In Java, reading CSV files is a common task for developers dealing with data. CSV files are a popular format for storing and exchanging data, but they can be challenging to read programmatically.
Fortunately, Java provides several libraries and classes to make this task easier. One of the most popular libraries for reading CSV files in Java is OpenCSV, which provides a simple and easy-to-use API for parsing CSV files. Additionally, Java’s built-in FileReader and BufferedReader classes can also be used to read CSV files.
By leveraging these libraries and classes, developers can quickly and easily read CSV files in their Java applications, allowing them to process and analyze data more efficiently.
What is CSV File?
CSV stands for Comma-Separated Values. It is just like another file, Which stores tabular data in it. The CSV File has a .csv extension.
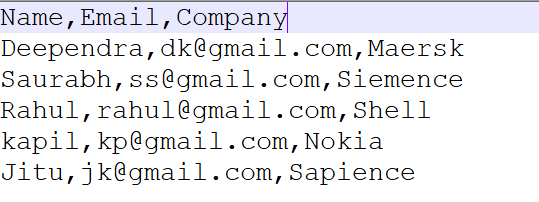
A comma separates the values inside the file (,). A comma is a default separate among the values present in the CSV file.
This type of file can be easily imported and exported from applications, Which store the data in tabular formats such as Microsoft Office and Excel.
How to create a CSV File?
Now we will see how to create a CSV File. There are two methods by which we can create a CSV File. The two methods are listed below:
- By Notepad
- By Microsoft Excel
We will see both of the methods for creating CSV files one by one. Let us see first the method to create CSV File.
How to create a CSV File using MS Excel
1. First of all create a new sheet and create a database formally tabular data. An example of tabular data is below as an image.
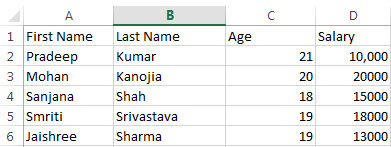
2. Save the file in CSV Format by giving it an appropriate name of your choice. In the picture below we are saving tabular data in CSV Format.
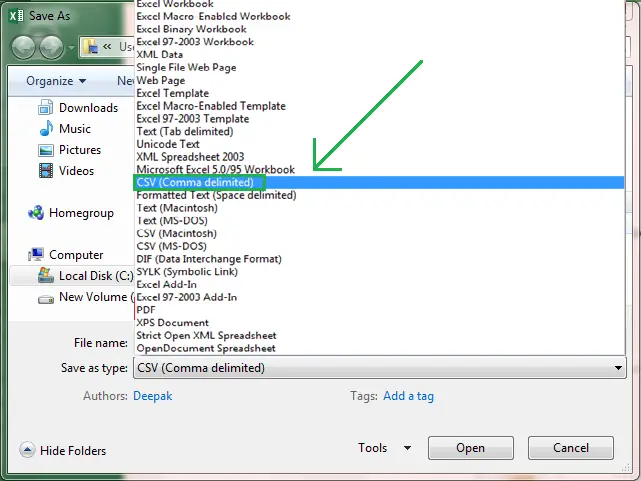
How to create a CSV File using Notepad:
CSV files can also be created using Notepad, Let us try to make some tabular data in Notepad, Which we can open in an Excel sheet.
The values in Notepad should be separated by a delimiter, Formally a comma(,). We insert the tabular data below in the image in Notepad File and then save it as a file in .csv format.
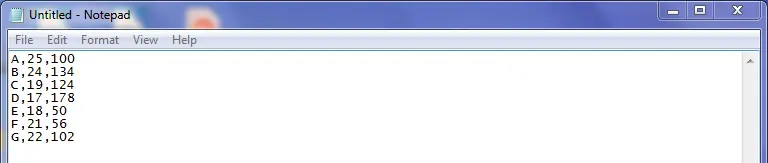
After saving it as a file in .csv format. The file can be opened via Microsoft Excel. In the image below our file created in notepad having .csv format has opened in Microsoft Excel.
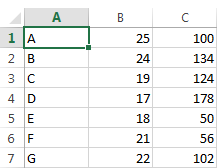
So far till now, We have seen What is CSV file, How it stores that data, and how to create it.
Now it’s time to discuss some methods by which we can read CSV Files in Java. So basically we will discuss three methods, Which are listed below.
- By Using Scanner
- By String class
- By OpenCSV library of Java
Let us discuss all three methods one by one.
1. Scanner Class to Read CSV File In Java
The scanner class contains a lot of in-built methods by which we can read a CSV File. First, We have to create an object of the Scanner class by putting a File type object containing the file’s location inside the Scanner constructor.
Then it breaks the data into the form of tokens and uses a delimiter as a separator between values. Then next() method provides the values of tabular Data.
