In C#, a “stack” is a collection of items that are stored and retrieved using a last-in, first-out (LIFO) order. In other words, the last item added to the stack is the first one to be removed.
The C# stack data structure is commonly used in programming for a variety of purposes, such as managing function calls, undo/redo operations, and parsing expressions.
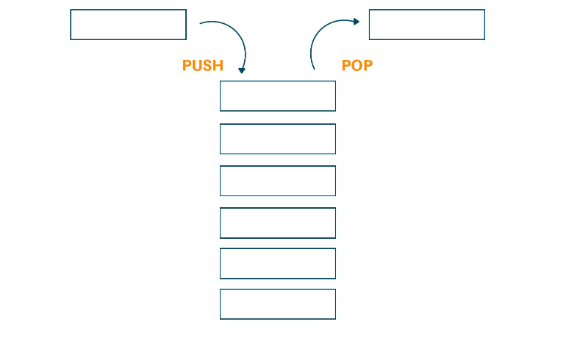
In C# stack is implemented as a class in the System.Collections.Generic namespace, and provides methods for pushing items onto the stack, popping items off the stack, and peeking at the top item without removing it.
When to Use C# Stack
The stack data structure is widely used in computer science and programming and has many applications across different domains. In C#, some common use cases of the stack collection include:
- Function calls: When a function is called, its arguments and local variables are pushed onto the call stack, and popped off when the function returns. This allows functions to be nested and called recursively without running out of memory.
- Undo/redo operations: In an application that supports undo and redo operations, the state of the application at each step can be stored on a stack. When the user performs an undo operation, the most recent state is popped off the stack and restored, while a redo operation pushes the state back onto the stack.
- Expression evaluation: When evaluating expressions in infix notation, the stack can be used to convert them to postfix notation and then evaluate them. Operators and operands are pushed onto the stack as they are encountered, and when an operator is reached, the required number of operands are popped off the stack and the result is pushed back on.
- Browser history: In a web browser, the back and forward buttons can be implemented using a stack to store the URLs of previously visited pages. When the user clicks the back button, the most recent URL is popped off the stack and loaded, while the forward button pushes the URL back onto the stack.
- Algorithmic problems: The stack data structure is a fundamental building block for many algorithmic problems, such as the tower of Hanoi, parenthesis matching, and depth-first search.
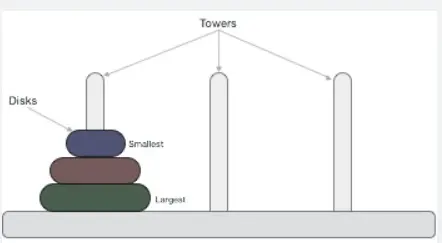
How to Initialize Stack in C#?
In C#, you can initialize a Stack collection by creating a new instance of the Stack class, which is available in the System.Collections.Generic namespace. Here are some examples of how to initialize a Stack in C#.
Using the default constructor: The simplest way to create a Stack is to use the default constructor, which creates an empty stack.
Stack<int> myStack = new Stack<int>();
Using the collection initializer syntax: You can also use the collection initializer syntax to add items to the Stack when you create it:
Stack<string> myStack = new Stack<string>() { "apple", "banana", "cherry" };
Converting an array or list to a Stack: You can convert an array or a list to a Stack using the Stack constructor that takes an IEnumerable parameter.
int[] myArray = { 1, 2, 3, 4, 5 }; Stack<int> myStack = new Stack<int>(myArray);
Using the Push method: You can add items to a Stack at runtime using the Push method.
Stack<double> myStack = new Stack<double>(); myStack.Push(3.14); myStack.Push(2.71);
Available Methods in C# Stack
In C#, the Stack class provides several operations that you can perform on a Stack collection:
Push: The Push method adds an item to the top of the Stack.
Stack<int> myStack = new Stack<int>(); myStack.Push(42);
Pop: The Pop method removes and returns the top item from the Stack.
Stack<int> myStack = new Stack<int>(); myStack.Push(42); int topItem = myStack.Pop();
Peek: The Peek method returns the top item from the Stack without removing it.
