Dive into the world of xUnit dependency injection and revolutionize your testing approach. With xUnit’s built-in dependency injection framework, you can effortlessly manage and control dependencies in your unit tests, leading to more modular, maintainable, and robust code.
This article delves into the core concepts of xUnit’s dependency injection, guiding you through its integration and showcasing its advantages. By leveraging real-world scenarios and best practices, you’ll uncover how to write cleaner test code, achieve higher code coverage, and facilitate easier debugging. Elevate your testing methodology with the insights gained from harnessing xUnit’s dependency injection capabilities.
In this blog post, we will discuss how xUnit dependency injection capabilities can make the process of writing clean and maintainable unit tests easier.
Dependency injection is a software design pattern that allows objects to be loosely coupled, making them more modular and easier to test. Instead of creating dependencies within the object, dependencies are “injected” from external sources, such as through method parameters or constructor arguments. This decoupling of dependencies promotes flexibility, reusability, and testability.
Just a refresher
Problems Without Dependency Injection
Let’s first try to understand the problems we want to solve if we don’t have a DI available in the testing framework.
Consider a simple example of an EMS application that has a EmployeeService
class responsible for managing employee details. This class has a dependency on a Repository
class for logging and performing the crud operations for employee objects.
Without dependency injection, the
class would need to create an instance of the EmployeeService
the
class within its methodsRepository
public class EmployeeService { private readonly IEmployeeRepository employeeRepository; public EmployeeService() { employeeRepository = new EmployeeRepository(); } public void AddEmployee(Employee employee) { // Perform business logic employeeRepository.Add(employee); } public void UpdateEmployee(Employee employee) { // Perform business logic employeeRepository.Update(employee); } public void DeleteEmployee(int employeeId) { // Perform business logic employeeRepository.Delete(employeeId); } public Employee GetEmployee(int employeeId) { // Perform business logic return employeeRepository.Get(employeeId); } public List<Employee> GetAllEmployees() { // Perform business logic return employeeRepository.GetAll(); } }
This is still manageable; just think about the case in which the EmployeeRepository class once more depends on the EmployeeContext class. then, how our code will look like.
private readonly IEmployeeRepository employeeRepository; public EmployeeService() { var dbContext=new EmployeeDbContext("ConnectionString"); employeeRepository = new EmployeeRepository(dbContext); }
Let’s try to create the XUnit class with the dependency on it similar to how we apply the dependency injection on our normal projects.
namespace Xunit.Demo.Application.Tests { public class EmployeeServiceTests { private readonly EmployeeService _unitUnderTest; public EmployeeServiceTests(IEmployeeRepository employeeRepository) { _unitUnderTest = new EmployeeService(employeeRepository); } [Fact] public void AddEmployee_ShouldNotThrow() { _unitUnderTest.AddEmployee(new Employee() { Id = 1, Age = 34, Name = "John" }); Assert.NotEmpty(_unitUnderTest.GetAllEmployees()); } } }
And, when I tried running the test case. This is what I got as a result.
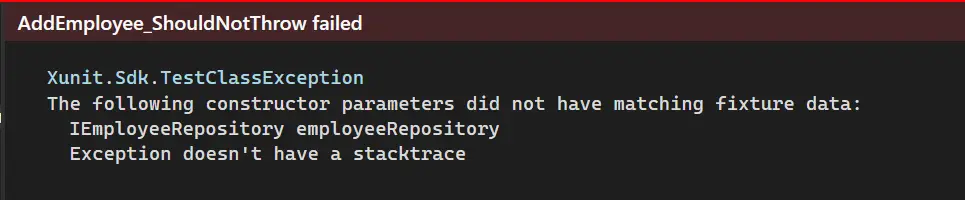
What is text fixture class?
In the context of unit testing, a test fixture is a fixed state or set of objects that is used as a baseline for running multiple tests. A test fixture class is a class that sets up the initial conditions or environment required for testing a specific type.
A test fixture class typically includes the following methods.
- Setup: This is the code that is executed before each test method within the test fixture class. It prepares the necessary resources, initializes objects, and sets up the initial state for the tests.