Below the code is provided as a demonstration to read CSV Files using the Scanner class.
package newPackage; import java.io.*; import java.util.Scanner; public class BeeTechnical { public static void main(String[] args) throws Exception { //Creating a File type object containing Location of CSV File File file = new File("D:\\CSV_File.csv"); //Scanner object containing file object in its Constructor Scanner sc = new Scanner(file); //Comma is assigned as a delimeter sc.useDelimiter(","); //sc.hasNext() returns boolean value true/false //Until there are some unread values in the CSV File, while (sc.hasNext()) { //find and returns the complete token as values System.out.print(sc.next()); } //closing the scanner object sc.close(); } }
Output:
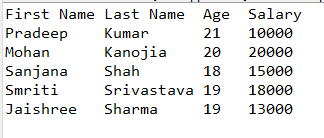
- In the code above, First, we are making a File type object “file” by giving the location of the CSV File in the File’s Constructor.
- Then we are putting this “file” object inside the Scanner class’s Constructor.
- After that, we are running a While loop until there are some unread values present in the File. sc.hasNext() method will return false when there are no values left to read, this will terminate the condition of the while loop.
2. Read CSV File using String Class
We can use the .split() function of the string class, This function identifies the delimiters in File and then splits the rows into tokens.
This String.split() method returns an array of a string of present values inside the file. In this method, we use BufferedReader class until the EOF(End of File) character is reached.
package newPackage; import java.io.BufferedReader; import java.io.FileReader; import java.io.IOException; public class BeeTechnical { public static void main(String[] args) { //String "line" is initialized, Which will print a row of file String line = ""; //String "splitBy" is initialized with Comma String splitBy = ","; //Try Block try { //parsing a CSV file's location into BufferedReader class constructor BufferedReader br = new BufferedReader(new FileReader("D:\\CSV_File.csv")); //br.readLine() returns boolean value true/false until there are //no lines left to read while ((line = br.readLine()) != null) { // use comma as separator String[] person = line.split(splitBy); //Printing Line of CSV File System.out.println("Person [First Name = " + person[0] + ", Last Name = " + person[1] + ", Age = " + person[2] + ", Salary = " + person[3] +"]"); } } //Catch Block catch (IOException e) { e.printStackTrace(); } } }
Output:

- In the code above, First, we are making an object “br” of BufferedReader class and putting a FileReader type object containing CSV File’s location, inside BufferedReader class’s Constructor.
- Then, run a while loop till there are lines left to read inside CSV File.
- A loop will terminate when there will no line to read further.
3. Read CSV File using OpenCSV API
It is a Third party API, Which contains numerous methods to read CSV Files. This library has some best methods to handle CSV Files as well.
package newPackage; import java.io.FileReader; import com.opencsv.CSVReader; public class BeeTechnical { public static void main(String[] args) { //Creating object of CSVReader Type CSVReader reader = null; //Try Block try { //parsing CSV file's location into CSVReader class constructor reader = new CSVReader(new FileReader("D:\\CSV_File.csv")); //String array nextLine is declared String [] nextLine; //reads one line at a time, read.hasNext() will not return null //until there are no lines left to read while ((nextLine = reader.readNext()) != null) { for(String token : nextLine) { System.out.print(token); } System.out.print("\n"); } } //Catch Block catch (Exception e) { e.printStackTrace(); } } }
Output:
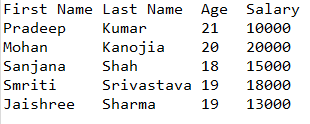
The output is the same as reading CSV Files using the Scanner class.
How to read CSV files in java using an input stream?
We will be using the getResourceAsStream method to obtain an InputStream object pointing to the “D:\CSV_File.csv” file located in the same package as our Java class. Then, we’re wrapping the InputStream object with an BufferedReader
object to read the contents of the file. Finally, we’re using the readLine
method to read each line of the CSV file and the split
method to separate the values on each line.
import java.io.BufferedReader; import java.io.InputStream; import java.io.InputStreamReader; import java.nio.charset.StandardCharsets; public class ReadCSVUsingInputStreamExample { public static void main(String[] args) { try { // Create an InputStream object pointing to your CSV file InputStream inputStream = ReadCSVUsingInputStreamExample.class.getResourceAsStream("D:\\CSV_File.csv"); // Wrap the InputStream object with a BufferedReader to read the contents BufferedReader reader = new BufferedReader(new InputStreamReader(inputStream, StandardCharsets.UTF_8)); // Read the CSV file line by line String line; while ((line = reader.readLine()) != null) { // Split the CSV line by commas String[] values = line.split(","); // Do something with the values for (String value : values) { System.out.print(value + " "); } System.out.println(); } // Close the reader and the input stream reader.close(); inputStream.close(); } catch (Exception ex) { ex.printStackTrace(); } } }
How to Read CSV files using multithreading in java?
