C# ArrayList class is a dynamic array that allows you to store and manipulate a collection of objects of any type. It provides methods to add, remove, and access elements in the array, and it can grow or shrink dynamically as needed.
The ArrayList class is part of the System.Collections namespace and is commonly used in C# programming for tasks such as storing and manipulating large amounts of data or working with variable-sized data structures.
How to Create C# ArrayList?
There are a few ways to create an ArrayList in C#. The best approach depends on the specific needs of your code and the context in which you are working. Note that since ArrayList is a legacy collection class in C#, it is recommended to use more modern collections like List<T> whenever possible.
Using the ArrayList constructor
In this example, we use the ArrayList
constructor to create an empty ArrayList (list1
), an ArrayList with an initial capacity of 10 (list2
), and an ArrayList with initial values (list3
). The third constructor takes an array of values that are added to the ArrayList.
ArrayList list1 = new ArrayList(); // create an empty ArrayList ArrayList list2 = new ArrayList(10); // create an ArrayList with an initial capacity of 10 ArrayList list3 = new ArrayList(new int[] { 1, 2, 3 }); // create an ArrayList with initial values
Using the Add method to add elements to an existing ArrayList
In this example, we create an empty ArrayList and then use the Add
method to add three elements to it: a string, an integer, and a DateTime
object.
ArrayList list = new ArrayList(); list.Add("hello"); list.Add(42); list.Add(DateTime.Now);
Using object and collection initializers
In this example, we use object and collection initializers to create an ArrayList with three initial elements: a string, an integer, and an DateTime
object. This syntax is similar to creating an object using the new
keyword but with the added convenience of initializing properties or adding elements to a collection at the same time.
ArrayList list = new ArrayList { "hello", 42, DateTime.Now };
How to Access Array Element in C#?
There are multiple ways to access elements of an ArrayList in C#. Let’s understand each of them with example provided below.
Using a loop to iterate over all the elements of the array
In this example, we use the Count
property of the ArrayList to determine the number of elements, and then iterate over the ArrayList using a for
loop. Inside the loop, we use the indexer to access individual elements.
ArrayList list = new ArrayList { "hello", 42, DateTime.Now }; for (int i = 0; i < list.Count; i++) { Console.WriteLine(list[i]); }
Using the foreach
loop to iterate over all the elements of the array
In this example, we use a foreach
loop to iterate over the ArrayList and access each element in turn. Since ArrayList is a collection of objects, we use the object
type for the loop variable.
ArrayList list = new ArrayList { "hello", 42, DateTime.Now }; foreach (object item in list) { Console.WriteLine(item); }
Using LINQ to query
In this example, we start by creating an ArrayList with three elements: a string, an integer, and a DateTime
object. We then use the OfType
extension method from LINQ to filter the ArrayList and get only the integer elements. Finally, we use the FirstOrDefault
method to get the first integer element, or return the default value (0) if there are no integer elements in the ArrayList.
// create an ArrayList with some elements ArrayList list = new ArrayList { "hello", 42, DateTime.Now }; // use LINQ to get the first integer element from the ArrayList int firstInt = list.OfType<int>().FirstOrDefault(); Console.WriteLine(firstInt); // output: 42
Multiple ways to remove the elements from the ArrayList
There are multiple ways to remove an element from an ArrayList in C#.
One way is to use the Remove
method, which removes the first occurrence of an element in the ArrayList. This method takes the value of the element as its parameter and removes the first element that matches that value.
ArrayList list = new ArrayList { "hello", 42, DateTime.Now }; list.Remove(42); // remove the integer element
Another way is to use the RemoveAt
method, which removes an element from the ArrayList at a specific index. This method takes the index of the element to be removed as its parameter.
ArrayList list = new ArrayList { "hello", 42, DateTime.Now }; list.RemoveAt(1); // remove the element at index 1 (which is the integer)
A third way to remove an element from an ArrayList is to use the RemoveAll
method with a lambda expression that defines the condition for removing elements. This method removes all elements that match the condition defined by the lambda expression.
ArrayList list = new ArrayList { "hello", 42, DateTime.Now, 42 }; list.RemoveAll(item => item.Equals(42)); // remove all occurrences of the integer
Finally, it is possible to remove elements from an ArrayList using a for
loop. In this approach, we iterate over the ArrayList and remove elements that match a certain condition. One important thing to note when using this approach is that we must iterate over the ArrayList in reverse order to avoid issues with the indices of the remaining elements.
