A Marker Interface in Java is an interface that does not contain any methods or fields, but instead, it is used as a marker or a tag to give special instructions to the compiler or JVM.
It simply marks or identifies the implementing class as having a particular characteristic or behavior. Examples of marker interfaces in Java include Serializable, Cloneable, and RandomAccess.
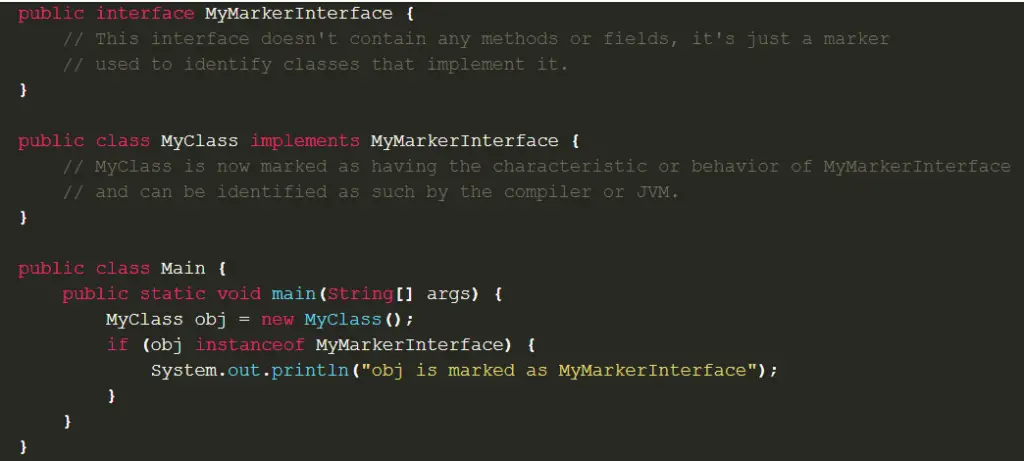
In this example, MyMarkerInterface
is a marker interface that doesn’t contain any methods or fields. MyClass
implements this interface, which means it’s marked as having the characteristic or behavior of MyMarkerInterface
. In the main
method, we create an object of MyClass
and check if it’s marked as MyMarkerInterface
using the instanceof
operator. If it is, we print a message to the console.
Built-in Marker interface in Java
Out of the box, Java provides 3 built-in marker interfaces for us:
java.lang.Cloneable
java.io.Serializable
java.rmi.Remote
You can review Javadoc for these interfaces to be sure that they perfectly suit the aforesaid definition of marker interface. As we already talk in the previous section about the Cloneable interface, let now give a floor to the remaining candidates.
java.io.Serializable
is used to make objects serializable. Serialization is writing Java objects as a sequence of bytes and reading these objects from the stream of bytes.
It is implemented ObjectOutputStream
for writing (or serialization) and ObjectInputStream
for reading (or deserialization). So in order to make a class serializable, we need to implement this marker interface.
Otherwise, we can receive NotSerializableException when for example calling ObjectOutputStream.writeObject(). By the way, all child classes (subclasses) of a serializable class are also serializable (we would talk about this in a moment).
java.rmi.Remote
is a bit more complicated and rare case and it is ok if you have not heard about it before. It is used to identify interfaces whose methods may be invoked from a non-local virtual machine.
Any remote object must directly or indirectly implement this interface. Only those methods specified in a remote interface (this is an interface that extends java.rmi.Remote) are available remotely. You can read more about remote interfaces in this good Oracle tutorial on remote interface design.
Also, there is another concept in Java that may seem similar – annotations. In the next section, we will overview the difference between annotations and marker interfaces.
How to create a marker interface in Java
it is possible to create marker interfaces in Java. A marker interface is an interface with no methods defined in it, and it is used to indicate to the Java compiler that a class implementing this interface should be treated specially.
Assume we want to indicate that a class is serializable, meaning that its objects can be converted into a stream of bytes and then deserialized back into an object. You can create a marker interface called Serializable
as follows:
public interface Serializable { }
Then, any class that implements this interface will be treated specially by the Java runtime environment and can be serialized and deserialized.
List of Marker Interfaces in Java
Here are some of the built-in marker interfaces in Java:
Serializable
: Indicates that a class is serializable, meaning that its objects can be converted into a stream of bytes and then deserialized back into an object.Cloneable
: Indicates that a class is cloneable, meaning that its objects can be copied by calling theclone()
method.RandomAccess
: Indicates that a List implementation supports fast, random access to its elements.SingleThreadModel
: Indicates that a servlet implements a thread-safe instance creation model. This interface has been deprecated in Java Servlet API version 2.4.Remote
: Indicates that a Java object is a remote object, meaning that it can be accessed from a different Java Virtual Machine.
Note that some of these interfaces have been deprecated or are not commonly used in modern Java programming, but they still exist in the Java language for backward compatibility and other purposes. Also, as I mentioned earlier, you can create your own marker interfaces to indicate special behaviors or properties of your classes.
Marker Interface vs Annotations
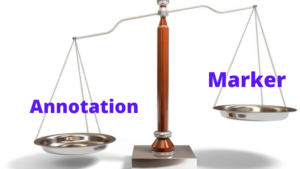
Java annotations may seem similar to marker interfaces as they allow us to do the same thing. We can apply an annotation to any class to indicate that it can be used in a specific logic. And there is a strong voice to abandon marker interfaces at and switch to annotations. But are they 100% similar? Consider the following code snippet:
class Document implements Serializable{ //..some fields }
So, we made our document serializable and now can write/read it with serialization as we talk before. But let’s consider if we make several concrete documents:
class Spreadsheet extends Document{} class Diagram extends Document{} //...
Hey! – you will ask – what if we don’t want to make diagrams serializable? But we can’t in this configuration. Marker interfaces follow polymorphism logic and therefore all children of a serializable class are also serializable. Another logic would be if we would use some @Serializable
annotation instead:
class Document{} @Serializable class Spreadsheet extends Document{} class Diagram extends Document{}
By the way Document
is also can be a marker interface, but we would talk about this in later posts.
Conclusion
In this short post, we discussed marker interfaces: what is it, what are built-in marker interfaces, and what is the difference between them and annotations. All Java developers to some degree encountered marker interfaces, but not everyone pays attention to that, in reality, it is a separate pattern. Hope this short article made you more interested in this concept. Have a nice day!
Comments are closed.