In the world of development, one common problem statement and frequently asked interview question is how to reverse a string in C#. In this article, we will explore several effective methods for achieving this task.
Using For Loop
Using a for
loop: One way to reverse a string is to loop through each character of the string and add it to a new string in reverse order.
string input = "hello"; string reversed = ""; for (int i = input.Length - 1; i >= 0; i--) { reversed += input[i]; } Console.WriteLine(reversed); // Output: "olleh"
Using While Loop
In this example, we start from the last index of the input string and iterate backward using a while loop. Inside the loop, we append each character to the reversedString
variable. The loop continues until we reach the first index of the input string (i.e., i >= 0
). Finally, we return the reversed string.
public static string ReverseString(string inputString) { string reversedString = ""; int i = inputString.Length - 1; while (i >= 0) { reversedString += inputString[i]; i--; } return reversedString; } // Example usage string inputStr = "Hello, World!"; string reversedStr = ReverseString(inputStr); Console.WriteLine(reversedStr); // Output: "!dlroW ,olleH"
Using a while loop allows us to achieve the same result as the previous example using a for loop, but with a slightly different looping construct.
Inbuilt Methods Array.Reverse
One of the simplest and most straightforward methods is to use the Array.Reverse
the method in conjunction with the ToCharArray
method of the string.
Here is an example:
using System; namespace ReverseString { class Program { static void Main(string[] args) { Console.WriteLine(ReverseString("Hello World!")); } static string ReverseString(string input) { char[] charArray = input.ToCharArray(); Array.Reverse(charArray); return new string(charArray); } } }
This program takes a string as input and returns the reversed string. The ToCharArray
method converts the string into an array of characters and Array.Reverse
reverses the elements in the array. Finally, the reversed character array is converted back into a string using the new string
constructor.
Using StringBuilder
The fastest way to reverse a string in C# is by using the StringBuilder
class. This class provides a mutable string object, which means that it can be modified without creating a new object every time.
string input = "hello"; StringBuilder reversedBuilder = new StringBuilder(); for (int i = input.Length - 1; i >= 0; i--) { reversedBuilder.Append(input[i]); } string reversed = reversedBuilder.ToString(); Console.WriteLine(reversed); // Output: "olleh"
Using Linq
Using LINQ: This method uses the Reverse()
extension method from the System.Linq
namespace to reverse the characters in the string. Here’s an example:
string input = "hello"; string reversed = new string(input.Reverse().ToArray()); Console.WriteLine(reversed); // Output: "olleh"
All of these methods produce the same output, which is the reversed string. The choice of which method to use will depend on factors such as performance, readability, and personal preference.
Using Recursion
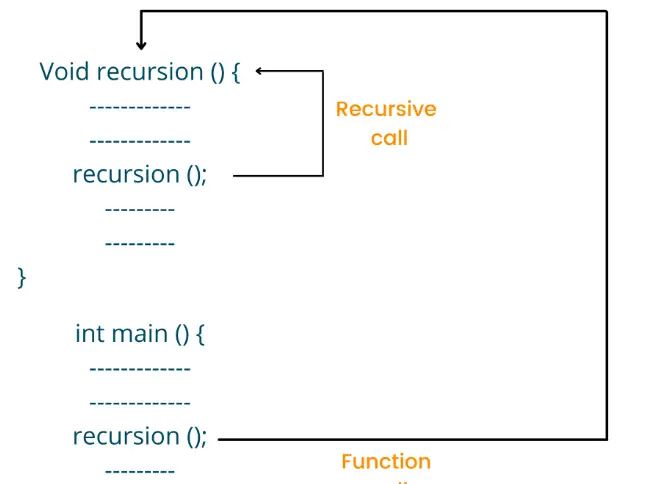
In C#, a string can be reversed using recursion. Recursion is a programming technique where a function calls itself repeatedly until a specific condition is met. Here’s an example of how to reverse a string using recursion:
using System; public class Program { public static void Main() { string input = "hello"; string reversed = ReverseString(input); Console.WriteLine(reversed); } public static string ReverseString(string str) { if (str.Length == 0) { return str; } else { return ReverseString(str.Substring(1)) + str[0]; } } }
In this example, we start by defining the ReverseString
method, which takes a string as input and recursively reverses it. The method first checks if the length of the string is 0.
If it is, then the method simply returns the input string. If the length is not 0, then the method calls itself with a substring of the input string that excludes the first character and concatenates the first character to the end of the reversed substring.
Note that recursion can be a powerful technique for solving certain problems, but it can also lead to stack overflow errors if not used carefully. It’s important to understand the limitations and trade-offs of recursion and choose an appropriate approach for each specific problem.
The fastest way to reverse string
In C#, the fastest way to reverse a string is by converting it to a character array, then using a for loop to swap the characters in place. This approach avoids the overhead of string concatenation or building a new string object.
public static string ReverseString(string inputString) { char[] charArray = inputString.ToCharArray(); int i = 0; int j = charArray.Length - 1; while (i < j) { char temp = charArray[i]; charArray[i] = charArray[j]; charArray[j] = temp; i++; j--; } return new string(charArray); } // Example usage string inputStr = "Hello, World!"; string reversedStr = ReverseString(inputStr); Console.WriteLine(reversedStr); // Output: "!dlroW ,olleH"
By manipulating the character array directly, we avoid unnecessary string allocations and improve performance. This approach has a time complexity of O(n/2), where n is the length of the input string, as we only need to iterate through half of the characters to reverse the string.
Conclusion
In conclusion, understanding the various approaches to reversing a string in C# and their trade-offs can help you write more efficient and performant code.
It’s important to choose the approach that best fits your specific use case and to always keep performance in mind when dealing with large or frequently updated strings.
Comments are closed.