In the realm of Java programming, concurrency holds the key to maximizing the potential of modern multi-core processors.
Multithreading empowers applications to handle multiple tasks simultaneously, drastically improving performance. However, with great power comes great responsibility. Managing shared data across multiple threads can be perilous, leading to data corruption and synchronization issues.
Here’s where Java Concurrent List comes to the rescue! These powerful data structures, found within the java.util.concurrent
package, offer a safe and efficient solution for handling lists in multithreaded environments.
In this article, we will delve into the world of Java Concurrent List and explore how it enables us to harness the full potential of multithreading while keeping our data intact and our applications running smoothly. Let’s unlock the secrets of Java Concurrent List and unleash the true power of concurrency!
What are Concurrent Lists?
In Java, concurrent lists are thread-safe data structures designed to store and manage elements in a list-like fashion. They are part of the java.util.concurrent
package and serve as an excellent choice when you need to share a list between multiple threads without risking data corruption or other concurrency-related issues.
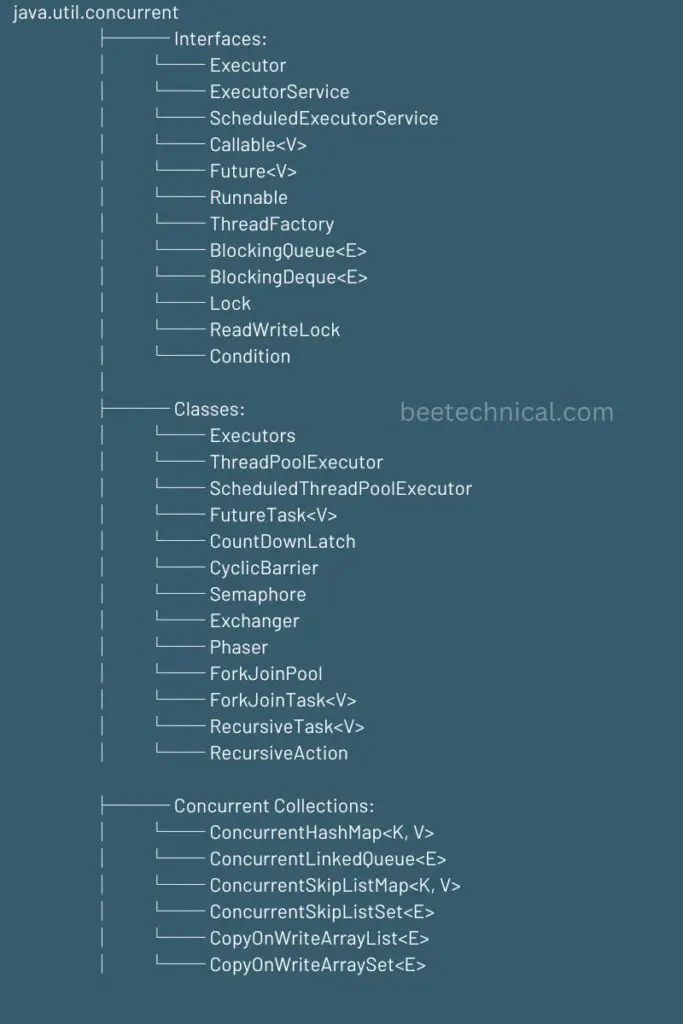
Java provides two main concurrent list implementations:
- CopyOnWriteArrayList
- ConcurrentLinkedDeque
CopyOnWriteArrayList With Example
This list is a thread-safe variant of the traditional ArrayList
. It ensures safety by creating a new copy of the underlying array every time an element is added, modified, or removed.
This approach allows for efficient reads but incurs a higher cost in terms of memory and performance during writes.
import java.util.concurrent.CopyOnWriteArrayList; public class ConcurrentListExample { public static void main(String[] args) { CopyOnWriteArrayList<String> concurrentList = new CopyOnWriteArrayList<>(); // Adding elements to the concurrent list concurrentList.add("Apple"); concurrentList.add("Banana"); concurrentList.add("Cherry"); // Concurrently read the elements using a separate thread Thread readerThread = new Thread(() -> { for (String fruit : concurrentList) { System.out.println(fruit); } }); readerThread.start(); // Concurrently modify the list using a separate thread Thread modifierThread = new Thread(() -> { concurrentList.add("Date"); concurrentList.remove("Apple"); }); modifierThread.start(); } }
In this example, we create a CopyOnWriteArrayList
named concurrentList
and add elements “Apple,” “Banana,” and “Cherry” to it.