ArrayList list = new ArrayList { "hello", 42, DateTime.Now, 42 }; for (int i = list.Count - 1; i >= 0; i--) { if (list[i].Equals(42)) { list.RemoveAt(i); } }
Commonly Used ArrayList Methods
Here are some of the commonly used methods of the ArrayList class in C#:
- Add(object value): Adds an object to the end of the ArrayList.
- AddRange(ICollection c): Adds the elements of a collection to the end of the ArrayList.
- Clear(): Removes all elements from the ArrayList.
- Contains(object item): Determines whether the ArrayList contains a specific element.
- IndexOf(object value): Searches for the specified object and returns the index of its first occurrence in the ArrayList.
- Insert(int index, object value): Inserts an element into the ArrayList at the specified index.
- InsertRange(int index, ICollection c): Inserts the elements of a collection into the ArrayList at the specified index.
- Remove(object obj): Removes the first occurrence of a specific object from the ArrayList.
- RemoveAt(int index): Removes the element at the specified index of the ArrayList.
- RemoveRange(int index, int count): Removes a range of elements from the ArrayList.
- ToArray(): Copies the elements of the ArrayList to a new object array.
- TrimToSize(): Sets the capacity of the ArrayList to the actual number of elements it contains.
These are just some of the methods available in the ArrayList class. Depending on your use case, you may find other methods that are more appropriate for your needs.
Commonly Used ArrayList Properties
Here are some of the commonly used properties of the ArrayList
class in C#:
- Capacity: Gets or sets the number of elements that the ArrayList can contain.
- Count: Gets the number of elements currently contained in the ArrayList.
- IsFixedSize: Gets a value indicating whether the ArrayList has a fixed size.
- IsReadOnly: Gets a value indicating whether the ArrayList is read-only.
- IsSynchronized: Gets a value indicating whether access to the ArrayList is synchronized (thread-safe).
- Item[int index]: Gets or sets the element at the specified index.
- SyncRoot: Gets an object that can be used to synchronize access to the ArrayList.
These properties provide various information about the ArrayList
object and allow us to manage its capacity, size, and thread-safety. For instance, we can use the Capacity
property to increase or decrease the number of elements that the ArrayList can contain. Similarly, we can use the Count
property to get the number of elements currently stored in the ArrayList. The IsReadOnly
property tells us whether the ArrayList is read-only, while the IsSynchronized
property tells us whether it is thread-safe. Finally, we can use the Item
property to get or set the value of an element at a specific index in the ArrayList.
Diffrence between Array and ArrayList
In C#, both arrays and ArrayLists are used to store a collection of elements, but they differ in several ways.
Array | ArrayList |
A fixed-size collection of elements of the same type. | A dynamic collection of objects that can grow or shrink in size. |
Memory allocation for the array is done at compile-time and is static. | Memory allocation for ArrayList is done at runtime and is dynamic. |
Array elements can be accessed directly using an index. | Elements in the ArrayList are accessed through an enumerator, which is slower than direct indexing. |
Length property is used to get the number of elements in the array. | Count property is used to get the number of elements in the ArrayList. |
Arrays can be multidimensional, with more than one dimension. | ArrayLists cannot be multidimensional. |
In summary, arrays are more efficient when working with a fixed-size collection of elements of the same type, while ArrayLists are more flexible for storing a dynamic collection of objects of different types. Arrays are also faster when it comes to direct indexing, while ArrayLists are slower due to their use of enumerators.
ArrayList arrayList = new ArrayList(); arrayList.Add(1); arrayList.Add(2); arrayList.Add(3); IEnumerable<int> filteredElements = arrayList.OfType<int>().Where(i => i > 1); foreach (int element in filteredElements) { Console.WriteLine(element); }
What is the difference between list and arraylist in c#?
