When working with C#, selecting the appropriate data type is crucial for designing efficient and maintainable code. This article explores the three primary data types in C#: records
, classes
, and structs
, and provides real-world examples to illustrate their best use cases. By understanding the characteristics and intended purposes of each, developers can make informed decisions when defining custom data types.
Records store a fixed set of named values and are often immutable. Classes are blueprints for creating objects with data (attributes) and behavior (methods). On the other hand, structs group together different data items under a single name and are usually used in languages that focus on low-level memory manipulation, being copied by value when passed around.
Records
Records, introduced in C# 9.0, are ideal for modeling immutable data. Consider a “Person” record representing a person’s name and age. With built-in support for immutability and value-based equality.
Let’s understand with an example.
public record Employee(1, "John Doe", "Engineering");
By default, records generate useful methods like Equals
, GetHashCode
, and ToString
based on their properties.
This eliminates the need for manual implementation, enhancing code maintainability. Records are often used for data transfer objects (DTOs), messages, or simple data containers where immutability and equality are critical.
Now, let’s create two instances of the person record and compare them:
var employee1 = new Employee(1, "John Doe", "Engineering"); var employee2 = new Employee(1, "John Doe", "Engineering"); bool areEqual = employee1.Equals(employee2); Console.WriteLine($"Are employee1 and employee2 equal? {areEqual}");
You can refer to the old article where we tried to understand the difference between ValueObject Vs Entity Class, and we had to implement the custom methods to compare all the properties.
When to Use Record
- Immutable Data Transfer Objects (DTOs):
- When transferring data between layers or systems, records can be used to represent immutable DTOs. These DTOs encapsulate data without exposing mutation methods, ensuring data integrity during transportation.
- Message Passing:
- Records are well-suited for representing messages in event-driven or messaging systems. They provide a concise and expressive way to define message types, facilitating communication between components.
- Caching and Memoization:
- Records can be used to represent cache keys or memoization results. Their immutability guarantees consistency, and the built-in value-based equality simplifies cache lookups or memoization checks.
- Event Sourcing and Event Log Entries:
- In event-sourcing architectures, records can represent events or event log entries. The immutability ensures that historical data remains unchanged, and value-based equality enables efficient event deduplication.
- Data Analysis and Aggregations:
- Records can be used to model data for analysis or aggregations. For instance, in financial applications, records can represent transactions, stock prices, or other data points used for calculations.
- API Response Objects:
- When designing APIs, records can be utilized to represent response objects returned from API endpoints. The built-in value-based equality simplifies comparison and allows for straightforward testing.
- Configuration and Settings:
- Records can be employed to represent configuration objects or application settings. Their immutability guarantees consistency, and the auto-generated
ToString
the method provides readable representations for logging or debugging.
- Records can be employed to represent configuration objects or application settings. Their immutability guarantees consistency, and the auto-generated
- Data Validation and Data Transformation:
- Records can be used for data validation or transformation operations. For example, a record can represent a validated input or serve as an intermediate step in a data transformation pipeline.
When to Use Structs
Structs are value types that are used when memory efficiency and value semantics are critical.
Structs are allocated on the stack or embedded within containing objects, resulting in efficient memory usage. They are copied by value, ensuring that modifications to one instance do not affect others.
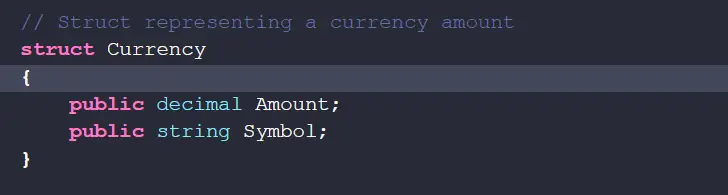
Structs are commonly used for lightweight data structures, numerical types, and scenarios where performance is paramount.
When to Use structs
- Lightweight Data Structures:
- Point or Vector structures: Used to represent two-dimensional or three-dimensional coordinates in graphics or geometric calculations.
- Complex numbers: Structs can be utilized to define a complex number data type with real and imaginary parts for mathematical computations.
- Performance-Critical Scenarios:
- Numerical types: Structs can be employed to create lightweight numerical types (e.g., Matrix, Quaternion) for efficient calculations in scientific or mathematical applications.
- Game development: Structs are commonly utilized to represent game entities, positions, velocities, or transformations due to their performance advantages.
- Small, Self-Contained Data:
- Date and time structures: Structs like DateTime or TimeSpan can be used to handle date and time-related operations efficiently.