We then launch two separate threads: one for reading the list and another for modifying it. Since CopyOnWriteArrayList
creates a new copy during modifications, the reading thread safely traverses the original list without any interference from the modifications.
ConcurrentLinkedDeque With Example
This list is an implementation of the Deque
interface and offers thread-safe operations for both ends of the list. It utilizes a lock-free algorithm based on concurrent updates to ensure safe multi-threaded access.
import java.util.concurrent.ConcurrentLinkedDeque; public class ConcurrentDequeExample { public static void main(String[] args) { ConcurrentLinkedDeque<Integer> concurrentDeque = new ConcurrentLinkedDeque<>(); // Adding elements to the concurrent deque concurrentDeque.add(10); concurrentDeque.add(20); concurrentDeque.add(30); // Concurrently remove elements from both ends using separate threads Thread frontRemoverThread = new Thread(() -> { System.out.println("Front Removed: " + concurrentDeque.pollFirst()); }); frontRemoverThread.start(); Thread rearRemoverThread = new Thread(() -> { System.out.println("Rear Removed: " + concurrentDeque.pollLast()); }); rearRemoverThread.start(); } }
In this example, we use a ConcurrentLinkedDeque
named concurrentDeque
to store integers. Two separate threads are launched to remove elements from the front and rear of the deque concurrently.
ConcurrentLinkedDeque
ensures safe concurrent access, allowing both threads to modify the deque simultaneously without any data corruption or conflicts.
Java concurrent List vs synchronized
Here’s a brief comparison between Java’s concurrent list implementations and using the synchronized
keyword for managing thread safety in multithreaded environments:
- Purpose:
- Concurrent List Implementations: Provide thread-safe data structures for efficient concurrent access and modification of lists.
- Synchronized Keyword: Ensures thread safety by allowing only one thread to execute a synchronized block or method at a time.
- Granularity:
- Concurrent List Implementations: Fine-grained control over concurrent access to different parts of the list.
- Synchronized Keyword: Coarse-grained control, as it locks the entire method or block.
- Performance:
- Concurrent List Implementations: Efficient for read-heavy scenarios, as they minimize contention between threads during reads.
- Synchronized Keyword: May lead to contention and reduced parallelism in high-concurrency scenarios.
- Write Operation:
- Concurrent List Implementations: Costly during writes due to copy-on-write strategy (for
CopyOnWriteArrayList
). - Synchronized Keyword: Can be faster for small critical sections.
- Concurrent List Implementations: Costly during writes due to copy-on-write strategy (for
- Concurrent Modification:
- Concurrent List Implementations: Efficient in scenarios with concurrent reads and few writes.
- Synchronized Keyword: Potential contention and reduced concurrency during modifications.
- Scalability:
- Concurrent List Implementations: Good scalability in read-heavy scenarios.
- Synchronized Keyword: Contention can limit scalability.
- Responsiveness:
- Concurrent List Implementations: Immediate visible changes for readers after each write.
- Synchronized Keyword: Changes become visible after releasing the lock.
- Ease of Use:
- Concurrent List Implementations: Easier to use and less error-prone in managing thread safety.
- Synchronized Keyword: Requires explicit synchronization, which can be more complex.
- Recommended Usage:
- Concurrent List Implementations: Suitable for read-heavy scenarios and cases with limited write operations.
- Synchronized Keyword: Recommended for specific scenarios where synchronization is required and contention is not a significant concern.
Conclusion
Concurrency is a powerful aspect of Java programming, enabling efficient utilization of multicore processors and enhancing application performance. Java concurrent lists, such as CopyOnWriteArrayList
and ConcurrentLinkedDeque
, provide thread-safe options for handling shared data structures in multithreaded environments.
By employing these concurrent lists, you can create robust and scalable applications that efficiently manage data across multiple threads, while avoiding the dreaded pitfalls of data corruption and inconsistent state.
Thank you for sharing your expertise in this area. I look forward to reading more of your insightful articles in the future!