Welcome to this interactive guide on using C# Set collections. In this article, we will dive into the world of sets, which are powerful data structures that allow you to store and manipulate unique elements efficiently.
In C#, a set collection stands as a collection type where every item is one-of-a-kind, leaving no room for duplicates. This exclusive feature is brought to you by the HashSet class, shaping the essence of the set collection.
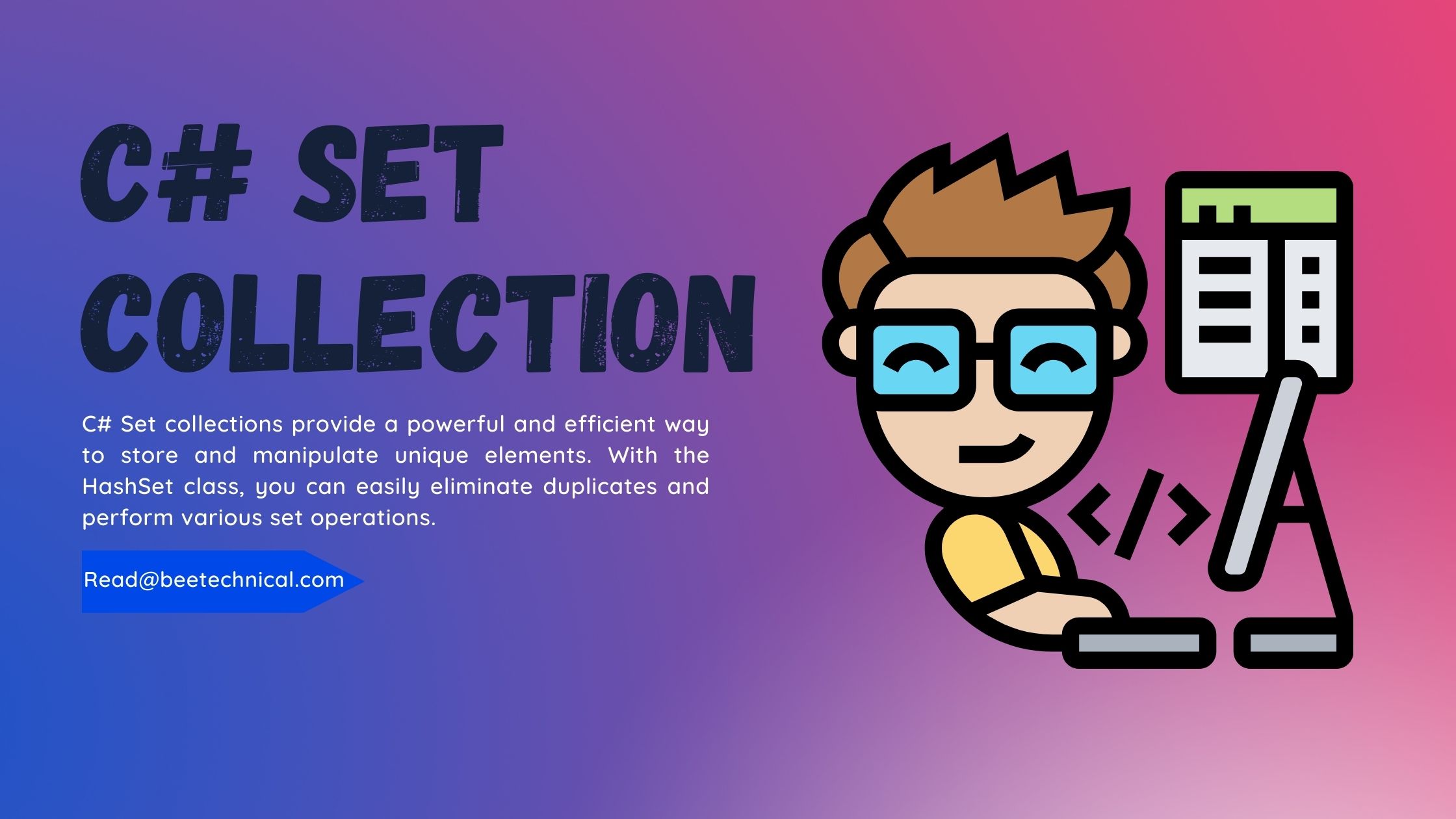
Whether you’re a beginner or an experienced C# developer, understanding set collections will expand your toolbox and enhance your programming skills. So, let’s get started!
