In C# Queue is a collection that is used to hold a group of elements, allowing elements to be added to the end of the collection and removed from the front. It follows the First-In-First-Out (FIFO) principle, meaning that the first element added to the queue is the first to be removed.

The Queue class is part of the System.Collections.Generic namespace provides a variety of methods to manipulate the elements in the collection, including Enqueue, Dequeue, Peek, Contains, and Count. These methods allow you to add elements to the end of the queue, remove elements from the front of the queue, retrieve the element at the front of the queue without removing it, check if an element is in the queue, and get the number of elements in the queue.
Characteristics of Queue<T> Class in C#
The Queue collection in C# programming has the following characteristics:
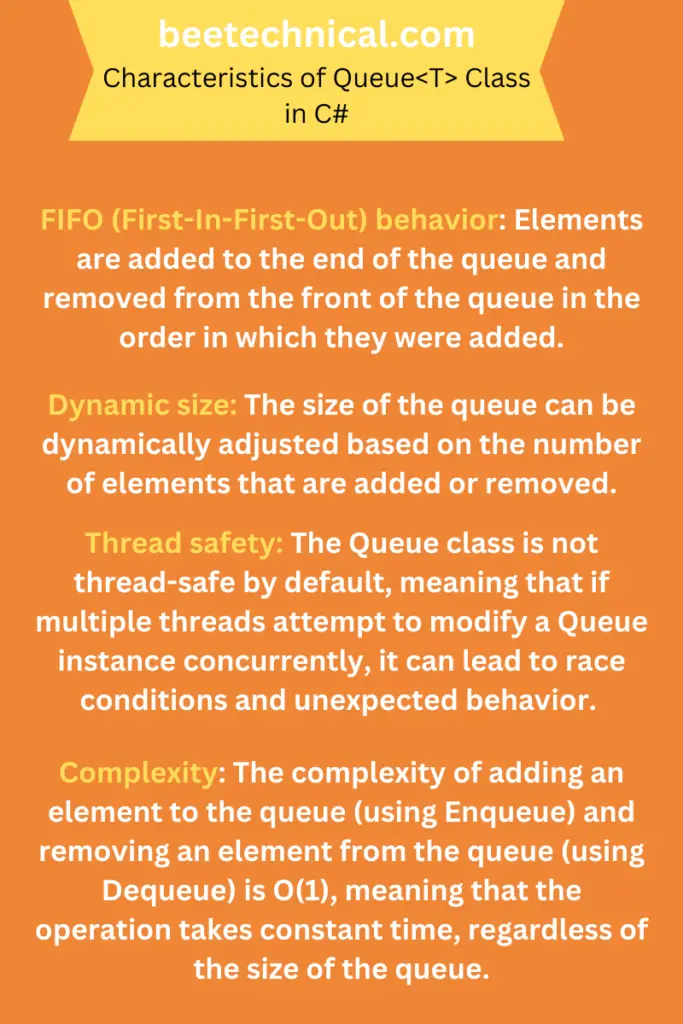
- FIFO (First-In-First-Out) behavior: Elements are added to the end of the queue and removed from the front of the queue in the order in which they were added.
- Dynamic size: The size of the queue can be dynamically adjusted based on the number of elements that are added or removed.
- Element access: The element at the front of the queue can be accessed using the Peek method, without removing the element from the queue. Elements can also be removed from the front of the queue using the Dequeue method.
- Thread safety: The Queue class is not thread-safe by default, meaning that if multiple threads attempt to modify a Queue instance concurrently, it can lead to race conditions and unexpected behavior. To ensure thread safety, you can use synchronization techniques such as locking or the ConcurrentQueue class.
- Complexity: The complexity of adding an element to the queue (using Enqueue) and removing an element from the queue (using Dequeue) is O(1), meaning that the operation takes constant time, regardless of the size of the queue.
When to use Queue<T> Collection?
Queues have several use cases in computer programming and computer systems. Here are some common use cases for queues:
- Task processing: Queues can be used to manage tasks or jobs that need to be processed in a specific order, such as in a batch processing system. The queue ensures that the tasks are processed in the order they were received.
- Event handling: In an event-driven system, events can be queued up for processing. The queue ensures that events are processed in the order they were received, which can be important in certain scenarios.
- Messaging systems: Queues can be used to manage message passing between different parts of a distributed system, ensuring that messages are processed in the correct order.
- Resource allocation: In resource-constrained systems, queues can be used to manage resource allocation. For example, if there are multiple processes competing for a single resource, a queue can be used to ensure that each process gets access to the resource in a fair and orderly manner.
- Buffering: Queues can be used to buffer input and output data in a system, allowing the system to handle bursts of data without overwhelming it.
Overall, queues are a versatile data structure that can be used in a wide range of scenarios where elements need to be processed in a specific order or where there is competition for limited resources.