In this example, we’re using a BufferedReader
to read the CSV file line by line. For each line of the CSV file, we’re submitting a new task ExecutorService to process the line. The ExecutorService is created with a fixed number of threads, which allows us to process multiple lines of the CSV file concurrently. We’re using a CSVLineProcessor class to process each line of the CSV file as a separate task. Finally, we’re shutting down ExecutorService
when all tasks have been completed and close the BufferedReader
.
import java.io.BufferedReader; import java.io.FileReader; import java.util.concurrent.ExecutorService; import java.util.concurrent.Executors; public class ReadCSVUsingMultithreadingExample { public static void main(String[] args) { try { // Create a BufferedReader to read the CSV file BufferedReader reader = new BufferedReader(new FileReader("example.csv")); // Create an ExecutorService with a fixed number of threads ExecutorService executorService = Executors.newFixedThreadPool(5); // Read the CSV file line by line String line; while ((line = reader.readLine()) != null) { // Submit a new task to the ExecutorService to process each line executorService.submit(new CSVLineProcessor(line)); } // Shutdown the ExecutorService when all tasks have completed executorService.shutdown(); // Close the reader reader.close(); } catch (Exception ex) { ex.printStackTrace(); } } private static class CSVLineProcessor implements Runnable { private final String line; public CSVLineProcessor(String line) { this.line = line; } @Override public void run() { // Process the CSV line String[] values = line.split(","); // Do something with the values for (String value : values) { System.out.print(value + " "); } System.out.println(); } } }
The multithreading approach to reading CSV files in Java is useful in situations where you need to process a large amount of data contained in the CSV file. By using multiple threads to read and process the data, you can significantly improve the performance of your application and reduce the time it takes to complete the processing.
For example, consider a scenario where you have a CSV file containing thousands of rows of data that need to be processed and analyzed. Reading and processing this data sequentially could take a long time and could cause delays in your application. By using multithreading, you can split the data processing into multiple threads that can run concurrently and complete the task much faster.
Additionally, the multithreading approach allows you to control the number of threads used to process the data. This can be useful when dealing with limited system resources, as you can adjust the number of threads to optimize performance without overloading the system.
Overall, using multithreading to read and process CSV files in Java is a powerful technique that can help improve the performance and scalability of your applications when dealing with large amounts of data.
How to java read CSV files into ArrayList?
In this example, we’re creating an ArrayList called csvData to hold the data read from the CSV file. We’re using a BufferedReader to read the CSV file line by line and then split each line into an array of String
values using the split
method. We’re then adding each array of String
values to the csvData
ArrayList using the add
method.
After reading the CSV file into the csvData
ArrayList, we can process the data in any way we want. In this example, we’re simply printing out the data to the console, but you could perform more complex operations on the data, such as filtering or sorting the data before processing it.
import java.io.BufferedReader; import java.io.FileReader; import java.util.ArrayList; import java.util.List; public class ReadCSVIntoArrayListExample { public static void main(String[] args) { List<String[]> csvData = new ArrayList<>(); try { // Create a BufferedReader to read the CSV file BufferedReader reader = new BufferedReader(new FileReader("example.csv")); // Read the CSV file line by line String line; while ((line = reader.readLine()) != null) { // Split the CSV line by commas String[] values = line.split(","); // Add the values to the ArrayList csvData.add(values); } // Close the reader reader.close(); } catch (Exception ex) { ex.printStackTrace(); } // Do something with the CSV data in the ArrayList for (String[] values : csvData) { for (String value : values) { System.out.print(value + " "); } System.out.println(); } } }
The use case for reading a CSV file into an ArrayList
in Java when you need to store the data from the CSV file in a dynamic data structure that allows for easy processing and manipulation.
The ArrayList
is a data structure that provides the ability to add and remove elements dynamically, making it an ideal choice for storing data that may change in size. By reading the CSV file into an, you can easily access and manipulate the data using the methods provided by the ArrayList
class.
For example, consider a scenario where you have a CSV file containing customer data that needs to be processed and analyzed. By reading the CSV file into an, you can easily manipulate the data to extract specific information, such as customer names or addresses. Additionally, you can easily perform operations on the data, such as sorting or filtering, to generate reports or other analyses.
Another use case for reading a CSV file into an ArrayList
is when you need to pass the data to another part of your application for further processing. For example, you may need to read the CSV file into an ArrayList
and then pass the data to a database or web service for processing. By using an, you can easily transfer the data between different parts of your application without having to worry about the underlying data structure or format.
Overall, reading a CSV file into an ArrayList
is a common use case in Java when dealing with data that needs to be processed and manipulated dynamically. The ArrayList
data structure provides a flexible and powerful way to store and manipulate data, making it an ideal choice for many applications.
Conclusion:
In conclusion, Java offers several options for developers to read CSV files, including third-party libraries like OpenCSV and built-in classes like FileReader and BufferedReader. These tools make it easier to parse and process CSV data in Java applications, enabling developers to work more efficiently with this popular data format. By utilizing these tools, developers can quickly and accurately read CSV files and unlock the valuable insights contained within their data.
Comments are closed.