In Java programming, the HashMap is a powerful data structure that allows you to store key-value pairs. Iterating through HashMap is a common operation when you want to access or process its elements. With the introduction of Java 8, the Stream API brought a more elegant and concise way to iterate and perform operations on collections. In this article, we’ll explore how to iterate through a HashMap using Streams with practical examples.
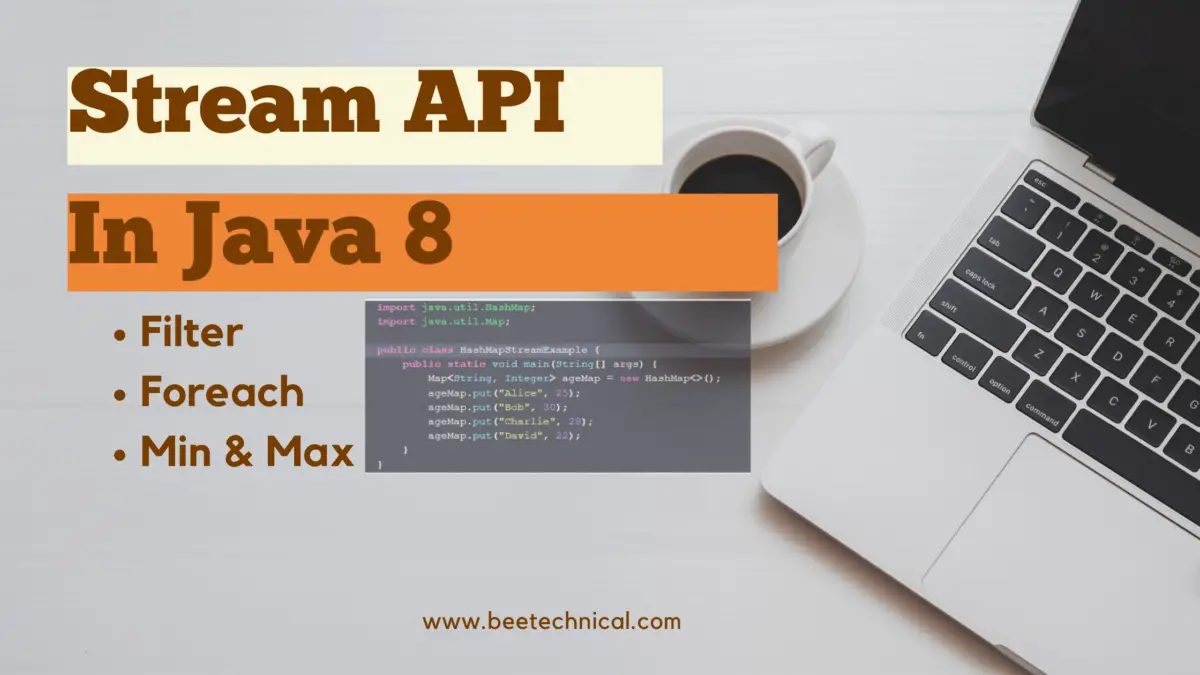
Stream API and HashMap
In Java, the Stream API introduced in Java 8 revolutionized the way we work with collections and data manipulation. The Stream API provides a more functional and declarative approach to processing data, allowing developers to perform operations on collections with ease and elegance.
The Stream API can be applied to HashMaps to perform operations on their elements, such as filtering, mapping, and reducing. Let’s explore how the Stream API can work with HashMaps using an example.
Iterating through HashMap
Consider a scenario where you have a HashMap containing names as keys and corresponding ages as values. We’ll demonstrate how to iterate through this HashMap using Streams.
Step 1: Creating the HashMap
import java.util.HashMap; import java.util.Map; public class HashMapStreamExample { public static void main(String[] args) { Map<String, Integer> ageMap = new HashMap<>(); ageMap.put("Alice", 25); ageMap.put("Bob", 30); ageMap.put("Charlie", 28); ageMap.put("David", 22); } }
Step 2: Using Streams to Iterate
Now, let’s use the Stream API to iterate through the HashMap and print the names along with their ages.
ageMap.entrySet().stream() .forEach(entry -> { String name = entry.getKey(); int age = entry.getValue(); System.out.println(name + " is " + age + " years old."); });
In this code snippet, we use the entrySet()
method to obtain a Set of key-value pairs (Map.Entry) from the HashMap. We then create a stream from this set and use the forEach
terminal operation to iterate through each entry. Inside the lambda expression, we extract the name and age from the entry and print the information.
Exploring Different Stream Methods
The Stream API in Java offers a range of methods that can be combined to perform various operations on collections like HashMaps. Let’s dive into some of these methods and see how they can be used with HashMaps.
Transforming and Collecting Data
Consider a scenario where you have a HashMap containing product names and their corresponding prices. You want to increase the price of each product by 10% and collect the modified entries into a new HashMap.
import java.util.HashMap; import java.util.Map; import java.util.stream.Collectors; public class StreamMethodsAndHashMap { public static void main(String[] args) { Map<String, Double> productPrices = new HashMap<>(); productPrices.put("Apple", 1.0); productPrices.put("Banana", 0.5); productPrices.put("Orange", 0.75); productPrices.put("Grapes", 2.5); // Using Stream API to transform and collect data Map<String, Double> updatedPrices = productPrices.entrySet().stream() .map(entry -> { String product = entry.getKey(); double originalPrice = entry.getValue(); double updatedPrice = originalPrice * 1.1; // Increase by 10% return Map.entry(product, updatedPrice); }) .collect(Collectors.toMap(Map.Entry::getKey, Map.Entry::getValue)); System.out.println("Updated prices: " + updatedPrices); } }
In the above code, we start with a HashMap named productPrices
, which contains product names as keys and their corresponding prices as values. Using the Stream API, we create a stream of the HashMap’s entries. We then use the map
operation to transform each entry by increasing the price by 10%. The map
operation returns a stream of the transformed entries, which are then collected into a new HashMap called updatedPrices
.
Filtet method in Stream API
Let’s use the same prodct price scenario and try to filter the data using stream API.
Map<String, Double> expensiveProducts = productPrices.entrySet().stream() .filter(entry -> entry.getValue() > 1.0) .collect(Collectors.toMap(Map.Entry::getKey, Map.Entry::getValue));
Min
and Max
Use these methods to find the minimum and maximum elements based on a comparator.
Map.Entry<String, Double> cheapestProduct = productPrices.entrySet().stream() .min(Map.Entry.comparingByValue()) .orElse(null);
Advantages of Using Streams
Using Streams for iterating through collections like HashMaps provides several benefits:
- Conciseness: Stream operations are concise and expressive, reducing the need for explicit loops and boilerplate code.
- Readability: Streams promote a more declarative style, making your code more readable and understandable.
- Parallelism: Streams can be easily parallelized, enabling efficient use of multi-core processors for better performance.
Conclusion
In this article, we’ve explored how to iterate through a HashMap using Streams in Java 8. We’ve seen that the Stream API provides an elegant way to process collections, making the code more concise and readable. By using streams, you can harness the power of functional-style programming to simplify your code and achieve better maintainability.
Remember, using Streams is just one way to iterate through a HashMap in Java. Depending on your specific use case, you might choose other methods, such as traditional for-each loops.