After reading this article. You will be familiar with the fundamentals of regular expression & how to use regex in python.
A regex in or Regular Expression in Python is a sequence of characters that forms a search pattern.RegEx can be used to check if a string contains the specified search pattern.
- Regular Expression are symbols in python that make your job in extracting much easy
- Before you can use regular expressions in your program, you must import the library using “ import re “
- There are some functions that you can use by this library that makes extracting data easier to code
- re.search()
- To see if a string matches a regular expression, similar to the find() method for strings
- re.findall()
- To see if a string matches a regular expression, similar to “find()+slicing “
Regular Expressions in python:
- ( ^ ) Matches the beginning of a line
- ( . ) Matches any character
- ( $ ) Matches The End of a line
- ( \s ) Matches any whitespace character
- ( \S ) Matches any non-whitespace character
- ( * ) Repeats a character zero or more time
- ( *? ) Repeats a character zero or more times (Nongreedy)
- ( + ) Repeats a character one or more time
- ( +? ) Repeats a character one or more times (Nongreedy)
- [aeiou] Matches a single character in the listed set
- [^XYZ] Matches a single character, not in the listed set
- [a-z0-9] the set of characters can include a range
- ( indicates where string extracting is to start
- ) indicates where string extracting is to End
- [ ] nonblank character
How to Use Regex in Python for Email Validation
In this example, we will give a simple code to find any emails in a text file
Step 1:
- When you use a regular expression you should import its library first which called ‘re’ that library gives you all the regular expressions that you need to use in the program.
- You can import this library by writing at the beginning of the code (import re)

Step 2:
- Make an input function for the user to let him enter the name of the text file
- Also, add to the name the text file extension (.txt)
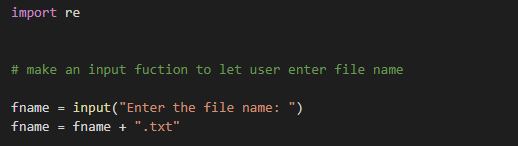
Step 3:
- You should use open function now to open the file and read it, using open() and an attribute ‘r’
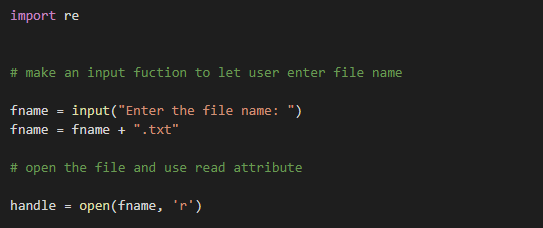
Step 4:
- Now we should start dealing with each line separately, so you should make a for loop
- inside that loop, you should add a statement block to find each line that has an email
- if you were about to use anything else as the previous tutorials, it would take many lines from you to find an email inside that line but using regular expression it only needs two lines
- you must use re.findall() and a regular expression ( ‘\S+@\S+’), that regular expression means you have to find any word that has ‘@’ and before and after the ‘@’ any word with no white spaces
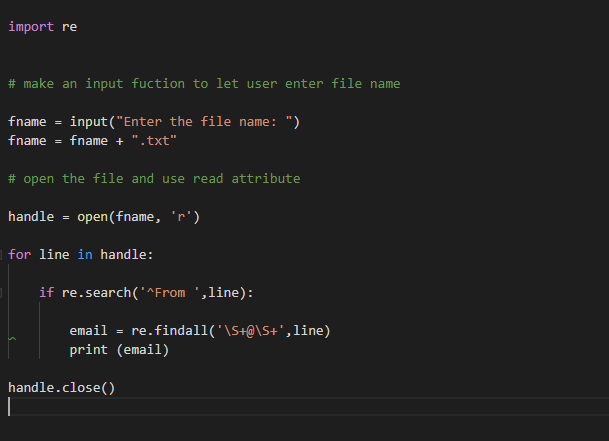
Step 5:
- Run the code to see how the regular expression code find the emails through the file
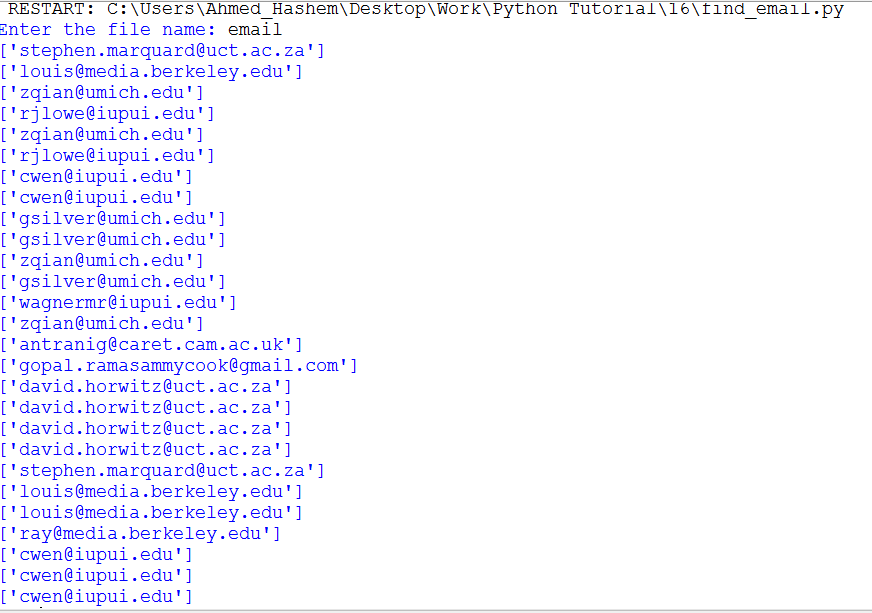
In this tutorial, We tried to understand the basics of regular expressions or regex in Python with example. Let us know about your thoughts or any concern in the below comments section.