Stack<int> myStack = new Stack<int>(); myStack.Push(42); int topItem = myStack.Peek();
Count: The Count property returns the number of items in the Stack:
Stack<int> myStack = new Stack<int>(); myStack.Push(42); myStack.Push(23); int count = myStack.Count; // count = 2
Clear: The Clear method removes all items from the Stack.
Stack<int> myStack = new Stack<int>(); myStack.Push(42); myStack.Clear();
Contains: The Contains method returns true if the Stack contains a specified item, and false otherwise.
Stack<int> myStack = new Stack<int>(); myStack.Push(42); bool containsItem = myStack.Contains(42); // containsItem = true
CopyTo: The CopyTo method copies the items in the Stack to an array.
Stack<int> myStack = new Stack<int>(); myStack.Push(42); myStack.Push(23); int[] myArray = new int[myStack.Count]; myStack.CopyTo(myArray, 0); // myArray = { 23, 42 }
By using these operations on a Stack in C#, you can add, remove, access, and manipulate items in a LIFO order, which can be useful in a wide range of programming scenarios.
How to iterate stack in C#?
In C#, you can iterate over the items in a Stack collection using a foreach loop or by converting the Stack to an array and then iterating over the array. Here’s how to do it.
Using a foreach loop: The foreach loop allows you to iterate over the items in a Stack in LIFO order, starting from the top.
Stack<string> myStack = new Stack<string>(); myStack.Push("apple"); myStack.Push("banana"); myStack.Push("cherry"); foreach (string item in myStack) { Console.WriteLine(item); } // Output: "cherry", "banana", "apple"
Using an array: The ToArray method converts the Stack to an array, which can then be iterated over using a for loop or another type of loop.
Stack<string> myStack = new Stack<string>(); myStack.Push("apple"); myStack.Push("banana"); myStack.Push("cherry"); string[] myArray = myStack.ToArray(); for (int i = 0; i < myArray.Length; i++) { Console.WriteLine(myArray[i]); } // Output: "cherry", "banana", "apple"
Available Properties in Stack Class in C#
In C#, the Stack class is defined in the System.Collections.Generic namespace and provides an implementation of the last-in-first-out (LIFO) data structure.
- Count: Gets the number of elements contained in the Stack.
- IsSynchronized: Gets a value indicating whether access to the Stack is synchronized (thread-safe).
- SyncRoot: Gets an object that can be used to synchronize access to the Stack.
- Capacity: Gets or sets the maximum number of elements that the Stack can contain.
Here is an example of how to use these properties in C#:
using System; using System.Collections.Generic; class Program { static void Main(string[] args) { Stack<int> stack = new Stack<int>(); // Add some elements to the stack stack.Push(1); stack.Push(2); stack.Push(3); // Use the Count property to get the number of elements Console.WriteLine("Number of elements in stack: " + stack.Count); // Use the Capacity property to get or set the maximum number of elements Console.WriteLine("Capacity of stack: " + stack.Capacity); // Use the IsSynchronized property to check if the stack is thread-safe Console.WriteLine("Is stack thread-safe? " + stack.IsSynchronized); // Use the SyncRoot property to get an object for synchronizing access object syncRoot = stack.SyncRoot; } }
Conclusion
In this article, we have tried to understand the stack class in detail. What it is, when to use it, and how to use it. Also, we tried to understand the different methods and properties available in the C# stack.
FAQ
When to use Stack vs Queue?
A stack operates on a Last-In-First-Out (LIFO) principle, meaning that the last item added to the stack is the first item to be removed. This makes it ideal for scenarios where items need to be added and removed in a specific order, such as undo/redo functionality or recursive function calls.
A queue, on the other hand, operates on a First-In-First-Out (FIFO) principle, meaning that the first item added to the queue is the first item to be removed. This makes it ideal for scenarios where items need to be processed in the order in which they are received, such as processing requests in a web server.
Is the Stack thread safe?
The Stack class in C# is not thread-safe by default. This means that if multiple threads attempt to modify a Stack instance concurrently, it can lead to race conditions and unexpected behavior.
What is the default capacity of the Stack object?
The default capacity of a Stack object in C# is 10. This means that the Stack object can hold up to 10 items before it needs to be resized. However, the capacity of a Stack object can be increased or decreased as needed using the SetCapacity method or by setting the Capacity property directly.
Comments are closed.