How to Add and Remove Items in the Queue<T>?
To add an item to the end of a Queue in C#, use the Enqueue method, and to remove an item from the front of the Queue, use the Dequeue method.
Enqueue Items
To add items to a Queue in C#, you can use the Enqueue method, which adds an element to the end of the queue. The Enqueue method has the following syntax:
myQueue.Enqueue(item);
Here, myQueue
is the name of your Queue instance, and item
is the item you want to add to the queue.
Queue<int> myQueue = new Queue<int>(); myQueue.Enqueue(10); myQueue.Enqueue(20); myQueue.Enqueue(30);
In this example, we create a Queue of integers and then use the Enqueue method to add three elements to the queue.
Dequeue Items
To remove items from a Queue in C#, you can use the Dequeue method, which removes and returns the element at the front of the queue. The Dequeue method has the following syntax:
var firstItem = myQueue.Dequeue();
Here, myQueue
is the name of your Queue instance, and firstItem
is a variable that will hold the element at the front of the queue.
Queue<int> myQueue = new Queue<int>(); myQueue.Enqueue(10); myQueue.Enqueue(20); myQueue.Enqueue(30); int firstItem = myQueue.Dequeue();
In this example, we create a Queue of integers, add three elements to the queue using the Enqueue method, and then remove the first element from the queue using the Dequeue method. The value of firstItem
will be 10
, since that was the first element added to the queue.
How to iterate through Queue<T> in C#?
you can iterate over a Queue using the foreach
loop or a while
loop.
Queue<string> myQueue = new Queue<string>(); // Add items to the queue myQueue.Enqueue("First"); myQueue.Enqueue("Second"); myQueue.Enqueue("Third"); // Using a foreach loop foreach (string item in myQueue) { Console.WriteLine(item); } // Using a while loop while (myQueue.Count > 0) { string item = myQueue.Dequeue(); Console.WriteLine(item); }
In the example above, we create a Queue of strings called myQueue
and add three items to it. We then use a foreach
loop to iterate over the items in the queue and print them to the console. Alternatively, we can use a while
loop to remove items from the queue using the Dequeue()
method until the queue is empty, and print each item to the console as we remove it.
Queue vs ConcurrentQueue in C#
Queue
and ConcurrentQueue
are two different classes that can be used to implement a first-in, first-out (FIFO) collection of items. Queue
is a non-thread-safe collection, meaning that it’s designed to be used in a single-threaded application. If you try to use a Queue
from multiple threads concurrently, you can run into issues such as race conditions and data corruption.
On the other hand, ConcurrentQueue
is a thread-safe collection that can be used in a multi-threaded environment. It provides atomic operations to add and remove items from the queue, so that multiple threads can access the queue simultaneously without causing issues.
Here’s an example of how to use Queue
:
Queue<string> myQueue = new Queue<string>(); // Add items to the queue myQueue.Enqueue("First"); myQueue.Enqueue("Second"); myQueue.Enqueue("Third"); // Remove items from the queue while (myQueue.Count > 0) { string item = myQueue.Dequeue(); Console.WriteLine(item); }
And here’s an example of how to use ConcurrentQueue
:
ConcurrentQueue<string> myQueue = new ConcurrentQueue<string>(); // Add items to the queue myQueue.Enqueue("First"); myQueue.Enqueue("Second"); myQueue.Enqueue("Third"); // Remove items from the queue string item; while (myQueue.TryDequeue(out item)) { Console.WriteLine(item); }
How to check if the Queue is full?
In C#, the Queue<T>
class does not have a fixed capacity, so it does not have a concept of being “full”. You can add as many items to the queue as you have memory available.
If you need to limit the number of items in the queue, you can create a new class that encapsulates a Queue<T>
and tracks the maximum capacity. Here’s an example:
public class FixedSizeQueue<T> { private Queue<T> queue; private int maxSize; public FixedSizeQueue(int maxSize) { this.queue = new Queue<T>(); this.maxSize = maxSize; } public void Enqueue(T item) { if (queue.Count >= maxSize) { throw new InvalidOperationException("Queue is full"); } queue.Enqueue(item); } // Other methods like Dequeue, Peek, Count, etc. }
In this example, we create a new class called FixedSizeQueue
that encapsulates a Queue<T>
and a maximum size. The Enqueue
method checks whether the queue is already full before adding an item, and throws an exception if it is. If the queue is not full, the item is added to the queue using the Enqueue
method of the Queue<T>
class. You can add other methods like Dequeue
, Peek
, Count
, etc. to this class as needed.
Conclusion
Queue<T>
is a useful collection in C# for managing a sequence of elements in a first-in, first-out order. It’s simple and easy to use, making it a popular choice for many developers. However, it’s important to keep in mind that Queue<T>
is not thread-safe and should not be used in a multi-threaded environment without proper synchronization.
Comments are closed.