Tuples in C# are a lightweight data structure that allows you to store multiple values of different types in a single object. They provide a convenient way to package related data together without the need to define a custom class or struct.
In C#, tuples are created using the tuple syntax, where you specify the types and values of the elements enclosed in parentheses.
When to Use Tupples?
Tuples can be used to return multiple values from a method, pass multiple values to a method, or even destructure them into individual variables.
They are particularly useful in scenarios where you need a temporary container for a small set of values that don’t warrant creating a separate type.
Tuples in C# provide a flexible and concise way to work with multiple values, improving code readability and reducing the need for additional data structures.
If you are not using the C# 7 version then the tuple can be included using the NuGet package System.ValueTuple
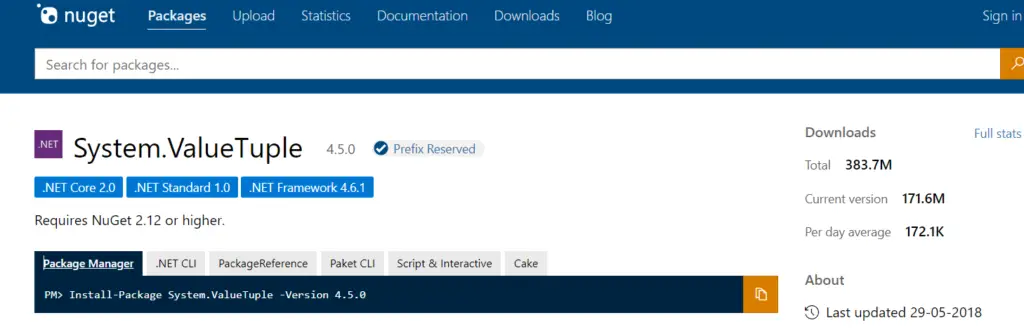
Tuple<> and Tuple both of the classes can be found inside the system namespace in C#.
var empInfo=new Tuple<string,string,int>("Deependra","Kushwaha",30);
Diffrent Ways to Create Tuples
In C#, there are different ways to create tuples, depending on your specific requirements and preferences.
Tuple Literal Syntax
The simplest way to create a tuple is by using the tuple literal syntax. You can enclose the values within parentheses and separate them with commas.
var tuple = (1, "Deependra", 27);
Tuple.Create Method
Another way to create a tuple is by using the static Create
method of the Tuple
class. This method allows you to explicitly specify the types and values of the tuple elements.
var tuple = Tuple.Create(1, "Deependra", 27);
Named Tuples
C# 7 introduced named tuples, which provide more clarity and expressiveness by assigning names to tuple elements. You can define named tuples by specifying the names along with the values.
var namedTuple = (Id: 1, Name: "Deependra", Age: 27);
Deconstruction
C# allows you to create tuples through deconstruction, where you assign values to individual variables from an existing data structure, such as an array or another tuple.
var array = new[] { 1, "Deependra", 27}; var (id, greeting, isActive) = array;
These are some of the common ways to create tuples in C#. Depending on your specific use case and the version of C# you are working with, you can choose the most suitable method to create and work with tuples effectively.
Example of Diffrent Usage
Tuples are a versatile data structure in C# that can be used in various scenarios to package and manipulate multiple values. Let’s understand some examples of it.
Returning Multiple Values from a Method
Tuples are often used to return multiple values from a method when you need to convey more than one piece of information.
public static (int, string) GetStudentDetails(int studentId) { // Retrieve student details from database int age = GetStudentAge(studentId); string name = GetStudentName(studentId); return (age, name); } // Usage: var studentDetails = GetStudentDetails(123); int age = studentDetails.Item1; string name = studentDetails.Item2;
Passing Multiple Values to a Method
Tuples can also be used to pass multiple values as parameters to a method. This is especially useful when the method requires related inputs that are closely associated.
public static void SavePersonInfo((string, int) personInfo) { string name = personInfo.Item1; int age = personInfo.Item2; // Save person information to the database } // Usage: var personInfo = ("John Doe", 30); SavePersonInfo(personInfo);
Deconstruction and Assignment
Tuples support deconstruction, which allows you to assign tuple elements to individual variables. This can be handy when you want to extract and work with specific values from a tuple.
var person = ("John Doe", 30); (string name, int age) = person; Console.WriteLine($"Name: {name}, Age: {age}");
These are just a few instances showcasing the usage of tuples in C#.
Difference between System.ValueTuple and System.Tuple?
In C#, both System.ValueTuple
and System.Tuple
are used to represent tuples, but there are some key differences between them
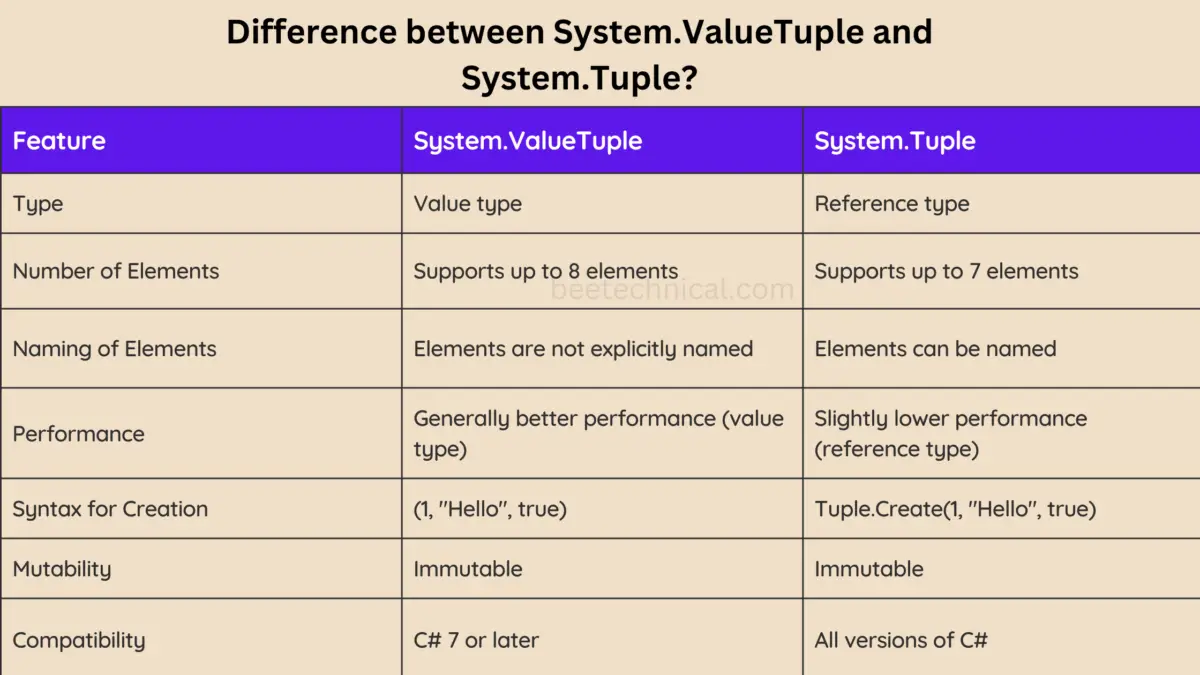
It’s important to note that while System.ValueTuple
is preferred for its performance and support for more elements, System.Tuple
can still be useful when explicit naming of tuple elements is desired. The choice between them depends on the specific requirements and preferences of your code.
Conclusion
Tuples in C# provide developers with a lightweight, concise, and efficient mechanism for packaging multiple values of different types into a single object.
They offer a simple and readable approach to handling temporary data containers without the need for defining custom classes or structs.