- Currency or monetary values: Structs can be used to define a Money structure to represent and handle currency amounts with precision.
- Interoperability:
- Platform Invoke (P/Invoke): When working with unmanaged code or APIs, structs can be used to represent data structures required for interoperability.
- Custom Data Types with Value Semantics:
- Immutable data: Structs can be used to create immutable data types for scenarios where data should not be modified after initialization.
- Configuration objects: Structs can be employed to define lightweight configuration objects with value semantics, avoiding unintended modifications.
- Embedded Systems:
- In low-level programming or embedded systems, structs are often utilized to represent hardware registers or data packets due to their memory efficiency.
Example Program for Struct in C#
Classes
Classes are suitable for modeling complex objects that require behavior. They allow for defining methods, properties, events, and other members to encapsulate functionality. Classes are commonly used for modeling real-world entities, implementing business logic, and creating reusable components.
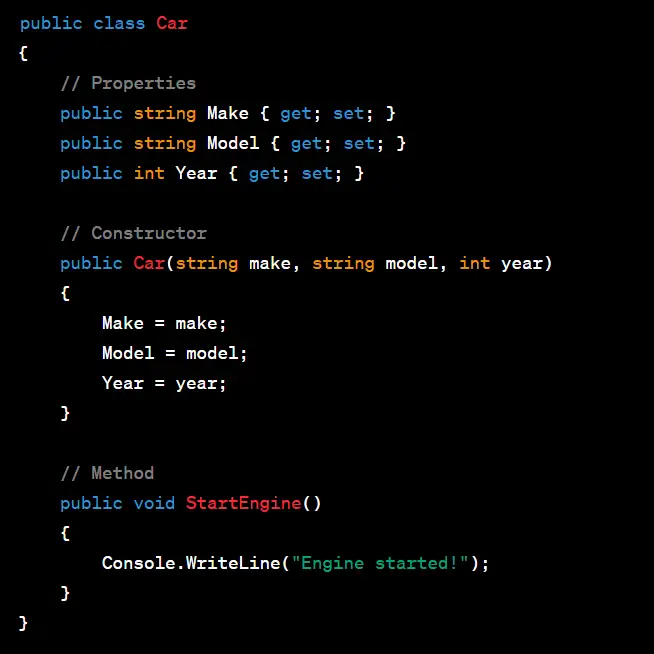
Classes in C# are versatile and widely used for various purposes. Here are some real-time use cases where classes excel.
use cases for Class
- Object-Oriented Modeling: Classes are fundamental to object-oriented programming and are used to model real-world entities. For example:
- A “Customer” class that represents customer information, such as name, address, and contact details.
- A “BankAccount” class that encapsulates account properties and behaviors, such as balance, deposits, and withdrawals.
- Business Logic and Processing:
- A “PaymentProcessor” class that handles payment transactions, verifies payment details and interacts with payment gateways.
- A “StockAnalyzer” class that analyzes stock market data, calculates performance metrics, and generates reports.
- GUI Components and Event Handling:
- A “Window” class that represents a graphical window in a desktop application, with properties and methods for layout, rendering, and event handling.
- A “Button” class that encapsulates the behavior of a button control, including events like clicking and styling options.
- Data Access and Persistence:
- A “DataAccessLayer” class that provides methods to interact with a database, including CRUD (Create, Read, Update, Delete) operations.
- An “EntityMapper” class that maps database records to corresponding entity objects, handling data transformation and abstraction.
- Service and API Integration:
- An “EmailService” class that sends emails, handles email templates and interacts with external email service providers.
- A “WeatherApiService” class that retrieves weather data from a third-party API, processes the response and exposes methods for accessing weather information.
- Utility and Helper Classes:
- A “StringUtils” class that provides various string manipulation methods, such as formatting, parsing, or validating strings.
- A “DateTimeUtils” class that offers date and time-related operations, including conversions, calculations, and formatting.
Classes are highly flexible and adaptable, allowing developers to encapsulate data and behavior into reusable components. They are extensively used in software development to organize code, create modular designs, and represent complex systems and interactions.
Example Program for Class in C#
Conclusion
By considering the characteristics and intended use cases of records, classes, and structs, developers can choose the most appropriate data type, leading to cleaner, more efficient, and maintainable code. Each data type has its own benefits and trade-offs, and understanding when to use each one helps optimize code design and improve overall software development practices.
Comments are closed.