As Python is a modern programming language, It provides many data types for different applications per our needs.
Sometimes we need to convert a data type into another data type formally known as typecasting in some computer programming languages.
This article is based on the same scenario. In this article, we will learn how to “Convert list to String” by using various in-built methods in Python.
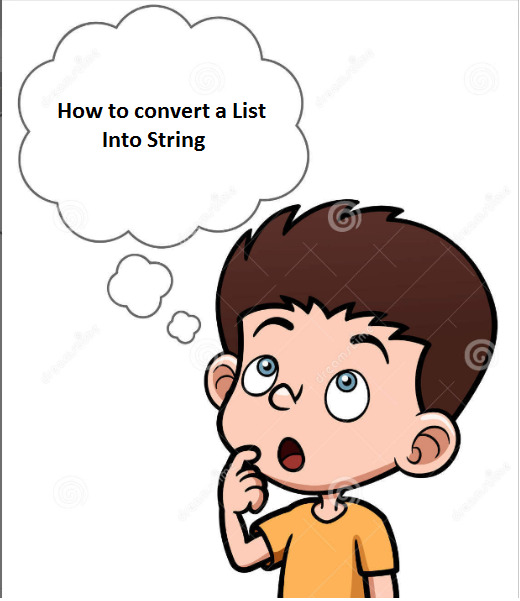
Before moving directly toward the conversion. Let us see the definition and difference between List and String in Python language.
What is String DataType in Python?
A String can be defined as the ordered sequence of characters from left to right. A string can contain greater than or equal to zero characters.
A string with zero characters is known as an Empty String. An empty string can be initialized via single or dual quotes and nothing in between the opening and closing quotes.
Below the code is provided as a demonstration of how to initialize an empty as well as a string containing a group of characters.
Code :
#initializing empty String empty_string = "" #initializing string containing characters string_containing_characters = "Beetechnical a tech blog site" #printing empty string print(empty_string) #printing string containing characters print(string_containing_characters)
Output:

What is List Datatype in Python?
A List can be defined as an ordered collection of elements of different data types.
It can easily contain characters, integers, float, etc as its elements.
There are many ways to initialize a List, The most common one is to put all elements in between square brackets, Whereas each element is in a single quote and separated by a comma (,) from its neighbor elements. Below the code is provided for the initialization of the List.
Code :
#initializing a List containing elements of different data try: List = ['A', 'B', 1, 3.5,] #printing List print("List : ", List)
Output :
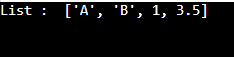
One can clearly see that list of variable names as List is containing characters A, and B integer 1, and a float value of 3.5. Therefore, It can be verified that a List can contain the elements of different data types.
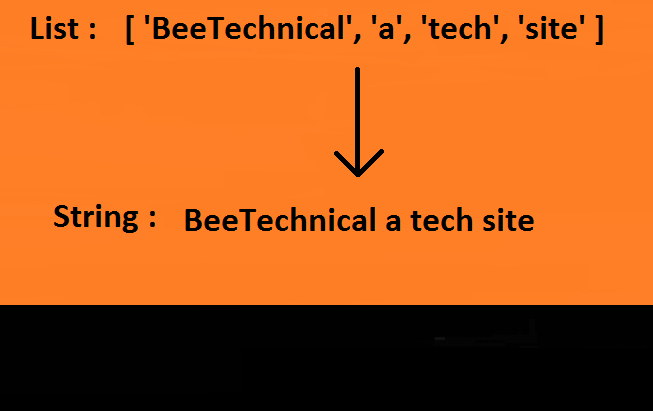
Convert List to String in Python
There is a total of 6 ways using in-built methods of python by which we can easily convert a List into a String in Python. Which 4 are most suitable as they can hold different data types while 2 carry some exceptional cases, Which are explained below as well with the most convenient methods.
There are mainly 4 ways we use to convert the list into a string in Python:
List Comprehension, map(), enumerate, reduce.
There are two more but this method fails when the list has both numbers as well as strings i.e.Iterate, join.
List Comprehension Method to Convert List to String
#Program to convert list into string # list comprehension List=["Learn", "python", 3, "with", "Beetechnical"] # conversion into String s=" ".join([str(x)for x in List]) print("Obtained String is : ", s)
Output :

In the code above, We can see that List contains a string along with an integer 3.
Therefore, We need to first convert elements into strings. That is why we are iterating over List from left to right using a loop and converting elements into the string using the str() function and then at last merging it into the final string that we will get as output after the end of the loop.
Convert List to Python using Map Function
Code :
#Program to convert list into string # initializing String List = ["Learn","python",3] # conversion into string using map function str=" ".join(map(str,List)) #printing string print("Obtained converted String : ",str)
Output :

In the code above, We are using the in-built map() function, While iterating over List and merging them into the String as variable str for the final desired output.
List to String using Enumerate
Code :
#Program to convert list into string using enumerate() # initalizing List List = ["Learn","python",3,"Beetechnical"] # conversion into String str=" ".join([str(x) for i, x in enumerate(List)]) #priting String print("Obtained converted String :", str)
Output :

In the code above, We declared a List containing elements of different data types and string str.
The above methodology is similar to List comprehension except for variables” i “and “enumerate()”, in this method, ‘i’ prevents from showing the index of list elements in the output.
Here index means Square bracket, otherwise, its output will remain as same as the output for the list we get.
Convert List to String Using Reduce Method
1. First import reduces function from functions.
2. Use two variables in which one traverses the list and another adds it to the string using the str function.
Code :
#Program to convert list into string using reduce #importing reduce from functools import reduce #initializing List List = ["Learn", "python", 3, "with", "BeeTechnical"] #Using reduce str = reduce(lambda i, j : i+ " " +str(j), List) #printing string print("Obtained string : ", str)
Output :

Convert List to String Using Iteration/Looping
Code :
#Program to convert list into string using simple iteration # Function for conversion def convertor(List): # initializing an empty string string = "" # traversing on the string using Loop for x in List: string += x # return string return string # driver code List = ["BeeTechnical", " a"," tech", " blog", " site"] #printing string print(convertor(List))
Output :

In the code above, We defined a function ourselves for the conversion of a List into a string by simply iteration List and taking each element of List and copying each element one by one into a string, and returning the string from the function.
It is not suitable as it gives errors if we take data type in a list other than character or string type. It can’t concatenate integers with strings.
List to String Using Join Method
# Python program to convert a list using join() #User defied function def convertor(List): # initializing an empty string string = " " # returning string return (string.join(List)) #Initializing List List = ["BeeTechnical"," python"," course"] #printing String print(convertor(List))
Output :

In the above-written code, we declared a user-defined function, Which takes List as an argument.
Inside the function scope, we are declaring an empty string of variable name string. This method is much simpler than the above method as in this no traversing or declaration of a random variable is required.
It only uses join() to join the elements of a given list into a string and return the string to the driver function.
It also has the same exception as above can’t accept integers in a list because it can’t concatenate strings and integers.
Conclusion:
In the article, we came to know what they are String and List, the difference between them, and how we can convert a list into a string in python by using various in-built functions, Limitations, and uses of the above methods are discussed as well.