NET code. Writing efficient and maintainable unit tests is made easier by its fluid syntax, support for lambda expressions, and verification abilities.
With the use of Moq, programmers may isolate individual sections of code, confirm interactions, and exhaustively test a variety of scenarios, ultimately producing more durable and dependable software programmes.
To avoid over-mocking or testing implementation specifics, it’s crucial to utilise Moq sparingly. Focus on testing behaviour and offering insightful information about the functionality of your code.