If you take any application, big or small, generating a random number is one of the basic operations required. If you are a big fan of writing unit test cases, you must have implemented this for mock data generation.
We can utilize the random module in python to generate a random number in python. List of methods offered by the random module to generate the number.
- random()
- randint()
- sample()
Generate a Random Number using the random function in python
The random() function takes no parameters and returns values uniformly distributed between 0 and 1.
import random randomNumber=random.random() print(randomNumber)
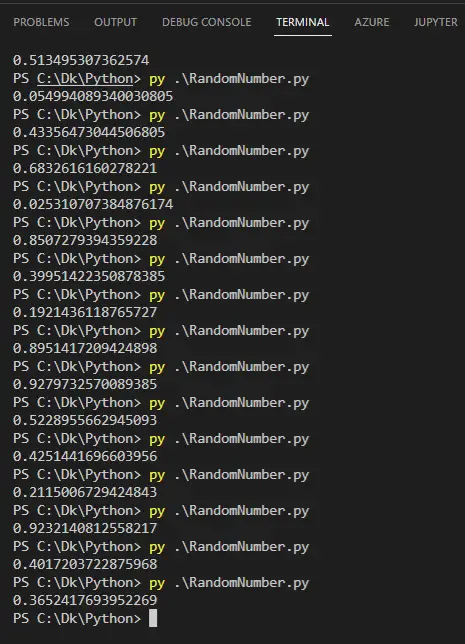
Random Function With Range in Python
We can use random.rand function to generate the random number between the lower and higher value provided in the function.
random.rand(0,100) | It will generate random numbers between 0 and 100, where the generated number will be greater than 0 and less than 100. | |
random.rand(1000,9999) | It will generate random numbers between 1000 and 999, where the generated number will be greater than 1000 and less than 999. | |
random.rand(1,6) | It will generate a random number between 1 and 6. where the generated number will be greater than 1 and less than 6. | |
import random randomNumber=random.randint(10,55) print(randomNumber)
As you can see in the above program, numbers will be generated between only 10 and 44.
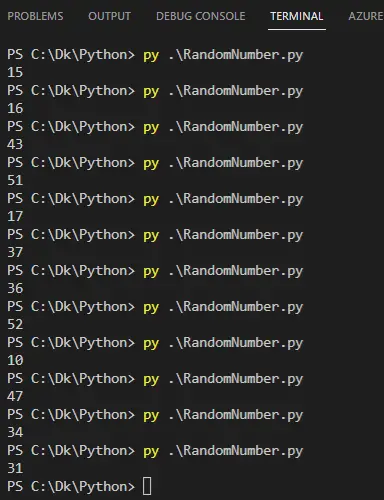
Random Sample Function in Python
To instantly generate a list of random numbers, we can also use the sample() method offered by the random module. Here, we specify a range and the number of random numbers that should be generated.
import random randomlist = random.sample(range(10, 30), 5) print(randomlist)
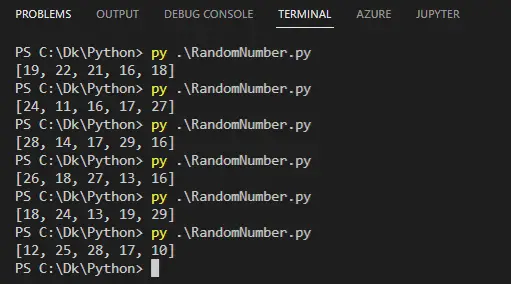
Conclusion
The random module offers a variety of functions that allow for the generation of random numbers. Among these functions are random, randint, and sample. We have examined the usage of these methods.
Comments are closed.