- Teardown: This is the code that is executed after each test method. It cleans up any resources, resets the environment, or performs any necessary cleanup actions after the test has finished. It helps ensure that each test is isolated and does not affect subsequent tests.
- Shared Objects: A test fixture class may contain shared objects or resources that are used across multiple test methods within the same fixture. These objects can be initialized in the setup method and cleaned up in the teardown method.
Let’s implement the Fixture class for EmployeeServiceTest which we are trying to test.
using Xunit.Demo.Application; namespace Xunit.Demo.Application.Tests { public class EmployeeServiceFixture { public IEmployeeRepository EmployeeRepository; public EmployeeServiceFixture() { EmployeeRepository = new EmployeeRepository(); } } public class EmployeeServiceTests:IClassFixture<EmployeeServiceFixture> { private readonly EmployeeService _unitUnderTest; public EmployeeServiceTests(EmployeeServiceFixture employeeServiceFixture) { _unitUnderTest = new EmployeeService(employeeServiceFixture.EmployeeRepository); } [Fact] public void AddEmployee_ShouldNotThrow() { _unitUnderTest.AddEmployee(new Employee() { Id = 1, Age = 34, Name = "John" }); Assert.NotEmpty(_unitUnderTest.GetAllEmployees()); } } }

DI using IServiceProvider in XUnit
To use IServiceProvider
for dependency injection in Xunit, you can leverage the built-in support provided by the Microsoft.Extensions.DependencyInjection
package. Here’s an example of how you can achieve DI using IServiceProvider
Xunit.
Add NuGet package into the project
<PackageReference Include="Microsoft.Extensions.DependencyInjection" Version="5.0.0" />
Configure your DI container in the test fixture setup method. This involves registering your dependencies and building the IServiceProvider
instance.
using Microsoft.Extensions.DependencyInjection; namespace Xunit.Demo.Application.Tests; public class EmployeeServiceFixture:IDisposable { private readonly IServiceScope _scope; public IEmployeeRepository EmployeeRepository; public EmployeeServiceFixture() { var serviceCollection = new ServiceCollection(); serviceCollection.AddTransient<IEmployeeRepository, EmployeeRepository>(); var serviceProvider = serviceCollection.BuildServiceProvider(); _scope = serviceProvider.CreateScope(); } public void Dispose() { _scope.Dispose(); } public T GetService<T>() { return _scope.ServiceProvider.GetRequiredService<T>(); } }
In this example, EmployeeServiceFixture
the IServiceProvider
by registering the desired dependencies using the ServiceCollection
and building the provider with BuildServiceProvider()
.
The CreateScope()
method is used to create a new scope for each test. The GetService<T>()
method allows you to retrieve the registered service instances from the current scope.
Now, lets jump into the service class which can utilize the fixture along with dependency injections with the help of the GetService method.
using Xunit.Demo.Application; namespace Xunit.Demo.Application.Tests { public class EmployeeServiceTests:IClassFixture<EmployeeServiceFixture> { private EmployeeService uut; public EmployeeServiceTests(EmployeeServiceFixture employeeServiceFixture) { uut = new EmployeeService(employeeServiceFixture.GetService<IEmployeeRepository>()); } [Fact] public void AddEmployee_ShouldNotThrow() { uut.AddEmployee(new Employee() { Id = 1, Age = 34, Name = "John" }); Assert.NotEmpty(uut.GetAllEmployees()); } } }
We could run the test without any issue post implementing the IServiceProvider in the fixture class.
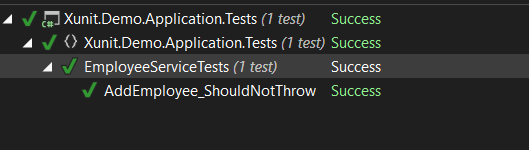
Conclusion
In conclusion, Xunit is a powerful unit-testing framework for .NET applications. While Xunit does not provide built-in support for dependency injection (DI), you can leverage external DI containers, such as IServiceProvider
, to achieve DI in your Xunit tests.
By using a test fixture class, you can set up the necessary resources, dependencies, and initial conditions required for your tests. The test fixture class encapsulates the setup and teardown actions, ensuring a consistent testing environment and reducing code duplication across multiple tests.