In C#, a List and an ArrayList are both collections that can store and manipulate objects. However, there are some differences between them:
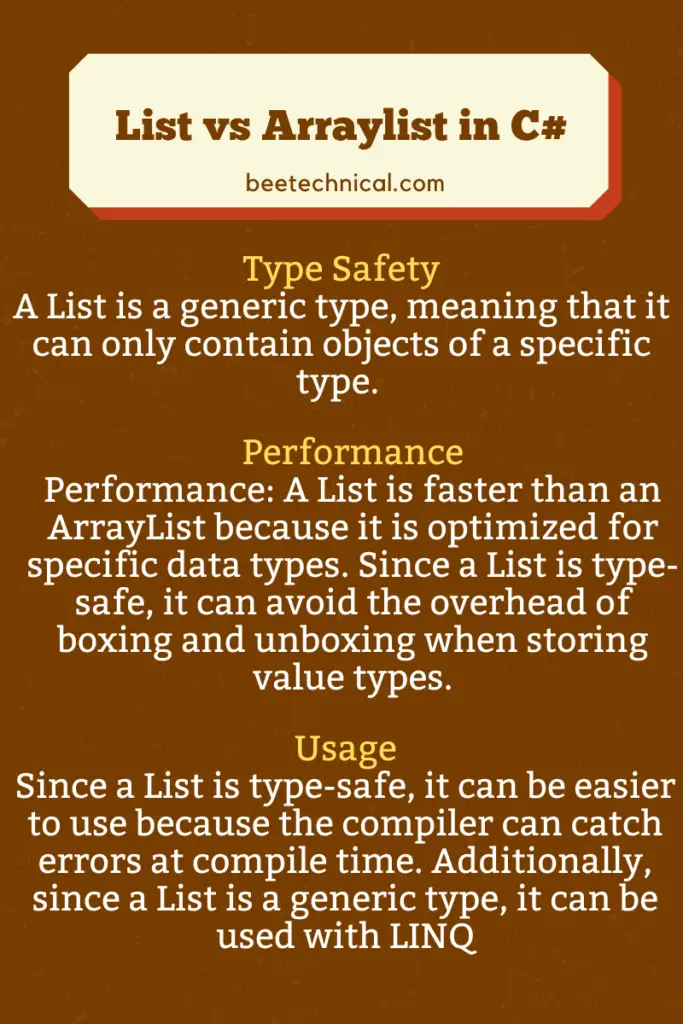
Type Safety: A List is a generic type, meaning that it can only contain objects of a specific type. For example, a List<int>
can only hold integer values. In contrast, an ArrayList is not type-safe and can store objects of any type.
- Performance: A List is faster than an ArrayList because it is optimized for specific data types. Since a List is type-safe, it can avoid the overhead of boxing and unboxing when storing value types. On the other hand, an ArrayList stores object as objects, which means that it has to box value types and unbox reference types, which can be slower.
- Usage: Since a List is type-safe, it can be easier to use because the compiler can catch errors at compile time. Additionally, since a List is a generic type, it can be used with LINQ, which provides a powerful set of features for querying and manipulating collections. In contrast, an ArrayList can be used when the type of the objects to be stored is unknown or when you want to store objects of different types in the same collection.
Conclusion
In conclusion, ArrayList in C# is a collection class that can store objects of any type. It provides dynamic resizing and a variety of methods for adding, removing, and manipulating elements. However, it is not type-safe, which means that it has to box value types and unbox reference types, which can result in slower performance compared to the type-safe List collection. As a result, if you know the type of the objects you want to store, it is recommended to use List instead of ArrayList for better performance and type safety.
Can we use linq on arraylist in c#
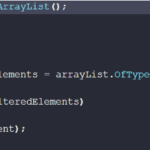
Yes, you can use LINQ (Language-Integrated Query) with an ArrayList in C# since ArrayList implements the IEnumerable
and ICollection
interfaces, which allow you to use LINQ extension methods on it.
Why arraylist is not thread safe?
ArrayList in C# is not thread-safe because it does not provide any built-in mechanism to synchronize access to its internal data structures. This means that if multiple threads access an ArrayList instance concurrently, there is a risk of data corruption or inconsistent state. For example, if one thread tries to modify the ArrayList while another thread is iterating over it, it may result in an exception being thrown or incorrect data being returned.
To avoid these issues, you can use synchronization mechanisms such as locking or the System.Collections.Concurrent classes (e.g. ConcurrentBag, ConcurrentQueue, ConcurrentStack) which are designed for multi-threaded access. Alternatively, you can use the generic List<T> class which is a type-safe collection and provides better performance and thread safety than ArrayList.
It’s important to note that not all collections in C# are thread-safe by default. It’s the responsibility of the developer to ensure that collections are accessed in a thread-safe manner if they are used in a multi-threaded context.
Comments are closed.