Understanding Set Collections
C# Set collections provide a powerful and efficient way to store and manipulate unique elements. With the HashSet class, you can easily eliminate duplicates and perform various set operations.
HashSet<T>
The HashSet<T> class is the most commonly used set collection implementation in C#. It offers high-performance operations for adding, removing, and searching elements. Let’s explore some key features of the HashSet class using interactive examples.
Create HashSet
HashSet<string> names = new HashSet<string>(); names.Add("Deependra"); names.Add("Saurabh"); names.Add("Rahul"); names.Add("Deependra"); // Adding a duplicate element Console.WriteLine("Number of names: " + names.Count); // Output: 3
In this example, we create a HashSet of strings named names
and add four elements. Notice that the duplicate element “Deependra” is automatically eliminated, resulting in a set of three unique names. The Count property gives us the total number of elements in the set.
Checking Element Existence
HashSet<int> numbers = new HashSet<int>() { 1, 2, 3, 4, 5 }; bool containsThree = numbers.Contains(3); bool containsTen = numbers.Contains(10); Console.WriteLine("Contains 3? " + containsThree); // Output: True Console.WriteLine("Contains 10? " + containsTen); // Output: False
In this example, we create a HashSet of integers named numbers
and use the Contains method to check if specific elements exist in the set. It returns a boolean value indicating whether the element is present.
Set Operations
HashSet<int> setA = new HashSet<int>() { 1, 2, 3, 4, 5 }; HashSet<int> setB = new HashSet<int>() { 4, 5, 6, 7, 8 }; HashSet<int> union = new HashSet<int>(setA); union.UnionWith(setB); HashSet<int> intersection = new HashSet<int>(setA); intersection.IntersectWith(setB); HashSet<int> difference = new HashSet<int>(setA); difference.ExceptWith(setB); Console.WriteLine("Union: " + string.Join(", ", union)); // Output: 1, 2, 3, 4, 5, 6, 7, 8 Console.WriteLine("Intersection: " + string.Join(", ", intersection)); // Output: 4, 5 Console.WriteLine("Difference: " + string.Join(", ", difference)); // Output: 1, 2, 3
In this example, we demonstrate common set operations using two sets, setA
and setB
. The UnionWith method combines the elements from both sets, the IntersectWith method finds the common elements, and the ExceptWith method returns the elements that are present in setA
but not in setB
.
Advantages of using HashSet
HashSet offers several advantages, including
- Efficient element storage with automatic elimination of duplicates.
- Fast lookup and retrieval of elements.
- Support for set operations like union, intersection, and difference.
- Integration with LINQ for powerful querying capabilities.
- Flexibility to handle custom equality logic using custom equality compares.
How do I optimize the performance of a HashSet?
To optimize the performance of a HashSet, consider the following strategies:
- Set an appropriate initial capacity to avoid frequent resizing.
- Implement a custom equality comparer if you need specialized comparison logic.
- Minimize hash collisions by ensuring a well-distributed GetHashCode implementation for custom objects.
- Use methods like IntersectWith, ExceptWith, and UnionWith for efficient set operations.
- Understand the performance characteristics of HashSet methods and choose the appropriate ones for your needs.
Can I make a HashSet immutable in C#?
Yes, you can use the ImmutableHashSet<T> class from the System.Collections.Immutable namespace to create an immutable HashSet in C#. ImmutableHashSet provides thread-safe and immutable operations, allowing you to create and modify sets without changing the original instances.
using System; using System.Collections.Immutable; class Program { static void Main() { // Create an empty immutable HashSet of strings ImmutableHashSet<string> fruits = ImmutableHashSet<string>.Empty; // Add elements to the set (returns a new set) fruits = fruits.Add("Apple"); fruits = fruits.Add("Banana"); fruits = fruits.Add("Orange"); // Display the current elements in the set Console.WriteLine("Current fruits:"); foreach (string fruit in fruits) { Console.WriteLine(fruit); } // Try to add a duplicate element (returns the same set) ImmutableHashSet<string> updatedFruits = fruits.Add("Apple"); // Check if the set remained unchanged bool isSameSet = updatedFruits == fruits; // Result: true // Display the updated set (same as the original set)
Conclusion
Congratulations on mastering the usage of set collections in C#! You now have a solid understanding of the HashSet class and its powerful capabilities. Set collections provide an efficient way to work with unique elements, making them invaluable for various programming tasks.
Comments are closed.