Xunit, a popular testing framework for .NET applications, offers an essential feature known as “Expected Exception.” This feature allows developers to precisely define and validate exceptions that their code is expected to throw under certain circumstances.
By harnessing Xunit’s Expected Exception feature, developers can establish more resilient tests that ensure their code handles exceptions appropriately and maintains expected behavior.
In this article, we delve into the ins and outs of Xunit Expected Exception feature, providing clear examples and step-by-step guidance on its implementation. Elevate your unit testing proficiency and bolster the reliability of your .NET projects with this powerful technique.
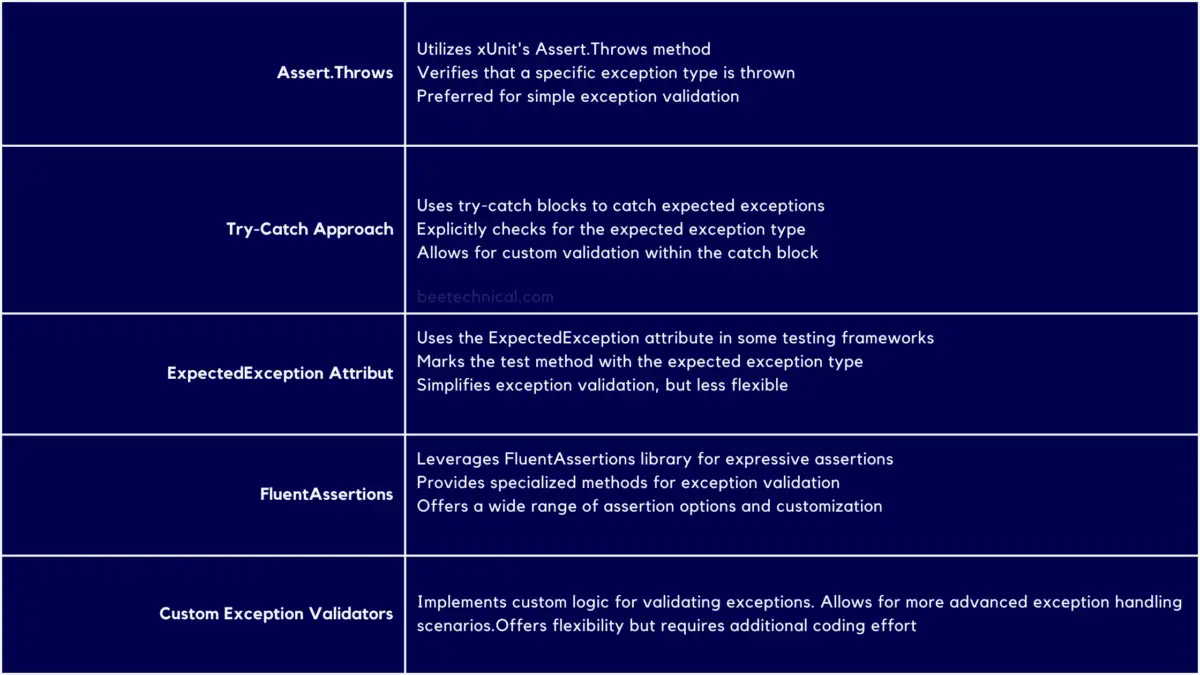
Using Lambda Expression
We can utilize Assert.Throws<TException>
method with a lambda expression as the delegate. Here’s an updated example:
using Xunit; public class MyTestClass { [Fact] public void DivideNumbers_ShouldThrow() { int dividend = 10; int divisor = 0; // Using lambda expression to test the exception Assert.Throws<DivideByZeroException>(() => DivideNumbers(dividend, divisor)); } private int DivideNumbers(int dividend, int divisor) { // Check for divisor being zero if (divisor == 0) { throw new DivideByZeroException("Divisor cannot be zero."); } return dividend / divisor; } }
In above example, we have a test method DivideNumbers_ShouldThrow
that verifies the occurrence of a DivideByZeroException
when attempting to divide dividend
by divisor
.
The Assert.Throws<DivideByZeroException>
method expects an action that throws the specified exception type (DivideByZeroException
in this case).
Using Try-Catch block
We can do the exception test by just placing the assert multiple times using the try catch blocks in the code.
using Xunit; public class MyTestClass { [Theory] [InlineData(10, 0)] [InlineData(1, 0)] public void TestException() { int dividend = 10; int divisor = 0; try { // Perform the division int result = dividend / divisor; // If the exception is not thrown, fail the test Assert.True(false, "Expected DivideByZeroException was not thrown."); } catch (DivideByZeroException) { // Exception was caught, the test passes Assert.True(true); } } }
As we see in this example. We have utilized the theory attribute along with InlineData to pass the information to the test case.
Using Xunit Expected Exception Attribute
[ExpectedException]
attribute is applied to the test method TestException
. The attribute specifies the expected exception type (DivideByZeroException
in this case). When the test is executed, it expects the specified exception to be thrown during the execution of the test method. If the exception is not thrown, the test will fail.
using Xunit; public class MyTestClass { [Fact] [ExpectedException(typeof(DivideByZeroException))] public void TestException() { // Perform the division int result = 10 / 0; } }
Please note that it’s recommended to use newer approaches like Assert.Throws
or FluentAssertions for validating exceptions in xUnit, as the [ExpectedException]
attribute is deprecated and may not be available in the latest versions.
Using Fluent Assertions
FluentAssertions provides a rich set of assertion methods and offers a more expressive syntax for assertions. It allows for additional validation of exception properties, messages, and more.
Remember to include the FluentAssertions NuGet package in your project for using this approach.
dotnet add package FluentAssertions --version 6.11.0
using Xunit; using FluentAssertions; public class MyTestClass { [Fact] public void TestException() { // FluentAssertions approach Action division = () => { int result = 10 / 0; }; division.Should().Throw<DivideByZeroException>(); } }
The Should().Throw<DivideByZeroException>()
method is then invoked on the division
action to assert that it throws a DivideByZeroException
. If the expected exception is not thrown, the test will fail.
Beyond Checking the Exception Type
When validating exceptions in unit tests, it’s often useful to go beyond simple type checks and verify other aspects such as exception messages, inner exceptions, and custom properties. Xunit provides a range of assertion methods that can be combined to achieve thorough exception validation.
using Xunit; public class MyTestClass { [Fact] public void TestCustomException() { try { // Code that may throw a custom exception throw new CustomException("Something went wrong"); } catch (CustomException ex) { // Combining Xunit assertions for exception validation Assert.IsType<CustomException>(ex); Assert.Equal("Something went wrong", ex.Message); Assert.Null(ex.InnerException); Assert.Contains("went wrong", ex.Message); } } }
In this example, we have a test method TestCustomException
that validates a custom exception of type CustomException
. We catch the exception and then proceed to combine multiple Xunit assertions for thorough validation.
Here’s a breakdown of the assertions used:
Assert.IsType<CustomException>(ex)
: Checks that the caught exception is of typeCustomException
.Assert.Equal("Something went wrong", ex.Message)
: Verifies that the exception message matches the expected value.Assert.Null(ex.InnerException)
: Ensures that the caught exception does not have an inner exception.Assert.Contains("went wrong", ex.Message)
: Asserts that the exception message contains the specified substring.
By combining these assertions, we can validate different aspects of the exception, such as its type, message content, absence of inner exception, and specific string patterns within the message.
This approach allows for comprehensive exception validation in unit tests, ensuring that the exceptions being thrown meet the expected criteria and providing detailed feedback when assertions fail.
Remember to tailor the combination of assertions based on the specific needs of your exception validation requirements and adjust the assertions accordingly.
Conclusion
Incorporating Xunit’s Expected Exception feature into your unit testing arsenal is a pivotal step towards creating robust and reliable .NET applications. By gaining a thorough understanding of how to utilize this feature effectively, you empower yourself to catch and address potential issues in your codebase before they manifest in production.